What is Docker Container? Containerize Your Application Using Docker
Docker is an open-source platform that allows developers to build, package, and deploy applications in containers. Containerization using Docker is a process that involves bundling an application and all its dependencies into a single container. This container can be run on any machine that has Docker installed, making it easier to move the application from one environment to another.
In This Article the points to be focused on:
- What is a Docker Container?
- Why We Need Docker Containers?
- Learn How Docker Containers Are Better Than Virtual Machines
- How a Docker Container Works?
What is a Docker Container?
A Docker container is a lightweight and portable executable package that contains everything needed to run an application, including code, libraries, system tools, and runtime.
Containers are isolated from the host operating system and other containers, providing a consistent environment for applications to run regardless of the underlying infrastructure. Docker containers are based on Docker images, which are read-only templates that define the container’s contents and behavior.
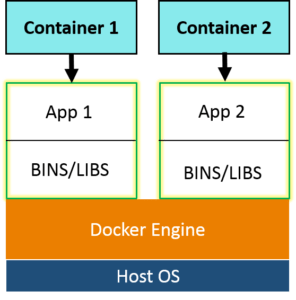
Docker containers can be easily created, deployed, and scaled across different environments, from development to production. They offer several benefits over traditional virtual machines, such as faster startup times, lower overhead, and greater flexibility.
Docker containers are commonly used in software development, testing, deployment, and microservices architectures. They provide a standardized way to package and distribute applications, making it easier to collaborate, share, and automate the software delivery process.
Example:
Here is an example of a simple Dockerfile
that containerizes a Node.js application:
# Use an official Node.js runtime as a parent image FROM node:14-alpine # Set the working directory to /app WORKDIR /app # Copy the current directory contents into the container at /app COPY . /app # Install any needed dependencies RUN npm install # Make port 3000 available to the world outside this container EXPOSE 3000 # Define environment variable ENV NODE_ENV=production # Run the app when the container launches CMD ["npm", "start"]
/app
, copies the contents of the current directory to the container, installs any needed dependencies using npm
, and exposes port 3000 to the outside world.
It also sets the NODE_ENV
environment variable to “production” and defines the command to run when the container launches, which is npm start
.
To build a Docker image from this Dockerfile, save it as “Dockerfile” in your Node.js project directory and run the following command:
docker build -t my-node-app .
This will build a Docker image called “my-node-app” based on the contents of the current directory. Once the image is built, you can run it as a container using the docker run
command.
Assuming that you have built the Docker image using the Dockerfile in the previous answer, you can run it as a container using the docker run
command, like this:
docker run -p 3000:3000 my-node-app
This command will start a new container from the “my-node-app” image and map port 3000 in the container to port 3000 on the host system, so that you can access the application from your web browser.
Once the container is running, you should be able to access the Node.js application by visiting http://localhost:3000
in your web browser.
You can also use other Docker commands to manage the container, such as docker stop
to stop the container, docker logs
to view the container’s logs, and docker exec
to execute commands inside the container.
Overall, Docker makes it easy to package and deploy Node.js applications in a consistent and reproducible way, making it easier to manage dependencies and deploy applications across different environments.
Why We Need Docker Containers?
Docker containers provide several benefits that make them a popular choice for software development and deployment. Here are some of the main reasons why we need Docker containers:
- Consistent and isolated environment: Docker containers provide a consistent and isolated environment for applications to run, which helps to prevent conflicts between dependencies and configuration issues. This makes it easier to build and deploy applications across different environments, from development to production.
- Portability: Docker containers are lightweight and portable, making them easy to move between different environments and platforms. This allows developers to build and test applications locally and then deploy them to production with confidence.
- Scalability: Docker containers can be easily scaled up or down to meet demand, making it easier to handle spikes in traffic or changes in resource requirements. This helps to reduce costs and improve application performance.
- Faster development and deployment: Docker containers allow developers to quickly build and test applications in a local environment, reducing the time and effort required to deploy applications to production.
