Pyspark Interview Questions
1. What is PySpark Architecture?
PySpark architecture is the underlying framework of PySpark that defines how data processing is carried out on a distributed computing platform. PySpark is built on top of Apache Spark, which is a distributed computing engine for large-scale data processing. PySpark provides a Python interface for Spark, allowing Python developers to easily use Spark’s capabilities to process large amounts of data.
PySpark architecture is divided into several layers, each with its own set of components:
- Application Layer: This layer is where PySpark applications are developed using PySpark API. The PySpark API allows developers to interact with Spark and perform various data processing operations.
- Spark Core Layer: This layer is the core of the Spark architecture and includes the distributed execution engine, cluster manager, and task scheduling capabilities. It provides the basic functionality for distributed computing, such as scheduling, memory management, and fault tolerance.
- Distributed Storage Layer: This layer is responsible for storing data across the cluster in a distributed manner. The primary storage component is the Resilient Distributed Dataset (RDD), which is a fault-tolerant, immutable data structure that allows data to be processed in parallel across the cluster.
- Distributed Processing Layer: This layer provides the ability to process data in parallel across the cluster using a variety of APIs, including Spark SQL, Spark Streaming, and Spark Machine Learning.
- Cluster Manager Layer: This layer is responsible for managing the cluster resources, including memory, CPU, and disk, and for scheduling tasks on the cluster. Spark supports several cluster managers, including YARN, Mesos, and Spark’s own standalone cluster manager.
PySpark architecture provides a scalable and fault-tolerant framework for processing large amounts of data across a distributed computing environment. It is widely used in various industries, including finance, healthcare, and retail, for processing big data and extracting insights from it.
2. What PySpark DAGScheduler?
The PySpark DAGScheduler is a key component of the PySpark execution engine that schedules Spark jobs on a cluster. DAG stands for Directed Acyclic Graph, which is a data structure used to represent the computation flow of a Spark job.
The PySpark DAGScheduler takes the Spark job represented as a series of transformations and actions and constructs a DAG of stages, which are sets of tasks that can be executed in parallel. The DAGScheduler optimizes the job execution by analyzing the dependencies between the stages and minimizing data shuffling between the nodes in the cluster.
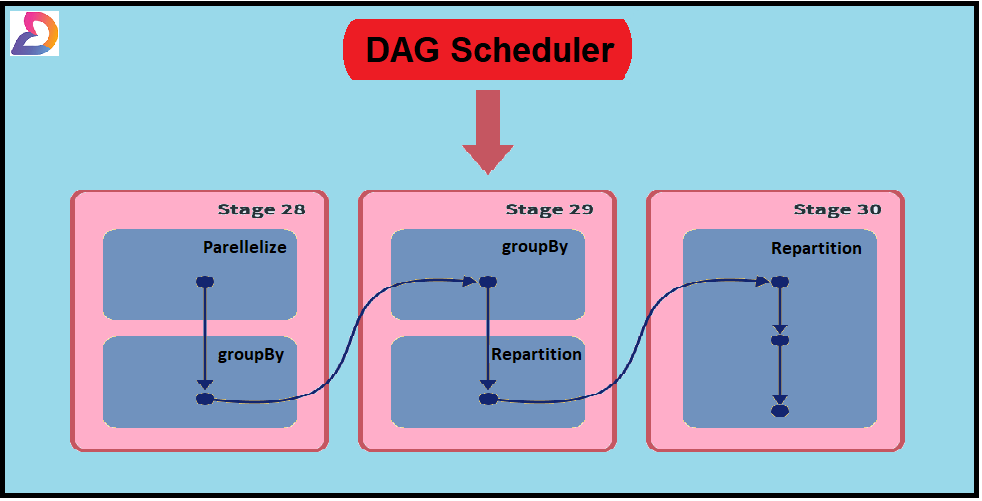
Here’s how the PySpark DAGScheduler works:
- Job creation: When a Spark application is submitted, PySpark creates a DAG of transformations and actions that need to be executed.
- Stage creation: PySpark DAGScheduler divides the job into stages based on the dependencies between the transformations. Each stage consists of a set of tasks that can be executed in parallel.
- Task scheduling: PySpark DAGScheduler schedules the tasks in each stage to run on the cluster nodes. It takes into account the available resources on each node and the dependencies between the tasks.
- Result aggregation: PySpark DAGScheduler aggregates the results of each task and returns the final output to the user.
The PySpark DAGScheduler plays a crucial role in optimizing the execution of Spark jobs by reducing data shuffling and minimizing the overhead of scheduling tasks on the cluster. It is one of the key components of the PySpark execution engine that makes it a powerful tool for processing large-scale data.
3. What is the common workflow of a spark program?
The common workflow of a Spark program involves the following steps:
- Creating a SparkContext: The SparkContext is the entry point for Spark and it is used to configure the Spark application. It allows the application to communicate with the Spark Cluster Manager and access the distributed data.
