NodeJs Interview Questions
1. What is Node.js? Where can you use it?
Node.js is a JavaScript runtime built on Chrome’s V8 JavaScript engine. It allows you to run JavaScript on the server side, creating server-side applications with JavaScript.
Node.js is commonly used for building backend services, such as APIs (Application Programming Interfaces) for web or mobile applications. It is also often used for real-time applications, such as chat or gaming applications, due to its ability to handle many connections concurrently.
In addition to building backend services, Node.js can also be used for tasks such as command-line tools, desktop applications, and even for creating servers for IoT (Internet of Things) devices.
Overall, Node.js is a powerful and flexible runtime that can be used for a wide range of purposes, making it a popular choice for developers building applications on the web.
2. Why use Node.js?
Node.js makes building scalable network programs easy. Some of its advantages include:
- It is generally fast
- It rarely blocks
- It offers a unified programming language and data type
- Everything is asynchronous
- It yields great concurrency
3. How does Node.js work?
A web server using Node.js typically has a workflow that is quite similar to the diagram illustrated below. Let’s explore this flow of operations in detail.
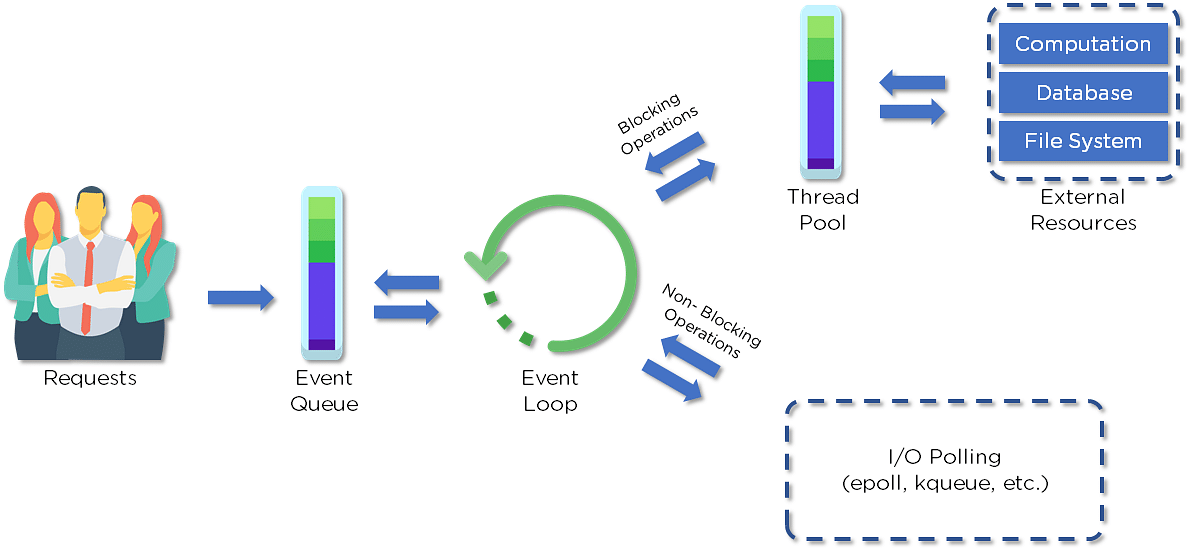
- Clients send requests to the webserver to interact with the web application. Requests can be non-blocking or blocking:
- Querying for data
- Deleting data
- Updating the data
- Node.js retrieves the incoming requests and adds those to the Event Queue
- The requests are then passed one-by-one through the Event Loop. It checks if the requests are simple enough not to require any external resources
- The Event Loop processes simple requests (non-blocking operations), such as I/O Polling, and returns the responses to the corresponding clients
A single thread from the Thread Pool is assigned to a single complex request. This thread is responsible for completing a particular blocking request by accessing external resources, such as computation, database, file system, etc.
Once the task is carried out completely, the response is sent to the Event Loop that sends that response back to the client.
