Create a Database in MongoDB
What is MongoDB?
MongoDB is a popular open-source NoSQL document-oriented database system that was developed by MongoDB Inc. It uses a flexible schema-less data model, which means that data can be stored in a variety of formats without a predefined structure. Instead of using tables and rows like traditional relational databases, MongoDB stores data as JSON-like documents with dynamic schemas, making it easier to store and retrieve complex data structures.
MongoDB’s document-oriented architecture allows for faster query execution and better scalability than traditional relational databases, especially when dealing with large amounts of unstructured data. MongoDB also supports dynamic indexing, replication, and automatic sharding, which enables it to scale horizontally across multiple servers.
MongoDB has become popular in recent years due to its ease of use, scalability, and flexibility. It is used by many large organizations, including eBay, Forbes, and The New York Times, as well as many smaller companies and startups. Its popularity has led to a large and active community of developers and users, who contribute to its development and provide support through online forums, user groups, and other resources.
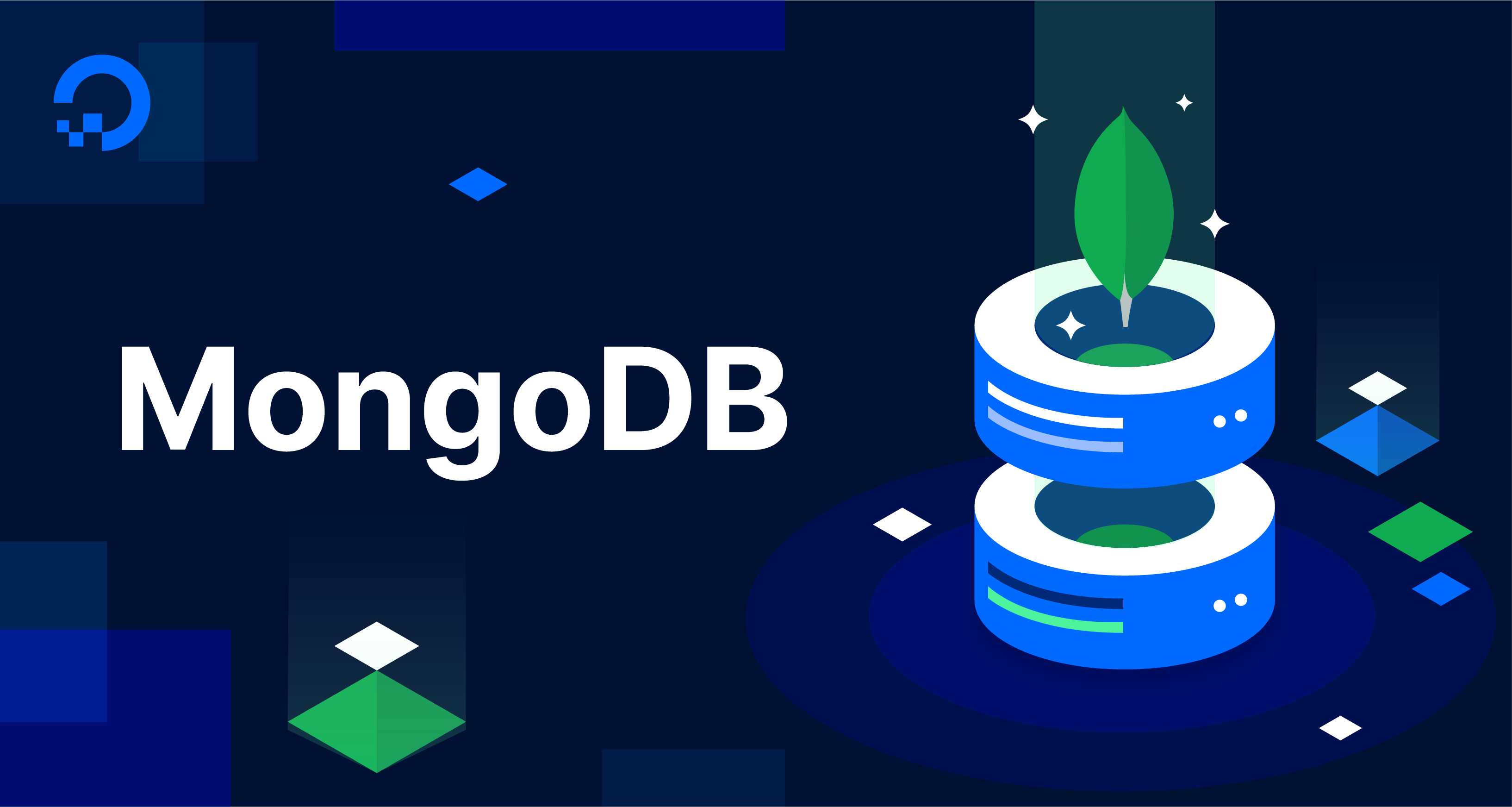
To create a database in MongoDB, follow these steps:
- Start by opening the MongoDB shell using the mongo command in your terminal or command prompt.