Loading data: In the second step, the data is loaded into Spark from a variety of sources such as HDFS, Amazon S3, or a local file system.
Transforming data: The data is transformed using a series of transformations such as filtering, grouping, aggregating, joining, and sorting. These transformations are performed on RDDs (Resilient Distributed Datasets) or DataFrames.
Caching data: If there is a need to reuse a RDD/DataFrame multiple times, the data can be cached in memory to avoid the cost of recomputing it each time.
- Performing actions: Actions are operations that return a value to the driver program or write data to an external storage system. Examples of actions include count, collect, save, and foreach.
- Monitoring and tuning: Spark applications can be monitored and tuned to improve performance. This involves analyzing the performance metrics such as the time taken for each stage, the amount of data shuffling, and the memory usage.
- Stopping the SparkContext: Once the Spark application has completed its tasks, the SparkContext is stopped to release the cluster resources.
Overall, the common workflow of a Spark program involves loading data, transforming it, caching it if necessary, performing actions, monitoring and tuning performance, and stopping the SparkContext. Spark provides a high-level API that makes it easy to perform distributed computing tasks and process large-scale data.
4. Why is PySpark SparkConf used?
PySpark’s SparkConf
is used to configure Spark settings, such as the Spark master URL, application name, and other settings related to the execution environment.
Here are some reasons why PySpark SparkConf
is used:
- Configuration of Spark settings:
SparkConf
allows the developer to set various Spark settings such as the number of cores, amount of memory, and number of executors. These settings can significantly affect the performance of the Spark application. - Flexibility in setting Spark properties: PySpark
SparkConf
allows developers to set Spark properties programmatically, which gives more flexibility than setting properties through configuration files. - Setting Spark properties in a specific context:
SparkConf
allows the developer to set Spark properties in a specific context, such as for a specific application, which ensures that the properties are only applied to that application. - Dynamically changing Spark properties:
SparkConf
also allows developers to dynamically change Spark properties during the runtime of a Spark application, which can be useful for optimizing performance based on the specific workload of the application.
Overall, SparkConf
provides an easy and flexible way to configure Spark settings for PySpark applications.
5. How will you create PySpark UDF?
To create a PySpark UDF (User-Defined Function), you can follow these steps:
- Import the required PySpark modules: You need to import
pyspark.sql.functions
andpyspark.sql.types
modules to create PySpark UDFs. - Define the Python function: You need to define a Python function that implements the logic of the UDF. The function should take one or more arguments and return a value.
- Define the UDF: You need to use the
pyspark.sql.functions.udf
function to define the UDF. This function takes two arguments – the Python function and the return type of the UDF. - Register the UDF: You need to register the UDF with Spark using the
DataFrame.withColumn
function. This function takes two arguments – the name of the new column and the UDF.
Here is an example of creating a PySpark UDF:
from pyspark.sql.functions import udf from pyspark.sql.types import IntegerType def square(x): return x * x square_udf = udf(square, IntegerType()) df = df.withColumn("squared_column", square_udf(df["column_name"]
In the example above, we define a Python function square
that takes one argument and returns the square of the argument. We then define the UDF square_udf
using the udf
function, specifying the Python function and the return type as IntegerType()
. Finally, we register the UDF with Spark using the withColumn
function to create a new column named "squared_column"
in the DataFrame df
.
Note that the return type of the UDF should match the type of the new column in the DataFrame. If the return type of the UDF is different, Spark will not be able to execute the UDF correctly.
6. What are the profilers in PySpark?
In PySpark, there are several profilers that can be used to analyze the performance of a Spark application. Here are a few of them:
- PySpark Profiler: PySpark Profiler is a web-based profiler that provides a detailed analysis of Spark application performance. It generates a range of visualizations, including graphs, heat maps, and tables, that help developers identify bottlenecks in their application. PySpark Profiler can also help to identify and diagnose issues related to data skew and partitioning.
- PySpark Job Profiler: PySpark Job Profiler is a command-line tool that can be used to analyze the performance of individual Spark jobs. It provides detailed information about the resources used by the job, including CPU, memory, and network usage. PySpark Job Profiler also provides statistics about the input and output data, as well as the time taken by each stage of the job.
- PySpark Monitoring UI: PySpark Monitoring UI is a web-based tool that provides real-time monitoring of a running Spark application. It provides detailed information about the resources used by the application, including CPU, memory, and network usage. PySpark Monitoring UI also provides statistics about the input and output data, as well as the time taken by each stage of the application.
- PySpark Executor Metrics: PySpark Executor Metrics is a collection of metrics that can be used to monitor the performance of individual Spark executors. These metrics include information about CPU and memory usage, garbage collection, and disk I/O. PySpark Executor Metrics can be useful for identifying issues related to resource utilization and garbage collection.
Overall, these profilers provide developers with powerful tools for analyzing the performance of Spark applications, identifying bottlenecks, and optimizing performance.