4. Why is Node.js Single-threaded?
Node.js uses a single-threaded model with event looping. This means that it uses a single thread to execute JavaScript code, and relies on events and callbacks to handle concurrent operations.
The single-threaded model of Node.js makes it efficient for applications that require a lot of I/O (input/output) operations, such as reading and writing to databases, reading and writing to the file system, or making HTTP requests. These types of operations are often slower than in-memory operations and can block the execution of the program if they are not handled asynchronously.
In a single-threaded model, the event loop can handle these blocking operations asynchronously, allowing the program to continue executing while the I/O operation is performed in the background. This makes it possible to handle many concurrent connections and requests without the overhead of creating and managing multiple threads.
Overall, the single-threaded model of Node.js can be very efficient for certain types of applications, and is one of the key reasons why it has become so popular for building scalable and high-performance backend services.
5. If Node.js is single-threaded, then how does it handle concurrency?
The Multi-Threaded Request/Response Stateless Model is not followed by the Node JS Platform, and it adheres to the Single-Threaded Event Loop Model. The Node JS Processing paradigm is heavily influenced by the JavaScript Event-based model and the JavaScript callback system. As a result, Node.js can easily manage more concurrent client requests. The event loop is the processing model’s beating heart in Node.js.
6. Explain callback in Node.js.
In Node.js, a callback is a function that is passed as an argument to another function and is executed after the first function completes. Callbacks are commonly used in Node.js to handle asynchronous operations, such as reading and writing to the file system or making HTTP requests.
Here is an example of using a callback in Node.js to read a file from the file system:
Copy codeconst fs = require('fs');
fs.readFile('/path/to/file.txt', (err, data) => {
if (err) {
console.error(err);
return;
}
console.log(data);
});
In this example, the readFile
function reads a file from the file system asynchronously and accepts a callback function as its second argument. The callback function is called with an error object (err
) and the data from the file (data
) as arguments. If an error occurred during the operation, the error object will be set and the callback can handle the error appropriately. If the operation was successful, the callback can use the data from the file.
Callbacks are an important concept in Node.js and are used extensively to handle asynchronous operations. They allow Node.js to perform non-blocking I/O operations, which makes it possible to handle many concurrent connections and requests without the overhead of creating and managing multiple threads.
7. What are the advantages of using promises instead of callbacks?
- The control flow of asynchronous logic is more specified and structured.
- The coupling is low.
- We’ve built-in error handling.
- Improved readability.
8. How would you define the term I/O?
- The term I/O is used to describe any program, operation, or device that transfers data to or from a medium and to or from another medium
- Every transfer is an output from one medium and an input into another. The medium can be a physical device, network, or files within a system
9. How is Node.js most frequently used?
Node.js is widely used in the following applications:
- Real-time chats
- Internet of Things
- Complex SPAs (Single-Page Applications)
- Real-time collaboration tools
- Streaming applications
- Microservices architecture
10. Explain the difference between frontend and backend development?
Frontend Development | Backend Development |
---|---|
Concerned with the visual design and user experience of a website or application | Concerned with the server-side operations and logic that power a website or application |
Uses languages such as HTML, CSS, and JavaScript | Uses languages such as Python, Java, and PHP |
Runs in the user’s web browser | Runs on the server |
Deals with client-side rendering and user interaction | Deals with server-side rendering, data storage, and business logic |
Focuses on the “front end” of the application, which the user sees and interacts with | Focuses on the “back end” of the application, which powers the front end and handles interactions with servers, databases, and other resources |
Frontend developers are responsible for building the user interface and user experience of a website or application, using languages such as HTML, CSS, and JavaScript. They focus on the visual design and layout of the application, as well as the interactions and behaviors of the user interface.
Backend developers, on the other hand, are responsible for building the server-side logic and operations of a website or application. They use languages such as Python, Java, and PHP to build the backend services and APIs (Application Programming Interfaces) that power the frontend of the application. This includes tasks such as storing and retrieving data from databases, handling user authentication and authorization, and performing server-side rendering of pages.