- To create a new database, use the command
use <database_name>
. For example, to create a database called “mydatabase”, typeuse mydatabase
. - Once you have created a new database, you can start adding data to it by creating collections. Collections are like tables in traditional relational databases. You can create a new collection using the
db.createCollection()
command. For example, to create a collection called “customers”, you would typedb.createCollection("customers")
. - You can then start inserting data into your collection using the
db.collection.insert()
command. For example, to insert a new document into your “customers” collection, you would typedb.customers.insert({ name: "John", age: 30, city: "New York" })
. - You can also retrieve data from your collection using the
db.collection.find()
command. For example, to find all documents in your “customers” collection, you would typedb.customers.find()
.
That’s it! You have now created a database in MongoDB and added data to it. Keep in mind that MongoDB is a flexible and scalable NoSQL database, so you can add and modify collections and documents as needed to meet your application’s requirements.
Creating a database in MongoDB is a simple process, but it’s important to understand when and how to create a new database to optimize the performance of your application.
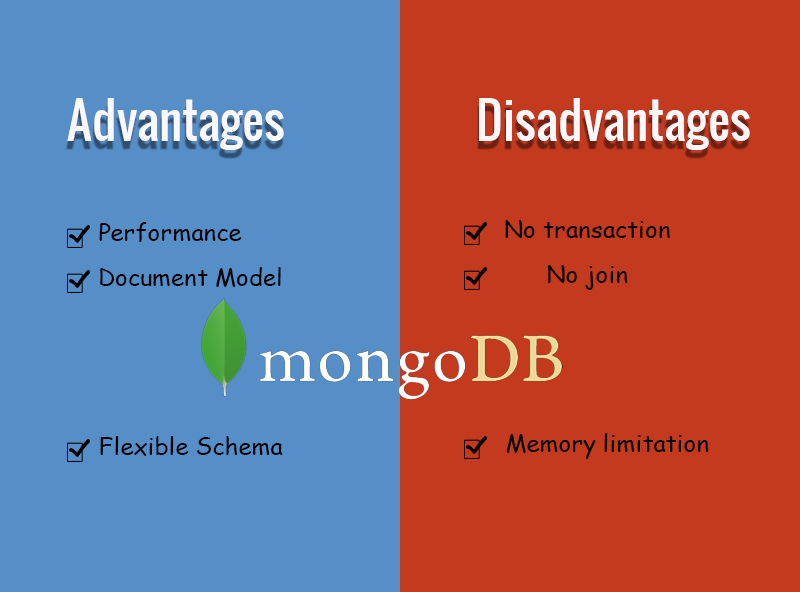
Here are some guidelines to consider:
When to Create a Database in MongoDB
Use a separate database for each application:
If you have multiple applications running on the same MongoDB server, it’s best to create a separate database for each application. This helps keep the data organized and makes it easier to manage.
Use a separate database for different environments:
If you have separate development, staging, and production environments, it’s best to use a separate database for each environment. This helps ensure that data from one environment doesn’t affect the others.
Use a separate database for different users:
If you have multiple users accessing your application, it’s best to use a separate database for each user. This helps keep the data secure and prevents unauthorized access.