7. How to create SparkSession?
In PySpark, SparkSession
is the entry point to create a Spark application. You can create a SparkSession
object by following these steps:
- Import the PySpark module: You need to import the
pyspark.sql
module to create aSparkSession
. - Create a
SparkSession
object: You can create aSparkSession
object by calling theSparkSession.builder
method. This method returns aSparkSession.Builder
object that can be used to configure and create aSparkSession
. - Configure the
SparkSession
: You can configure theSparkSession
by calling various methods on theSparkSession.Builder
object. For example, you can set the master URL, application name, and other Spark configuration properties. - Create the
SparkSession
: You can create theSparkSession
by calling thegetOrCreate()
method on theSparkSession.Builder
object.
Here is an example of creating a SparkSession
in PySpark:
from pyspark.sql import SparkSession spark = SparkSession.builder \ .master("local[*]") \ .appName("My PySpark Application") \ .config("spark.some.config.option", "some-value") \ .getOrCreate()
In this example, we first import the SparkSession
module. We then create a SparkSession.Builder
object and configure it by setting the master URL to "local[*]"
, setting the application name to "My PySpark Application"
, and setting a Spark configuration property "spark.some.config.option"
to "some-value"
. Finally, we create the SparkSession
by calling the getOrCreate()
method.
Once you have created a SparkSession
, you can use it to create DataFrame
s and execute Spark operations on them.
8. What are the different approaches for creating RDD in PySpark?
The following image represents how we can visualize RDD creation in PySpark:
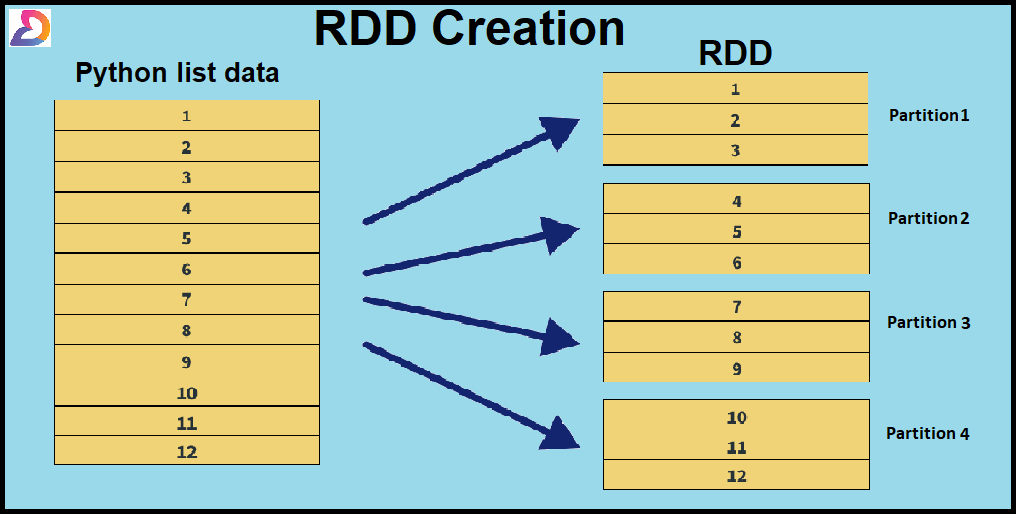
In the image, we see that the data we have is the list form and post converting to RDDs, we have it stored in different partitions.
In PySpark, there are three approaches for creating RDDs (Resilient Distributed Datasets):
- Creating RDD from a collection: You can create an RDD by parallelizing an existing Python collection such as a list, tuple or set. This is typically done using the
parallelize()
method of the SparkContext object. For example, you can create an RDD of integers as follows:
from pyspark import SparkContext sc = SparkContext("local", "RDD creation example") data = [1, 2, 3, 4, 5] rdd = sc.parallelize(data)
- Creating RDD from an external data source: You can create an RDD by reading data from an external data source such as a file or a database. This is typically done using the
textFile()
orwholeTextFiles()
method of the SparkContext object. For example, you can create an RDD of text lines from a file as follows:
from pyspark import SparkContext sc = SparkContext("local", "RDD creation example") rdd = sc.textFile("file.txt")
- Creating RDD from another RDD: You can create an RDD by applying transformations to an existing RDD. This is typically done using the transformation operations such as
map()
,filter()
,flatMap()
, andunion()
. For example, you can create a new RDD of squares from an existing RDD of integers as follows:
from pyspark import SparkContext sc = SparkContext("local", "RDD creation example") data = [1, 2, 3, 4, 5] rdd1 = sc.parallelize(data) rdd2 = rdd1.map(lambda x: x**2)
In this example, we create an RDD of integers (rdd1
) from a Python list, and then apply the map()
transformation to create a new RDD of squares (rdd2
). Note that the map()
transformation is lazy and does not execute until an action operation is performed on the RDD.