- Improved collaboration: Docker containers provide a standardized way to package and distribute applications, making it easier for teams to collaborate and share code. This helps to improve the overall quality and reliability of the software.
Learn How Docker Containers Are Better Than Virtual Machines
Docker containers and virtual machines (VMs) are both technologies used to create isolated environments for applications to run. While both technologies offer advantages, Docker containers are generally considered to be better than virtual machines in several ways:
- Resource efficiency: Docker containers use a shared kernel with the host operating system, which makes them more lightweight and efficient than virtual machines. Containers only need to run the application and its dependencies, while virtual machines require a full guest operating system and hardware emulation layer.
- Faster startup and deployment times: Docker containers can be started up and deployed much faster than virtual machines, which can take several minutes to boot up. This makes it easier to deploy applications quickly and respond to changes in demand.
- Consistent environments: Docker containers provide a consistent environment for applications to run, making it easier to deploy applications across different environments and platforms. Virtual machines can have compatibility issues between different hardware and software configurations.
- Better resource utilization: Docker containers can run multiple applications on a single host, using resources more efficiently than virtual machines. Each virtual machine requires a full operating system, which can be wasteful when running multiple applications.
- Easier management: Docker containers are easier to manage than virtual machines, as they can be controlled with simple command-line tools and APIs. Virtual machines require more complex management tools and interfaces.
How a Docker Container Works?
Docker containers work by utilizing the Docker platform to isolate an application and its dependencies from the underlying system and other applications running on the same host.
Here are the key steps involved in how a Docker container works:
- Creating a Docker image: A Docker image is a read-only template that contains the application and its dependencies. The image is created using a Dockerfile, which is a script that specifies the necessary components and configurations.
- Running the Docker container: A Docker container is created by running an instance of the Docker image. The container is a runnable instance of the image that includes a writable layer for the application to use.
- Isolating the container: Docker containers are isolated from the host operating system and other containers, providing a secure and consistent environment for the application to run.
- Managing the container: Docker provides a set of tools for managing containers, including starting, stopping, and restarting containers, monitoring container logs, and connecting containers to networks and storage.
- Sharing the container: Docker containers can be easily shared and distributed across different environments and platforms, providing a consistent way to run applications in development, testing, and production.
The below diagram is basically, a way to use Docker. And I am assuming that you have an idea about Docker Image and Dockerfile.
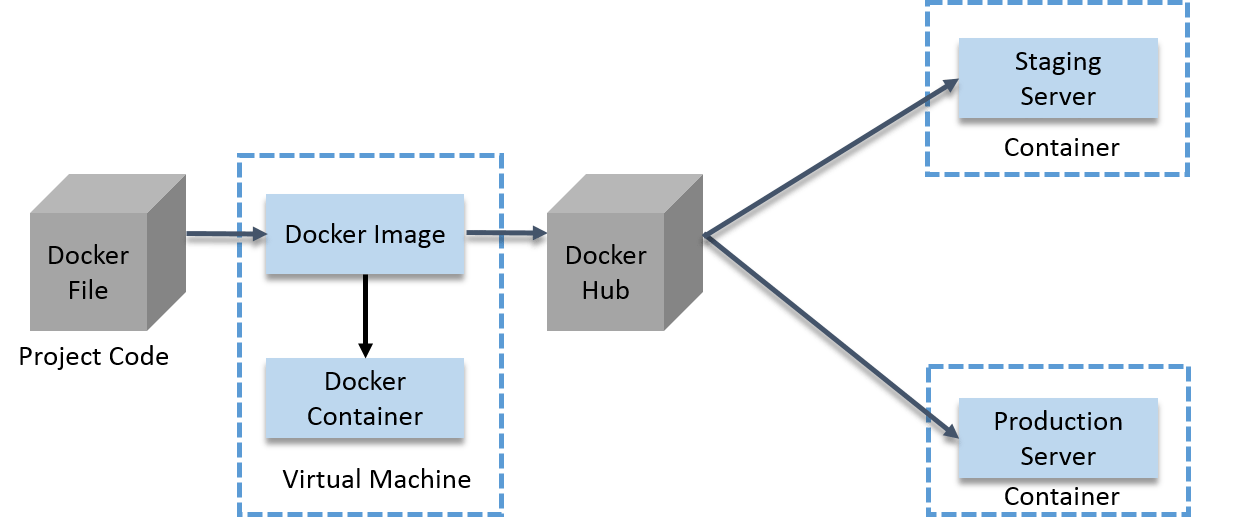
Below is the explanation of the diagram
- A developer will first write the project code in a Docker file and then build an image from that file.