Overall, frontend and backend development are two distinct areas of web development that involve different skills and technologies, but they often work closely together to build complete web applications.
11. What is NPM?
NPM stands for Node Package Manager, responsible for managing all the packages and modules for Node.js.
Node Package Manager provides two main functionalities:
- Provides online repositories for node.js packages/modules, which are searchable on search.nodejs.org
- Provides command-line utility to install Node.js packages and also manages Node.js versions and dependencies
12. What are the modules in Node.js?
Modules are like JavaScript libraries that can be used in a Node.js application to include a set of functions. To include a module in a Node.js application, use the require() function with the parentheses containing the module’s name.
Node.js has many modules to provide the basic functionality needed for a web application. Some of them include:
Core Modules | Description |
HTTP | Includes classes, methods, and events to create a Node.js HTTP server |
util | Includes utility functions useful for developers |
fs | Includes events, classes, and methods to deal with file I/O operations |
url | Includes methods for URL parsing |
query string | Includes methods to work with query string |
stream | Includes methods to handle streaming data |
zlib | Includes methods to compress or decompress files |
13. What is the purpose of the module .Exports?
In Node.js
, a module encapsulates all related codes into a single unit of code that can be parsed by moving all relevant functions into a single file. You may export a module with the module and export the function, which lets it be imported into another file with a needed keyword.
14. Why is Node.js preferred over other backend technologies like Java and PHP?
Some of the reasons why Node.js is preferred include:
- Node.js is very fast
- Node Package Manager has over 50,000 bundles available at the developer’s disposal
- Perfect for data-intensive, real-time web applications, as Node.js never waits for an API to return data
- Better synchronization of code between server and client due to same code base
- Easy for web developers to start using Node.js in their projects as it is a JavaScript library
15. What is the difference between Angular and Node.js?
Angular | Node.js |
It is a frontend development framework | It is a server-side environment |
It is written in TypeScript | It is written in C, C++ languages |
Used for building single-page, client-side web applications | Used for building fast and scalable server-side networking applications |
Splits a web application into MVC components | Generates database queries |
Also Read: What is Angular?
16. Which database is more popularly used with Node.js?
There are many different databases that can be used with Node.js, and the most popular choice will depend on the specific needs of your application. Some of the most popular databases used with Node.js include:
- MongoDB: MongoDB is a NoSQL database that stores data in a flexible, JSON-like format called BSON. It is popular with Node.js developers because it is easy to use and can be easily integrated with Node.js using the MongoDB driver.
- MySQL: MySQL is a popular open-source relational database management system. It is widely used with Node.js and can be easily integrated using the MySQL module.
- PostgreSQL: PostgreSQL is another popular open-source relational database management system. It is known for its reliability and performance, and can be easily used with Node.js using the pg module.
- SQLite: SQLite is a lightweight, embedded database that is often used for smaller applications or for testing. It can be easily integrated with Node.js using the sqlite3 module.
Ultimately, the choice of database will depend on the specific needs and requirements of your application. You may want to consider factors such as the type of data you need to store, the performance and scalability requirements of your application, and your team’s familiarity with different database technologies.
17. What are some of the most commonly used libraries in Node.js?
There are two commonly used libraries in Node.js:
- ExpressJS – Express is a flexible Node.js web application framework that provides a wide set of features to develop web and mobile applications.
- Mongoose – Mongoose is also a Node.js web application framework that makes it easy to connect an application to a database.