9. How can we create DataFrames in PySpark?
In PySpark, you can create DataFrames using different methods, such as:
Creating DataFrame from an RDD: You can create a DataFrame from an RDD by using the toDF()
method. For example:
from pyspark.sql import SparkSession spark = SparkSession.builder.appName("DataFrame creation example").getOrCreate() data = [("Alice", 25), ("Bob", 30), ("Charlie", 35)] rdd = spark.sparkContext.parallelize(data) df = rdd.toDF(["Name", "Age"]) df.show(
Creating DataFrame from a list of dictionaries: You can create a DataFrame from a list of dictionaries by using the createDataFrame()
method. For example:
from pyspark.sql import SparkSession spark = SparkSession.builder.appName("DataFrame creation example").getOrCreate() data = [{"Name": "Alice", "Age": 25}, {"Name": "Bob", "Age": 30}, {"Name": "Charlie", "Age": 35}] df = spark.createDataFrame(data) df.show(
Creating DataFrame from a CSV file: You can create a DataFrame from a CSV file by using the read.csv()
method. For example:
from pyspark.sql import SparkSession spark = SparkSession.builder.appName("DataFrame creation example").getOrCreate() df = spark.read.csv("data.csv", header=True, inferSchema=True) df.show(
In this example, we read a CSV file named data.csv
with a header row and infer the schema from the data. Note that the inferSchema
option is set to True
, which automatically infers the data types of the columns.
These are some of the common methods to create DataFrames in PySpark. Once you have created a DataFrame, you can perform various operations such as filtering, grouping, aggregating, joining, and more using the DataFrame API.
10. Is it possible to create PySpark DataFrame from external data sources?
Yes, it is possible to create a PySpark DataFrame from external data sources. PySpark supports reading data from various file formats, databases, and streaming sources.
Here are some examples of how you can create a PySpark DataFrame from external data sources:
- Reading from CSV file:
from pyspark.sql import SparkSession spark = SparkSession.builder.appName("DataFrame creation example").getOrCreate() df = spark.read.csv("file_path.csv", header=True, inferSchema=True
- Reading from JSON file:
from pyspark.sql import SparkSession spark = SparkSession.builder.appName("DataFrame creation example").getOrCreate() df = spark.read.json("file_path.json"
- Reading from Parquet file:
from pyspark.sql import SparkSession spark = SparkSession.builder.appName("DataFrame creation example").getOrCreate() df = spark.read.parquet("file_path.parquet"
- Reading from a database:
from pyspark.sql import SparkSession spark = SparkSession.builder.appName("DataFrame creation example").getOrCreate() jdbc_url = "jdbc:mysql://localhost:3306/mydb" table_name = "my_table" properties = {"user": "my_user", "password": "my_password"} df = spark.read.jdbc(url=jdbc_url, table=table_name, properties=propertie
In this example, we read data from a MySQL database table named my_table
using the JDBC API. Note that you need to provide the JDBC URL, table name, and authentication credentials as properties.
There are many more data sources supported by PySpark such as Avro, ORC, Delta Lake, Kafka, and more. You can refer to the PySpark documentation for more details on reading data from external sources.
11. What do you understand by Pyspark’s startsWith() and endsWith() methods?
startsWith()
and endsWith()
are methods in PySpark that can be used to check if a string column in a PySpark DataFrame starts or ends with a given string.
startsWith()
method:
The startsWith()
method can be used to check if a string column in a PySpark DataFrame starts with a given string. The syntax of the startsWith()
method is:
df.filter(df.<column_name>.startswith("<string>"))
For example, let’s say we have a DataFrame df
with a column named “Name” containing names of people. We can use the startsWith()
method to filter out names that start with the letter “A” as follows:
df.filter(df.Name.startswith("A"))
This will return a new DataFrame containing only the rows where the “Name” column starts with the letter “A”.
endsWith()
method:
The endsWith()
method can be used to check if a string column in a PySpark DataFrame ends with a given string. The syntax of the endsWith()
method is:
df.filter(df.<column_name>.endswith("<string>"))
For example, let’s say we have a DataFrame df
with a column named “Email” containing email addresses. We can use the endsWith()
method to filter out email addresses that end with “.com” as follows:
df.filter(df.Email.endswith(".com"))
This will return a new DataFrame containing only the rows where the “Email” column ends with “.com”.
Both startsWith()
and endsWith()
methods can be used with other PySpark DataFrame methods and transformations to perform more complex operations on string columns.
12. What is PySpark SQL?
PySpark SQL is a module in PySpark that provides a programming interface to work with structured and semi-structured data using SQL (Structured Query Language) queries. It provides a DataFrame API that allows developers to query and manipulate data using SQL statements, similar to the way we work with data in traditional relational databases.
The PySpark SQL module is built on top of the Spark SQL engine, which is an optimized and distributed SQL engine that supports various data sources and formats such as Parquet, ORC, Avro, JSON, and JDBC data sources. It also provides support for SQL functions, window functions, and user-defined functions (UDFs) that can be used to transform and aggregate data.