- This image will contain the entire project code.
- Now, you can run this Docker Image to create as many containers as you want.
- This Docker Image can be uploaded on Docker hub (It is basically a cloud repository for your Docker Images, you can keep it public or private).
- This Docker Image on the Docker hub can be pulled by other teams such as QA or Prod.
This not only prevents the wastage of resources but also makes sure that the computing environment that is there in a Developer’s laptop is replicated in other teams as well. know how Docker solves the problem of microservices.
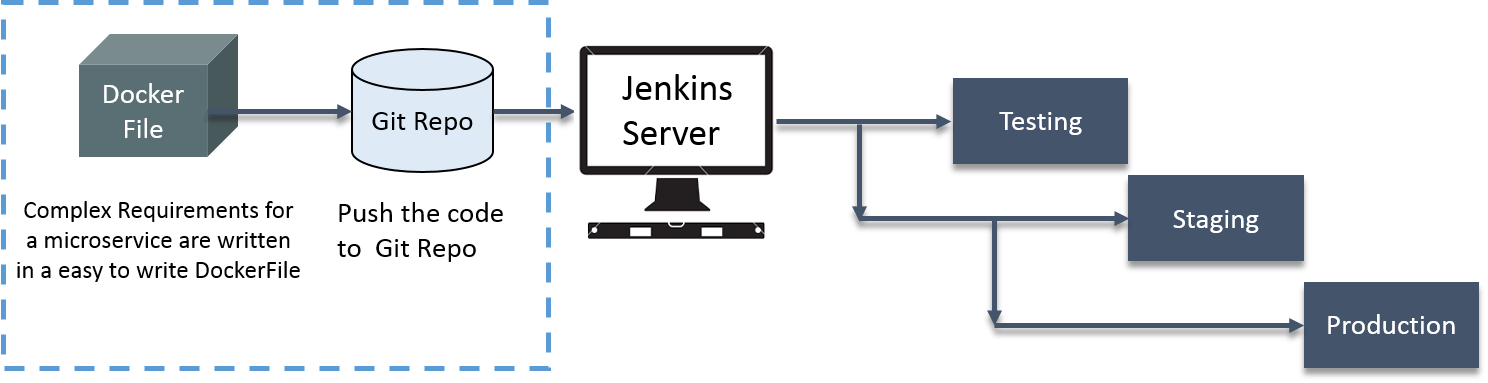
Below is the explanation of the diagram:
- Firstly, we wrote the complex requirements within a Dockerfile.
- Then, we pushed it on GitHub.
- After that, we used a CI server (Jenkins).
- This Jenkins server will pull it down from Git and then build the exact environment. This will be used in Production servers as well as in Test servers.
- We deployed it out to staging (It refers to deploying your software onto servers for testing purposes, prior to deploying them fully into production.) environments for Testers.
- Basically, we rolled exactly what we had in Development, Testing, and Staging into Production.
Learn the basics of Docker & know how we can use Docker Compose for multi-container applications.
Conclusion:
Docker containers provide a modern and efficient way to package, deploy, and manage applications, offering benefits such as consistency, portability, scalability, and resource efficiency. By using containerization technology, Docker containers create isolated environments for applications to run, providing a consistent and reliable way to deploy software across different environments and platforms. With the rise of cloud computing and microservices architectures, Docker containers have become an increasingly popular choice for modern software development and deployment.