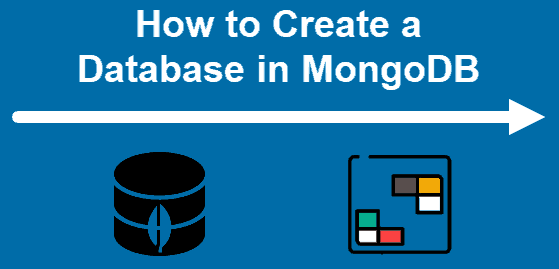
How to Create a Database in MongoDB?
To create a new database in MongoDB, you can use the following command in the MongoDB shell:
perl use <database_name>
Replace <database_name>
with the name of the database you want to create. If the database does not already exist, MongoDB will create it for you.
let me walk you through the process of creating a database in MongoDB with some code examples.
Step 1: Install MongoDB and Start the Server
Before we can create a database, we need to have MongoDB installed and running. You can download MongoDB from the official website and follow the installation instructions for your operating system. Once you have MongoDB installed, start the server by running the following command in your terminal or command prompt:
mongod
Step 2: Connect to MongoDB using the MongoDB Shell
Next, we need to connect to MongoDB using the MongoDB shell. Open a new terminal or command prompt window and run the following command:
mongo
This will start the MongoDB shell and connect you to the default test database.
Step 3: Create a New Database
To create a new database, use the use
command followed by the name of the database you want to create. For example, to create a database called “myblog”, run the following command:
perl use myblog
If the database does not already exist, MongoDB will create it for you.
Step 4: Create a Collection
Next, we need to create a collection within our new database. A collection is similar to a table in a relational database. To create a collection, we use the db.createCollection()
method. For example, to create a collection called “posts”, run the following command:
python db.createCollection("posts")
Step 5: Insert Data into the Collection
Now that we have a collection, we can insert data into it. To insert a document (or row) into the collection, we use the db.collection.insert()
method. For example, to insert a new blog post into our “posts” collection, run the following command:
php db.posts.insert({ title: "My First Blog Post", content: "Lorem ipsum dolor sit amet, consectetur adipiscing elit...", author: "John Doe", date: new Date() })
This will insert a new document into our “posts” collection with the specified fields.
Step 6: Query Data from the Collection
We can query the data from our collection using the db.collection.find()
method. For example, to retrieve all blog posts from our “posts” collection, run the following command:
db.posts.find()
This will return a cursor object containing all the documents in the “posts” collection. We can iterate over the cursor object to access each document individually.

That’s it! We have created a new database in MongoDB, created a collection within it, inserted data into the collection, and queried the data. This is just a basic example, but MongoDB provides many more features and options for working with data. With MongoDB, you have the flexibility to store and retrieve data in a way that best fits your application’s needs.
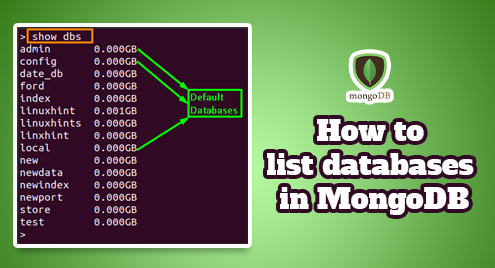
How to List Databases in MongoDB?
To list the databases in MongoDB, you can use the following command in the MongoDB shell:
sql show dbs
This command will display a list of all the databases that exist on the MongoDB server, including the default databases that are created when MongoDB is installed.
However, it’s important to note that this command only shows the databases that have data in them. If a database exists but has no collections or documents, it will not be listed by this command.
To list all the databases, including those that are empty, you can use the following command:
sql show databases
This command will display a list of all the databases that exist on the MongoDB server, regardless of whether they have data in them or not.
It’s important to note that the show databases
command does not provide any information about the collections or documents within each database. To view the collections in a database, you can switch to that database using the use
command and then use the show collections
command to list the collections.
perl use <database_name> show collections
Replace <database_name>
with the name of the database you want to view. This command will display a list of all the collections in the specified database.