With PySpark SQL, developers can perform complex data analysis tasks using SQL queries, without needing to write complex and time-consuming MapReduce code. PySpark SQL also provides integration with other PySpark modules such as PySpark Streaming and PySpark MLlib, which makes it easy to build end-to-end data pipelines for large-scale data processing and machine learning.
13. How can you inner join two DataFrames?
In PySpark, we can perform an inner join on two DataFrames using the join()
method. The join()
method takes two DataFrames and a join condition as parameters and returns a new DataFrame that contains the rows with matching keys in both DataFrames.
Here is an example of how to perform an inner join on two DataFrames in PySpark:
from pyspark.sql.functions import col # Create the first DataFrame df1 = spark.createDataFrame([(1, 'Alice'), (2, 'Bob'), (3, 'Charlie')], ['id', 'name']) # Create the second DataFrame df2 = spark.createDataFrame([(1, 'New York'), (2, 'San Francisco'), (4, 'Boston')], ['id', 'city']) # Inner join the two DataFrames on the 'id' column joined_df = df1.join(df2, on='id', how='inner') # Show the result joined_df.sho
In this example, we first create two DataFrames df1
and df2
. The first DataFrame has columns ‘id’ and ‘name’, and the second DataFrame has columns ‘id’ and ‘city’. We then use the join()
method to perform an inner join on the ‘id’ column of both DataFrames. The resulting DataFrame joined_df
will contain only the rows with matching ‘id’ values in both DataFrames.
We can also specify the join condition using the col()
function. For example, if we want to perform an inner join on two DataFrames where the ‘id’ column of df1
is equal to the ‘person_id’ column of df2
, we can use the following code:
# Inner join the two DataFrames on the 'id' and 'person_id' columns joined_df = df1.join(df2, col('id') == col('person_id'), how='inner')
14. What do you und erstand by Pyspark Streaming? How do you stream data using TCP/IP Protocol?
PySpark Streaming is a module in PySpark that allows developers to process and analyze real-time streaming data from various sources such as Kafka, Flume, Twitter, and HDFS. It provides a high-level API for building scalable, fault-tolerant, and real-time streaming applications that can process data in mini-batches.
To stream data using TCP/IP Protocol in PySpark, we can use the socketTextStream()
method of the StreamingContext
object. This method creates a data stream from a TCP/IP socket by listening to a specific hostname and port.
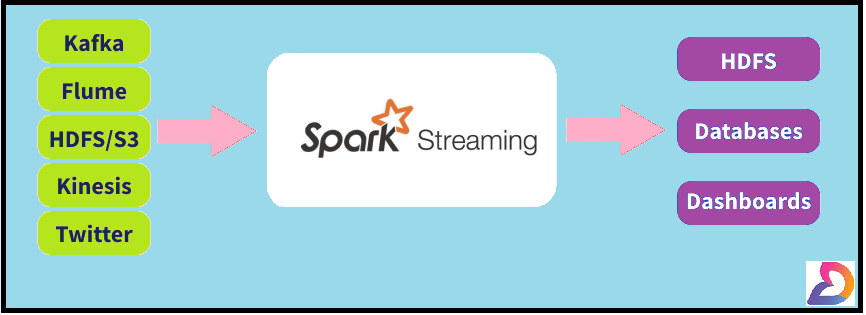
Here is an example of how to stream data using TCP/IP Protocol in PySpark:
from pyspark.streaming import StreamingContext # Create a local StreamingContext with two execution threads and a batch interval of 1 second ssc = StreamingContext(spark.sparkContext, 1) # Create a DStream that reads data from a TCP socket lines = ssc.socketTextStream("localhost", 9999) # Split the lines into words words = lines.flatMap(lambda line: line.split(" ")) # Count each word in each batch wordCounts = words.map(lambda word: (word, 1)).reduceByKey(lambda a, b: a + b) # Print the word counts wordCounts.pprint() # Start the streaming context ssc.start() # Wait for the streaming to finish ssc.awaitTermina
In this example, we create a StreamingContext
with a batch interval of 1 second. We then create a DStream using the socketTextStream()
method by specifying the hostname and port number to listen to. The lines in the stream are then split into words, and we count the frequency of each word using reduceByKey()
. Finally, we print the word counts using the pprint()
method and start the streaming context using start()
.
To send data to this streaming application, we can use the nc
(netcat) command in the terminal to create a TCP/IP socket and send data to it. Here is an example of how to send data to the streaming application using nc
:
$ nc -lk 9999 hello world this is a test
In this example, we create a TCP/IP socket on port 9999 using the -l
option of nc
, and we send two lines of text to it. The streaming application will receive these lines, split them into words, and count the frequency of each word in each batch. The results will be printed to the console every second, as specified by the batch interval.
15. What would happen if we lose RDD partitions due to the failure of the worker node?
If we lose RDD partitions due to the failure of a worker node in PySpark, Spark’s fault-tolerant mechanism will kick in and the lost partitions will be recomputed automatically on other available nodes. This process is known as RDD recovery, which is a crucial component of Spark’s fault-tolerant design.