18. What are the pros and cons of Node.js?
Here is a table that summarizes some of the pros and cons of using Node.js:
Pros | Cons |
---|---|
– Node.js uses JavaScript, which is a familiar and popular language for many developers. This makes it easier for developers to learn and use Node.js. | – Node.js uses a single-threaded model, which can make it less efficient for certain types of applications that require a lot of CPU-intensive operations. |
– Node.js has a large and active community, which means there is a wealth of resources and libraries available for developers to use. | – Node.js can be more difficult to debug than some other languages, especially when dealing with asynchronous code. |
– Node.js is good at handling a large number of concurrent connections, making it suitable for real-time applications such as chat or gaming. | – Node.js may not be suitable for applications that require strong guarantees of consistency, such as financial transactions. |
– Node.js has good support for building RESTful APIs, making it a popular choice for building backend services. | – Node.js is not suitable for CPU-intensive applications, such as video encoding or image processing. |
Overall, Node.js is a popular and powerful runtime that is well-suited for building scalable and high-performance backend services and real-time applications. However, it may not be the best choice for every type of application, and it is important to consider the specific needs and requirements of your application when deciding whether to use Node.js.
19. What is the command used to import external libraries?
The “require” command is used for importing external libraries. For example – “var http=require (“HTTP”).” This will load the HTTP library and the single exported object through the HTTP variable.
Now that we have covered some of the important beginner-level Node.js interview questions let us look at some of the intermediate-level Node.js interview questions.
NodeJs Interview Questions and Answers For Intermediate Level
20. What does event-driven programming mean?
An event-driven programming approach uses events to trigger various functions. An event can be anything, such as typing a key or clicking a mouse button. A call-back function is already registered with the element executes whenever an event is triggered.
21. What is an Event Loop in Node.js?
Event loops handle asynchronous callbacks in Node.js. It is the foundation of the non-blocking input/output in Node.js, making it one of the most important environmental features.
22. Differentiate between process.nextTick() and setImmediate()?
process.nextTick()
and setImmediate()
are both methods that can be used to schedule a callback function to be executed asynchronously in Node.js. However, they operate slightly differently and are suitable for different use cases.
process.nextTick()
is a method that allows you to schedule a callback function to be executed on the next iteration of the event loop. This means that the callback will be executed as soon as possible, after the current synchronous code has completed.setImmediate()
is a method that allows you to schedule a callback function to be executed after the current iteration of the event loop. This means that the callback will be executed at the end of the current event loop cycle, after any I/O operations and timers have been completed.
Here is an example that illustrates the difference between process.nextTick()
and setImmediate()
:
Copy codeconsole.log('Start');
process.nextTick(() => {
console.log('Next tick');
});
setImmediate(() => {
console.log('Immediate');
});
console.log('End');
In this example, the output will be:
Copy codeStart
End
Next tick
Immediate
As you can see, the process.nextTick()
callback is executed before the setImmediate()
callback, even though it was scheduled after the setImmediate()
callback. This is because the process.nextTick()
callback is scheduled to be executed on the next iteration of the event loop, while the setImmediate()
callback is scheduled to be executed at the end of the current event loop cycle.
Overall, process.nextTick()
and setImmediate()
are both useful methods for scheduling asynchronous callbacks in Node.js, but they operate slightly differently and are suitable for different use cases. It is important to understand the differences between these methods and choose the one that is most appropriate for your specific needs.
23. What is an EventEmitter in Node.js?
- EventEmitter is a class that holds all the objects that can emit events
- Whenever an object from the EventEmitter class throws an event, all attached functions are called upon synchronously
24. What are the two types of API functions in Node.js?
In Node.js, there are two main types of API functions:
- Synchronous functions: These are functions that block the event loop and wait for the operation to complete before returning a result. Synchronous functions are generally easier to use and understand, but they can block the event loop and prevent other operations from being performed while the function is executing.
- Asynchronous functions: These are functions that do not block the event loop and return immediately, allowing other operations to be performed while the function is executing. Asynchronous functions are generally more efficient and perform better in Node.js, but they can be more difficult to use and understand, as they often rely on callbacks or promises to handle the result of the operation.
Here is an example of a synchronous function in Node.js:
Copy codeconst fs = require('fs');
const data = fs.readFileSync('/path/to/file.txt');
console.log(data);
And here is an example of an asynchronous function in Node.js:
Copy codeconst fs = require('fs');
fs.readFile('/path/to/file.txt', (err, data) => {
if (err) {
console.error(err);
return;
}
console.log(data);
});
Overall, it is important to understand the differences between synchronous and asynchronous functions and choose the appropriate type of function for your specific needs. Synchronous functions are generally easier to use, but asynchronous functions are generally more efficient and perform better in Node.js.