In addition to the commands mentioned above, there are other ways to list databases and their contents in MongoDB, depending on your needs. Here are a few more options:
- List databases using the
db.adminCommand({listDatabases: 1})
command: This command returns a list of all the databases on the server, including those that are empty. - List the size of each database using the
db.stats()
command: This command returns a summary of statistics for the current database, including the size of the database in bytes. - List the collections in a database using the
db.getCollectionNames()
command: This command returns an array of the names of all the collections in the current database. - List the number of documents in a collection using the
db.collection.count()
command: This command returns the number of documents in the specified collection. - List the contents of a collection using the
db.collection.find()
command: This command returns all the documents in the specified collection.
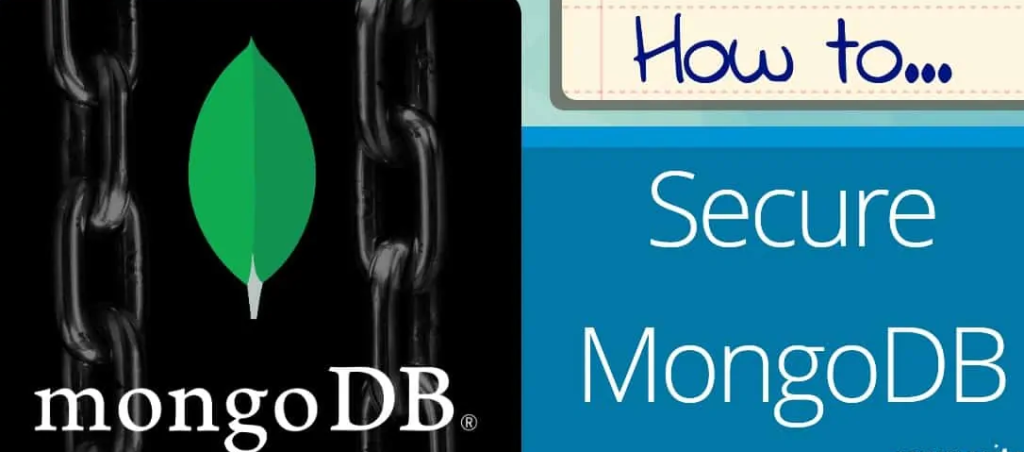
By using these commands, you can gain a better understanding of the databases and collections in your MongoDB instance and perform various operations on them.
How to secure database in mongodb?
MongoDB offers several built-in security features that you can use to secure your database. Here are some of the most important steps you can take to secure your MongoDB database:
Set up Authentication:
MongoDB allows you to set up authentication so that only authorized users can access your database. You can create user accounts and assign roles to those accounts to control what they can do in the database.
Use Encryption:
MongoDB offers several forms of encryption to protect data both in transit and at rest. You can use SSL/TLS to encrypt data in transit and enable encryption at rest using encryption algorithms like AES-256.
Implement Access control:
MongoDB offers a variety of access control mechanisms that you can use to restrict access to your database. You can use IP whitelisting, network interfaces, and other features to limit access to your database to only authorized users and applications.
Regularly update your MongoDB installation:
MongoDB regularly releases updates to address security vulnerabilities and other issues. Make sure to keep your MongoDB installation up to date to take advantage of the latest security features and bug fixes.
Monitor database activity:
Use MongoDB’s built-in auditing features or third-party tools to monitor database activity and detect suspicious or unauthorized activity. This can help you identify and respond to security incidents before they become serious.
By following these steps, you can improve the security of your MongoDB database and help protect your data from unauthorized access and other security threats.
Conclusion:
In conclusion, MongoDB is a popular NoSQL document-oriented database system that offers a flexible and scalable data model. To secure your MongoDB database, it’s important to implement best practices such as setting up authentication, using encryption, implementing access control, regularly updating your installation, and monitoring database activity. By taking these steps, you can help protect your data from unauthorized access and other security threats. It’s also important to stay up to date with the latest MongoDB security features and vulnerabilities and to follow industry best practices to ensure the security of your database. This is how we create Database in MongoDB.