When a worker node fails, Spark will detect the failure and mark the RDD partitions stored on that node as lost. The driver node will then send a message to the remaining worker nodes requesting them to recompute the lost partitions. The worker nodes that receive this message will check if they have the necessary data to recompute the lost partitions. If so, they will start recomputing the lost partitions and store the results on their local disk.
If the lost partitions cannot be recomputed from the available data on the remaining nodes, Spark will fall back to reading the data from the storage system (such as HDFS) where the original data was stored, and then recompute the lost partitions. This ensures that even if multiple worker nodes fail simultaneously, Spark can still recover the lost partitions and continue processing the data.
16. Tell me a few algorithms which support PySpark.
PySpark supports a wide range of machine learning and data mining algorithms, including but not limited to:
- Linear Regression
- Logistic Regression
- Decision Trees
- Random Forest
- Gradient Boosted Trees
- Naive Bayes
- Support Vector Machines (SVM)
- K-Means Clustering
- Hierarchical Clustering
- Principal Component Analysis (PCA)
- Singular Value Decomposition (SVD)
- Association Rule Mining (ARM)
- Collaborative Filtering (CF)
- Deep Learning Algorithms (e.g., Convolutional Neural Networks, Recurrent Neural Networks, etc.)
These algorithms are implemented in PySpark’s Machine Learning Library (MLlib), which provides a scalable and distributed platform for training and deploying machine learning models on large datasets. PySpark also supports external machine learning libraries such as TensorFlow, Keras, and Scikit-Learn through its integration with Python.
17. Tell me the different SparkContext parameters.
SparkContext is the entry point to any PySpark application, and it takes several parameters to configure the runtime environment. Some of the most commonly used SparkContext parameters are:
- appName: The name of the Spark application.
- master: The URL of the cluster manager to connect to (e.g., “local” for a local mode or “spark://master:7077” for a cluster mode).
- sparkHome: The path to the Spark installation directory.
- pyFiles: A list of Python files to be distributed to the worker nodes.
- environment: A dictionary of environment variables to be set on the worker nodes.
- executorMemory: The amount of memory to allocate to each executor process (e.g., “2g” for 2 gigabytes).
- numExecutors: The number of executor processes to start on the worker nodes.
- executorCores: The number of CPU cores to allocate to each executor process.
- driverMemory: The amount of memory to allocate to the driver process (e.g., “1g” for 1 gigabyte).
- driverCores: The number of CPU cores to allocate to the driver process.
These parameters can be passed to the SparkContext constructor as key-value pairs, like this:
from pyspark import SparkContext, SparkConf conf = SparkConf().setAppName("MyApp").setMaster("local[*]") sc = SparkContext(conf=conf)
In this example, we are setting the application name to “MyApp” and the master URL to “local[*]”, which means that we want to run the application in local mode using all available CPU cores.
18. What is RDD? How many types of RDDs are in PySpark?
RDD stands for Resilient Distributed Dataset, which is a fundamental data structure in PySpark. It is an immutable distributed collection of objects that can be processed in parallel across a cluster of machines.
PySpark supports two types of RDDs:
- Parallelized Collections: RDDs that are created by parallelizing an existing collection in the driver program.
- Hadoop Datasets: RDDs that are created by loading data from Hadoop Distributed File System (HDFS) or other storage systems that Hadoop supports, such as Amazon S3, Cassandra, and HBase.
19. Tell me the different cluster manager types in PySpark.
PySpark supports three types of cluster managers that can be used to manage resources and allocate tasks to workers in a distributed environment:
- Standalone cluster manager: This is the default cluster manager in PySpark and is designed to work only with Spark. It has a simple architecture and is easy to set up, making it ideal for small or medium-sized clusters.
- Apache Mesos: This is a general-purpose cluster manager that can be used to run other distributed applications besides Spark. Mesos provides fine-grained resource allocation and supports dynamic partitioning of resources.
- Hadoop YARN: This is the cluster manager used by Hadoop and is designed to support multiple applications running simultaneously on the same cluster. YARN provides resource allocation and scheduling capabilities, making it suitable for large-scale clusters with many different types of workloads.
20. What do you understand about PySpark DataFrames?
PySpark DataFrames are distributed collection of data organized into named columns, which are similar to tables in a relational database. They are designed to handle large-scale datasets and provide a more high-level API than RDDs, which makes it easier to manipulate data using SQL-like queries and DataFrame operations.
PySpark DataFrames are immutable, meaning that once they are created, they cannot be modified. Instead, operations on DataFrames create new DataFrames. PySpark DataFrames also offer support for a variety of data formats, including CSV, JSON, and Parquet, as well as integration with external data sources like Hadoop Distributed File System (HDFS), Apache Cassandra, and Apache HBase.
DataFrames in PySpark are built on top of RDDs and use lazy evaluation, which means that transformations on a DataFrame are not executed immediately but are queued up and executed in a single pass when an action is performed. This enables PySpark to optimize the execution plan and avoid unnecessary computation, resulting in faster processing times.