25. What is the package.json file?
The package.json file is the heart of a Node.js system. This file holds the metadata for a particular project. The package.json file is found in the root directory of any Node application or module
This is what a package.json file looks like immediately after creating a Node.js project using the command: npm init
You can edit the parameters when you create a Node.js project.
26. How would you use a URL module in Node.js?
The URL module in Node.js provides various utilities for URL resolution and parsing. It is a built-in module that helps split up the web address into a readable format.
27. What is the Express.js package?
Express.js is a popular web application framework for Node.js. It is designed to make it easy to build web applications and APIs (Application Programming Interfaces) with Node.js.
Express.js provides a set of functions and middleware that simplify the process of building web applications with Node.js. It allows you to define routes and handle HTTP requests, as well as provide functionality such as parsing request bodies, serving static files, and handling cookies.
Express.js is often used as the backend for web applications and is known for its simplicity and flexibility. It is easy to learn and can be used to build a wide range of web applications, from simple websites to complex APIs.
Overall, Express.js is a popular choice for building web applications and APIs with Node.js, and is widely used by developers around the world.
28. How do you create a simple Express.js application?
- The request object represents the HTTP request and has properties for the request query string, parameters, body, HTTP headers, and so on
- The response object represents the HTTP response that an Express app sends when it receives an HTTP request
29. What are streams in Node.js?
Streams are objects that enable you to read data or write data continuously.
There are four types of streams:
Readable – Used for reading operations
Writable − Used for write operations
Duplex − Can be used for both reading and write operations
Transform − A type of duplex stream where the output is computed based on input
30. How do you install, update, and delete a dependency?
31. How do you create a simple server in Node.js that returns Hello World?
- Import the HTTP module
- Use createServer function with a callback function using request and response as parameters.
- Type “hello world.”
- Set the server to listen to port 8080 and assign an IP address
32. Explain asynchronous and non-blocking APIs in Node.js.
- All Node.js library APIs are asynchronous, which means they are also non-blocking
- A Node.js-based server never waits for an API to return data. Instead, it moves to the next API after calling it, and a notification mechanism from a Node.js event responds to the server for the previous API call
33. How do we implement async in Node.js?
As shown below, the async code asks the JavaScript engine running the code to wait for the request.get() function to complete before moving on to the next line for execution.
34. What is a callback function in Node.js?
A callback is a function called after a given task. This prevents any blocking and enables other code to run in the meantime.
In the last section, we will now cover some of the advanced-level Node.js interview questions.
Node.js Interview Questions and Answers For Experienced Professionals
This section will provide you with the Advanced Node.js interview questions which will primarily help experienced professionals.
35. What is REPL in Node.js?
REPL stands for Read Eval Print Loop, and it represents a computer environment. It’s similar to a Windows console or Unix/Linux shell in which a command is entered. Then, the system responds with an output
36. What is the control flow function?
The control flow function is a piece of code that runs in between several asynchronous function calls.
37. How does control flow manage the function calls?
38. What is the difference between fork() and spawn() methods in Node.js?
fork() | spawn() |
fork() is a particular case of spawn() that generates a new instance of a V8 engine. | Spawn() launches a new process with the available set of commands. |
Multiple workers run on a single node code base for multiple tasks. | This method doesn’t generate a new V8 instance, and only a single copy of the node module is active on the processor. |
39. What is the buffer class in Node.js?
In Node.js, the Buffer
class is a global class that can be used to create a buffer object to store raw data in the form of binary data.
A buffer is a container for raw binary data. In Node.js, Buffer
objects are used to represent arbitrary arrays of data, whether it be ascii, binary or some other format. Buffers are similar to arrays of integers, but correspond to fixed-sized, raw memory allocation outside the V8 heap.
