
Apache Spark is an open-source big data processing framework that provides powerful tools for data analysis and processing. It offers two core data structures for processing big data:
- RDDs (Resilient Distributed Datasets) and
- DataFrames.
Both RDDs and DataFrames provide the ability to process big data in a distributed environment, but they differ in their design, functionality, and performance!
RDD (Resilient Distributed Datasets)
It is a fundamental data structure in Apache Spark, a popular big data processing framework. RDDs are designed to provide fast and scalable processing of large datasets by distributing the data across multiple nodes in a cluster.
RDDs are partitioned, immutable collections of objects that are stored across the nodes in the cluster. These objects can be of any type including integers, strings, tuples, and more. RDDs provide a set of high-level transformations and actions that allow users to perform complex data processing tasks with ease.
One of the key benefits of RDDs is that they are resilient, meaning that they can automatically recover from node failures. In case of a node failure, Spark will automatically re-compute the data that was stored on the failed node, ensuring that the data remains available and accessible.
Here is the code for word count in PySpark using RDDs:
from pyspark import SparkContext, SparkConf # Initialize SparkContext conf = SparkConf().setAppName("WordCountRDD") sc = SparkContext(conf=conf) # Read text file and create RDD text_file = sc.textFile("sample_text.txt") # Perform word count operation word_counts = text_file.flatMap(lambda line: line.split(" ")) \ .map(lambda word: (word, 1)) \ .reduceByKey(lambda a, b: a + b) # Save the result word_counts.saveAsTextFile("word_count_result_rdd") # Stop SparkConsc.stop()
Once an RDD has been created, users can perform a wide range of transformations and actions on it. Some of the common transformations include map
, filter
, and reduceByKey
. Actions include count
, first
, take
, and collect
.
DataFrames
DataFrames in PySpark is a distributed collection of data organized into named columns. They are designed to provide a higher level of abstraction compared to RDDs and are optimized for performance and ease of use. Unlike RDDs, DataFrames are able to handle both structured and semi-structured data, making it possible to work with a wider range of data types
DataFrames in PySpark are built on top of Spark SQL and can be efficiently processed using Spark’s optimized execution engine. They provide a convenient way to perform operations on data using Spark’s built-in functions, as well as user-defined functions (UDFs) written in Python or Scala. DataFrames are also optimized for performance, as they take advantage of Spark’s built-in optimizations such as predicate pushdown, column pruning, and data skipping.
Here is the code for word count in PySpark using DataFrames:
from pyspark.sql import SparkSession # Initialize SparkSession spark = SparkSession.builder.appName("WordCountDF").getOrCreate() # Read text file and create DataFrame text_df = spark.read.text("sample_text.txt") # Perform word count operation word_counts_df = text_df.select(explode(split(text_df.value, " ")).alias("word")) \ .groupBy("word") \ .count() # Save the result word_counts_df.write.format("csv").save("word_count_result_df") # Stop SparkSession spark.stop()
DataFrames in PySpark provide a convenient way to work with structured data, and they have several features that make them well-suited for big data processing and analytics. Some of these features include:
- Support for multiple data sources: DataFrames can be created from a variety of data sources, including Apache Parquet, Apache Avro, Apache ORC, JSON, and CSV files.
- Query optimization: DataFrames are optimized for performance, as they take advantage of Spark’s built-in optimizations such as predicate pushdown, column pruning, and data skipping.
- Built-in functions: DataFrames provide a large number of built-in functions for working with data, such as filtering, aggregating, and transforming data.
- User-defined functions: DataFrames also support user-defined functions (UDFs), which can be written in Python or Scala.
Understanding the difference
One of the main differences between RDDs and DataFrames is their performance. DataFrames are optimized for performance, with built-in optimizations for common operations, such as filtering, grouping, and aggregating. Additionally, DataFrames can take advantage of Spark’s Catalyst Optimizer, which provides further performance optimizations. On the other hand, RDDs are more flexible, but their performance can suffer when dealing with complex data transformations and operations.
Another difference between RDDs and DataFrames is the way they handle data types. DataFrames provide a more rigid schema, with defined data types for each column. This makes it easier to work with the data, as the data types are known in advance. RDDs, on the other hand, do not have a defined schema, and data types must be inferred at runtime. This can make working with RDDs more difficult, as data type issues can arise when performing transformations and operations.
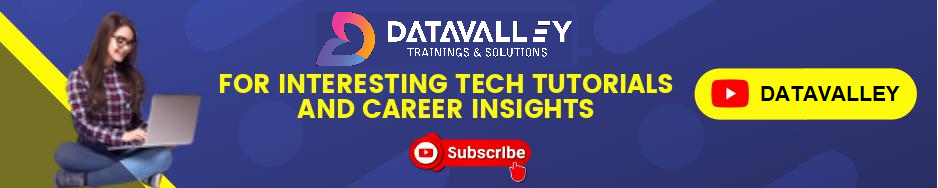
Here is a table defining the differences for you to understand at ease,
Feature | RDD | Dataframe |
Structure | Unstructured data | Structured data |
Schema | No schema defined | Has a schema defined |
Performance | Low-level API for performance optimization | Optimized for performance |
Type safety | Not type safe | Type safe |
Lazy evaluation | Fully evaluated before results are returned | Lazily evaluated for better performance |
Operations | A limited set of operations | Rich set of high-level operations |
API | Low-level API | High-level API |
Storage format | Can be stored in any format, such as text, sequence files, etc. | Optimized for columnar storage in formats such as Parquet, ORC, or Avro |
Optimization | Manual optimization needed | Automatically optimized by Catalyst optimizer |
Interoperability | Can be used with any data source | Can be used with any data source that can be represented as a table |
Conclusion
Both RDDs and DataFrames have their strengths and weaknesses, and the choice between them will depend on the specific requirements of your big data processing task. If you are working with structured data and require performance optimizations, DataFrames are likely the better choice. If you need more flexibility and the ability to perform complex data transformations, RDDs may be the better choice.
It’s important to understand the differences between RDDs and DataFrames so you can make an informed decision when choosing the appropriate data structure for your big data processing needs.