21. Explain SparkSession in PySpark.
SparkSession is the entry point to PySpark and is responsible for managing the lifecycle of a PySpark application. It is a unified interface that provides access to all the functionalities of Spark and is used to create DataFrame, Dataset, and RDDs.
SparkSession provides a convenient way to create and configure Spark-related settings such as the number of executors, the amount of memory allocated per executor, and other Spark-related configuration parameters.
In addition to the Spark-related settings, SparkSession also provides the ability to connect to external data sources such as Hive, JDBC, and Cassandra. It allows users to interact with data stored in external data sources as if they were DataFrames in PySpark.
SparkSession is a singleton object, meaning that only one instance of SparkSession can be active in a single JVM. It can be accessed by calling the SparkSession.builder()
method and then configuring it using the various methods available on the builder object. Once the SparkSession object is configured, it can be used to create and manipulate DataFrames and perform various other PySpark operations.
22. What do you know about PySpark UDF?
PySpark UDF stands for User-Defined Function, which is a feature in PySpark that allows users to define their own custom functions to operate on PySpark DataFrames. UDFs are useful when the built-in functions in PySpark are not sufficient to perform the required operation.
PySpark UDFs can be defined using Python, Java, or Scala, but in this case, I will focus on Python UDFs. Python UDFs can be created using the udf()
function provided by the pyspark.sql.functions
module.
To create a Python UDF, you define a Python function that takes one or more input arguments and returns a single output value. You then register this function with PySpark using the udf()
function, specifying the input and output data types.
Once the UDF is defined, it can be applied to a PySpark DataFrame using the withColumn()
method. This method creates a new column in the DataFrame by applying the UDF to each row of the existing column.
It is important to note that PySpark UDFs can have a significant performance impact on the PySpark application, especially when the UDF is applied to a large DataFrame. Therefore, it is recommended to use built-in PySpark functions wherever possible, and only use UDFs when necessary.
23. How is PySpark exposed in Big Data?
PySpark is a Python API for Apache Spark, which is a distributed computing framework designed to process large-scale data sets. PySpark provides a simple and intuitive interface to interact with Spark, making it a popular choice for data scientists and engineers working in the Big Data domain.
PySpark can be used to process data stored in various data sources including Hadoop Distributed File System (HDFS), Apache Cassandra, and Amazon S3. It also supports a variety of data formats such as CSV, JSON, and Parquet.
PySpark is typically deployed in a distributed environment, with a cluster of machines working together to process data. The data is partitioned across the cluster, and each machine processes a subset of the data in parallel.
PySpark can be exposed in Big Data in several ways. One way is to use PySpark in conjunction with other Big Data tools like Apache Hadoop, Apache Hive, and Apache Pig. This allows users to process data using PySpark and store the results in a distributed file system like HDFS.
Another way to expose PySpark in Big Data is to use cloud-based solutions like Amazon EMR, Google Dataproc, and Microsoft Azure HDInsight. These services provide a managed environment for running PySpark on Big Data, allowing users to focus on their data processing tasks without worrying about managing the underlying infrastructure.
24. What do you know about the PySpark DAGScheduler?
The PySpark DAGScheduler is an essential component of Apache Spark’s execution engine. DAG stands for Directed Acyclic Graph, which is a data structure used to represent a series of transformations that need to be applied to a dataset to produce the final output. The DAGScheduler is responsible for optimizing and scheduling these transformations and actions on a PySpark application’s RDDs and DataFrames.
When a PySpark application is executed, the transformations on the RDDs and DataFrames are not executed immediately but instead are added to a DAG of transformations. The DAGScheduler then analyzes this DAG to optimize the execution plan, grouping operations together where possible and reordering operations for maximum efficiency.
The DAGScheduler also handles fault tolerance, which is critical in a distributed computing environment where nodes can fail at any time. In the event of a node failure, the DAGScheduler will reschedule the failed tasks on other nodes to ensure that the PySpark application can continue running without interruption.
Once the DAGScheduler has optimized the execution plan, it hands off the execution to the PySpark TaskScheduler, which is responsible for launching tasks on the worker nodes and managing their execution.
25. Which workflow do we need to follow in PySpark?
When working with PySpark, it is recommended to follow a standard workflow that includes several key steps:
- Initialize SparkSession: The first step is to create a SparkSession, which is the entry point for any PySpark application. The SparkSession provides a unified API for working with data across multiple data sources and processing engines.
- Load data: Next, you need to load data into PySpark. This could involve reading data from files or databases, or creating RDDs or DataFrames from scratch.
- Transform data: Once you have loaded the data, you can perform various transformations on it using PySpark’s built-in functions or user-defined functions. These transformations could involve filtering, aggregating, joining, or manipulating the data in various ways.
- Cache data: PySpark provides an efficient caching mechanism that can be used to store intermediate results in memory. Caching can speed up iterative algorithms that require multiple passes over the same data.