Buffers are useful when working with data that is not in a string form, or when the data is larger than the maximum size allowed for a string. For example, you might use a buffer to read a file, or to send binary data over a network connection.
Here is an example of creating and using a buffer:
Copy codeconst buf1 = Buffer.alloc(10);
// Creates a buffer of length 10, with all the elements initialized to 0
const buf2 = Buffer.from([1, 2, 3]);
// Creates a buffer containing [1, 2, 3]
const buf3 = Buffer.from('Hello World', 'utf8');
// Creates a buffer containing the utf8-encoded string 'Hello World'
buf3.toString('utf8');
// Returns 'Hello World'
buf3[0] = 97;
// Changes the first byte of the buffer to 97 (the ASCII value for 'a')
Note that in Node.js 13.2.0 and newer, the Buffer
class has been moved to the buffer
module. To use the Buffer
class in these versions, you will need to import it using:
Copy codeconst Buffer = require('buffer').Buffer;
40. What is piping in Node.js?
In Node.js, “piping” refers to the mechanism for connecting the output of one stream to the input of another stream, allowing data to be passed between the two streams. This is done using the pipe
method of a stream.
Piping is commonly used in Node.js to read data from a file and write it to another file or to send data over a network connection. It is also useful for transforming data as it is passed from one stream to another.
Here is an example of piping in Node.js:
Copy codeconst fs = require('fs');
const readStream = fs.createReadStream('input.txt');
const writeStream = fs.createWriteStream('output.txt');
readStream.pipe(writeStream);
In this example, data is read from the input.txt
file using a readable stream, and is then written to the output.txt
file using a writable stream. The pipe
method connects the output of the readStream
to the input of the writeStream
, allowing the data to be passed from the readStream
to the writeStream
.
You can also specify a transformation function as an argument to the pipe
method to transform the data as it is passed from one stream to another. For example:
Copy codeconst zlib = require('zlib');
const gzip = zlib.createGzip();
const readStream = fs.createReadStream('input.txt');
const writeStream = fs.createWriteStream('output.txt.gz');
readStream.pipe(gzip).pipe(writeStream);
In this example, the data is first passed through the gzip
stream, which compresses the data using gzip, and then it is written to the output.txt.gz
file using the writeStream
.
41. What are some of the flags used in the read/write operations in files?
42. How do you open a file in Node.js?
To open a file in Node.js, you can use the fs
(file system) module. The fs
module provides a variety of functions for working with files, including reading, writing, and modifying file contents.
Here is an example of how you can open a file in Node.js and read its contents:
Copy codeconst fs = require('fs');
fs.readFile('path/to/file.txt', 'utf8', (err, data) => {
if (err) {
console.error(err);
return;
}
console.log(data);
});
In this example, the readFile
function is used to read the contents of the file path/to/file.txt
. The second argument specifies the encoding of the file, in this case utf8
, which tells the function to return the data as a string. The third argument is a callback function that is called when the file has been read. If an error occurs, it is passed as the first argument to the callback. If the file is read successfully, the data is passed as the second argument to the callback.
You can also use the fs.open
function to open a file and obtain a file descriptor, which can be used to read or write the file. Here is an example of how you can use fs.open
to open a file and read its contents:
Copy codeconst fs = require('fs');
fs.open('path/to/file.txt', 'r', (err, fd) => {
if (err) {
console.error(err);
return;
}
const buffer = Buffer.alloc(1024);
fs.read(fd, buffer, 0, buffer.length, 0, (err, bytesRead, buffer) => {
if (err) {
console.error(err);
return;
}
console.log(buffer.toString('utf8', 0, bytesRead));
});
});
In this example, the fs.open
function is used to open the file path/to/file.txt
in read-only mode. The fs.read
function is then used to read the contents of the file into a buffer. The third argument to fs.read
is a callback function that is called when the file has been read. If an error occurs, it is passed as the first argument to the callback. The second and third arguments to the callback are the number of bytes read and the buffer, respectively.
43. What is callback hell?
- Callback hell, also known as the pyramid of doom, is the result of intensively nested, unreadable, and unmanageable callbacks, which in turn makes the code harder to read and debug
- improper implementation of the asynchronous logic causes callback hell
44. What is a reactor pattern in Node.js?
A reactor pattern is a concept of non-blocking I/O operations. This pattern provides a handler that is associated with each I/O operation. As soon as an I/O request is generated, it is then submitted to a demultiplexer
45. What is a test pyramid in Node.js?
46. For Node.js, why does Google use the V8 engine?
The V8 engine, developed by Google, is open-source and written in C++. Google Chrome makes use of this engine. V8, unlike the other engines, is also utilized for the popular Node.js runtime. V8 was initially intended to improve the speed of JavaScript execution within web browsers. Instead of employing an interpreter, V8 converts JavaScript code into more efficient machine code to increase performance. It turns JavaScript code into machine code during execution by utilizing a JIT (Just-In-Time) compiler, as do many current JavaScript engines such as SpiderMonkey or Rhino (Mozilla).
47. Describe Node.js exit codes.
48. Explain the concept of middleware in Node.js.
Middleware is a function that receives the request and response objects. Most tasks that the middleware functions perform are:
- Execute any code
- Update or modify the request and the response objects
- Finish the request-response cycle
- Invoke the next middleware in the stack
49. What are the different types of HTTP requests?
HTTP defines a set of request methods used to perform desired actions. The request methods include:
GET: Used to retrieve the data
POST: Generally used to make a change in state or reactions on the server
HEAD: Similar to the GET method, but asks for the response without the response body
DELETE: Used to delete the predetermined resource
50. How would you connect a MongoDB database to Node.js?
To create a database in MongoDB:
- Start by creating a MongoClient object
- Specify a connection URL with the correct IP address and the name of the database you want to create
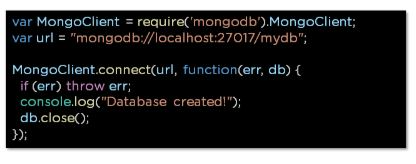
51. What is the purpose of NODE_ENV?
52. List the various Node.js timing features.
Node.js provides several functions for scheduling tasks to be executed at a later time. These functions are part of the timers
module, which is a built-in module in Node.js.
Here is a list of the various timing features in Node.js:
setTimeout
: schedules a function to be executed after a specified number of milliseconds have elapsed.setInterval
: schedules a function to be executed repeatedly at a specified interval, in milliseconds.clearTimeout
: cancels a timeout previously set withsetTimeout
.clearInterval
: cancels a timeout previously set withsetInterval
.setImmediate
: schedules a function to be executed as soon as the event loop is idle.clearImmediate
: cancels a timeout previously set withsetImmediate
.
Here is an example of using setTimeout
to schedule a function to be executed after a delay:
Copy codeconst timer = setTimeout(() => {
console.log('Hello, World!');
}, 1000);
In this example, the function () => console.log('Hello, World!')
will be executed after a delay of 1 second (1000 milliseconds).
You can use clearTimeout
to cancel a timeout that was previously set with setTimeout
:
Copy codeclearTimeout(timer);
This will prevent the scheduled function from being executed.
You can use setInterval
in a similar way to schedule a function to be executed repeatedly at a specified interval:
Copy codeconst interval = setInterval(() => {
console.log('Hello, World!');
}, 1000);
In this example, the function () => console.log('Hello, World!')
will be executed repeatedly every 1 second (1000 milliseconds). You can use clearInterval
to cancel a timeout that was previously set with setInterval
.
setImmediate
and clearImmediate
work in a similar way, but schedule a function to be executed as soon as the event loop is idle.
53. What is WASI, and why is it being introduced?
The WASI class implements the WASI system called API and extra convenience methods for interacting with WASI-based applications. Every WASI instance represents a unique sandbox environment. Each WASI instance must specify its command-line parameters, environment variables, and sandbox directory structure for security reasons.