- Execute actions: After transforming the data, you can execute actions to produce the final results. Actions are operations that trigger the computation of the DAG, such as counting the number of rows, writing the data to a file or database, or collecting the results into a local variable.
- Optimize performance: Throughout the workflow, it is important to optimize the performance of the PySpark application. This can involve tuning various configuration parameters, caching frequently used data, and using appropriate data partitioning strategies.
- Handle errors: Finally, it is important to handle errors and exceptions that may occur during the PySpark application’s execution. This could involve adding error handling code or retrying failed tasks in case of node failures or other issues.
26. Tell me how RDD is created in PySpark?
RDDs (Resilient Distributed Datasets) are the fundamental data structure in PySpark, representing a fault-tolerant collection of elements that can be processed in parallel across a distributed cluster.
There are two ways to create RDDs in PySpark:
- Parallelizing an existing collection: You can create an RDD from an existing Python collection (e.g., a list, tuple, or set) by calling the
sc.parallelize()
method. This method distributes the elements of the collection across the cluster and creates an RDD.
Here’s an example:
from pyspark.sql import SparkSession # create a SparkSession spark = SparkSession.builder.appName("create-rdd").getOrCreate() # create an RDD from a list of numbers rdd = spark.sparkContext.parallelize([1, 2, 3, 4, 5]
- Loading data from an external data source: You can also create an RDD by loading data from an external data source such as HDFS, S3, or a database. PySpark supports a variety of data formats, including CSV, JSON, and Parquet, and provides methods for loading data from each of these formats.
Here’s an example:
from pyspark.sql import SparkSession # create a SparkSession spark = SparkSession.builder.appName("create-rdd").getOrCreate() # create an RDD by loading a text file rdd = spark.sparkContext.textFile("hdfs://path/to/file.txt"
In both cases, PySpark distributes the elements of the RDD across the cluster and partitions them into smaller chunks that can be processed in parallel. Once an RDD is created, you can perform various transformations and actions on it using PySpark’s API to process the data in parallel.
27. Can we create a Data frame using an external database?
We can create the data frame locally in HDFC, HBase, MySQL, and any cloud.
28. Explain Add method.
In PySpark, the add method is used to add two numerical values or columns. This method is primarily used in the pyspark.sql.functions module.
The add method can take two arguments: col1 and col2. These arguments can be either columns or numerical values. If the arguments are columns, then the add method adds the values of the two columns element-wise. If the arguments are numerical values, then the add method simply adds the two values together.
Here is an example of using the add method in PySpark:
from pyspark.sql.functions import col df = spark.createDataFrame([(1, 2), (3, 4)], ["col1", "col2"]) # add two columns df.withColumn("sum", col("col1") + col("col2")).show() # add two numerical values x = 1 y = 2 result = x + y print(resul
In the above example, we create a PySpark DataFrame with two columns (col1 and col2). We then use the withColumn method to add a new column sum, which is the element-wise sum of col1 and col2.
We also show an example of adding two numerical values using the + operator. The result of the addition is stored in the result variable.
29. Do you think PySpark is similar to SQL?
PySpark and SQL are related but distinct technologies that serve different purposes, so while there are similarities, they are not identical.
PySpark is a Python library used for distributed computing on big data sets, based on the Apache Spark framework. PySpark provides a high-level API that allows you to write parallel data processing jobs that run on a cluster of machines, and it offers many built-in functions for data manipulation and analysis. It uses a programming language (Python) to perform distributed processing.
SQL, on the other hand, is a language used to manage and manipulate data in a relational database management system (RDBMS). SQL is used to create, modify, and query tables and their contents in a database. SQL is a declarative language, meaning you tell the system what you want to accomplish and it figures out how to do it.
While PySpark can perform similar operations to SQL, such as filtering, aggregating, and joining data, it does so using a programming language rather than a declarative language. PySpark can be more flexible than SQL, as it allows for custom functions and operations that may not be available in SQL. However, SQL can be easier to learn and use for simple queries and operations.
30. Why use Akka in PySpark?
Akka is a message-driven, actor-based concurrency framework for building highly concurrent, distributed, and fault-tolerant systems. PySpark, on the other hand, is a Python library for distributed computing on big data sets, based on the Apache Spark framework. So, the question of why to use Akka in PySpark depends on what you want to achieve.
One possible use case for using Akka in PySpark is to build a fault-tolerant and scalable messaging system for communication between distributed PySpark workers. Akka can be used to build an actor system that receives and processes messages asynchronously, which can help reduce the overhead of managing communication and coordination between PySpark workers. This can lead to improved performance and scalability, especially in scenarios where there are a large number of PySpark workers processing data in parallel.
Another use case for using Akka in PySpark is to implement custom parallel algorithms or distributed machine learning models that require more fine-grained control over the distributed processing. Akka can be used to build custom actors that implement specific computation steps or algorithms, which can be coordinated to perform complex distributed computations. This can enable you to implement custom distributed processing logic that may not be possible with the built-in PySpark functions.