What is Python Scikit-Learn?
Scikit-learn, also known as sklearn, is an open-source library for machine learning in Python. It is built on top of other popular Python libraries such as NumPy and SciPy, and provides a wide range of tools for tasks such as classification, regression, clustering, and dimensionality reduction. Scikit-learn is designed to be easy to use, with a consistent interface for working with different models and a wide range of functions for preprocessing and feature extraction. It is widely used in both academic and industry settings, and is well-documented and supported by an active community. The library is built on top of other Python libraries such as NumPy and SciPy, and provides a wide range of machine learning algorithms like linear and logistic regression, support vector machines, decision trees, random forests, gradient boosting, and many more.
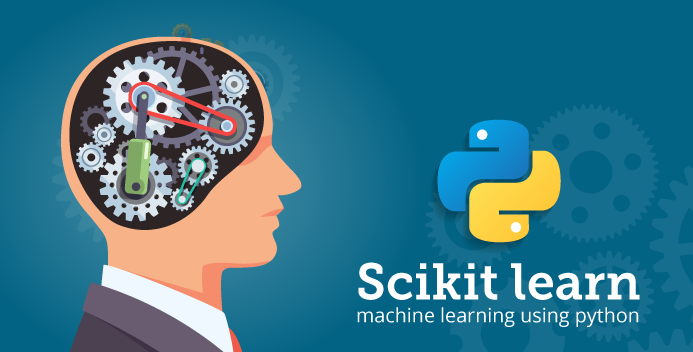
Scikit-learn is a popular machine learning library for Python. It is built on top of NumPy and SciPy, and provides a range of tools for tasks such as classification, regression, clustering, and dimensionality reduction.
One of the key advantages of scikit-learn is that it provides a consistent interface for working with different models, making it easy to switch between different algorithms and compare their performance. It also has a wide range of functions for preprocessing and feature extraction, which can be combined with models to create powerful workflows.
Here is an example of using scikit-learn to train a simple linear regression model:
from sklearn.linear_model import LinearRegression from sklearn.datasets import make_regression # Generate some synthetic data X, y = make_regression(n_samples=100, n_features=1, noise=20) # Create a linear regression object model = LinearRegression() # Fit the model to the data model.fit(X, y) # Make predictions y_pred = model.predict(X) # Print the model's coefficients print(model.co
This is just a basic example, scikit-learn offers many more models, preprocessing and feature extraction methods, metrics, evaluation methods and utilities.
You can find more information and examples in the scikit-learn documentation: https://scikit-learn.org/stable/
As you can see, scikit-learn is a powerful tool for machine learning in Python, and is widely used in both academic and industry settings. With its user-friendly interface and strong community support, it is an excellent choice for anyone looking to get started with machine learning in Python.
What is Machine learning?
Machine learning is a method of teaching computers to learn from data, without being explicitly programmed. It is a branch of artificial intelligence that involves building algorithms and models that enable computers to learn from data, and make predictions or decisions based on that data.
In machine learning, a model is trained on a dataset, which is a collection of examples. The model learns from these examples, and is then able to make predictions or decisions about new examples it has never seen before.
There are several types of machine learning, depending on the type of task and the structure of the data. They include:
- Supervised learning: the model is trained on labeled data, where the correct output is provided for each input. Examples include linear regression and support vector machines.
- Unsupervised learning: the model is trained on unlabeled data, and is used to discover hidden patterns or structure in the data. Examples include k-means and hierarchical clustering.
- Semi-supervised learning: the model is trained on a mix of labeled and unlabeled data, and is used to make predictions or decisions about new examples.
- Reinforcement learning: the model is trained through trial-and-error, and is used to make decisions in an environment.

Python Learn Polymorphism in Python with Examples is a popular programming language for machine learning, and it has several libraries and frameworks that can be used for machine learning tasks. Scikit-learn is one of the most popular libraries for machine learning in Python, but there are many others such as TensorFlow, Keras, PyTorch, and others.
Python’s simplicity, flexibility, and large community have made it one of the most popular languages for machine learning, with many tutorials, libraries and frameworks, and a large community of users.
In addition to linear regression, scikit-learn also provides a wide range of other models, including:
- Classification: logistic regression, k-nearest neighbors, decision trees, random forests, and support vector machines (SVMs)
- Clustering: k-means, hierarchical clustering, and DBSCAN
- Dimensionality reduction: principal component analysis (PCA) and t-SNE
- Model selection: cross-validation and hyperparameter tuning
- Ensemble methods: bagging, boosting, and stacking
Scikit-learn also provides many tools for working with different types of data, such as text and images. For example, it has functions for loading and manipulating image data, and for extracting features such as edges and textures.
In addition to its built-in functions, scikit-learn also integrates with other popular Python libraries for data science and machine learning, such as pandas for data manipulation and matplotlib for visualization. This makes it easy to use scikit-learn as part of a larger data science workflow.
Scikit-learn is a powerful and versatile library that makes it easy to apply machine learning techniques to real-world problems. With its wide range of models, preprocessing and feature extraction tools, and utilities for model selection and evaluation, it is an excellent choice for anyone looking to get started with machine learning in Python.
It’s worth mentioning that there are some other libraries you can use if you are looking for specific functionality such as deep learning, image processing, natural language processing etc. However, scikit-learn is a great starting point as a general-purpose library with a lot of functionality.
How to Install Scikit-Learn?
Scikit-learn can be easily installed using the Python package manager pip.
To install the latest version of scikit-learn, you can use the following command:
pip install -U scikit-learn
Make sure to use the pip command that is associated with the Python version you’re using.
You can also install scikit-learn and its dependencies using the package manager of your operating system, like apt-get for Ubuntu or conda for Anaconda.
conda install scikit-learn
Once the installation is complete, you can check if scikit-learn is installed by importing it in a Python script:
import sklearn
print(sklearn.__version__)
It’s also worth noting that scikit-learn requires a few dependencies to work properly such as NumPy, SciPy and joblib. These libraries should be automatically installed along with scikit-learn, but in case you encounter any issues, you can install them manually using pip or your operating system package manager.
It’s always a good idea to use a virtual environment when installing python packages, so that you have a clean environment and you can always have the right version of the packages you need.
In addition to the core functionality provided by scikit-learn, there are also many additional tools and libraries that can be used in conjunction with it to extend its capabilities.
One popular library that is often used with scikit-learn is XGBoost, which provides an efficient implementation of gradient boosting for decision trees. This can be used to improve the performance of scikit-learn’s existing gradient boosting implementation, and is particularly useful for large and complex datasets.
Another library that is often used with scikit-learn is Keras, which is a high-level neural network library written in Python. It can be used to train deep learning models, and can be run on top of TensorFlow, CNTK, or Theano. This allows you to use scikit-learn’s preprocessing and feature extraction tools, and then use Keras to train a neural network for the final prediction.
Additionally, you can use scikit-learn with libraries such as pandas for data manipulation, and matplotlib for visualization. These libraries are widely used in the data science community and can make it easy to work with different types of data, and to create detailed visualizations of results.
Scikit-learn is also well supported by a large and active community, which means that there are many resources available for learning and troubleshooting. The documentation and tutorials provided by the scikit-learn community are extensive and cover a wide range of topics. There are also many books, online courses, and tutorials available that cover machine learning with scikit-learn in more detail.
In summary, scikit-learn is a powerful and versatile library that makes it easy to apply machine learning techniques to real-world problems. With its wide range of models, preprocessing and feature extraction tools, and utilities for model selection and evaluation, it is an excellent choice for anyone looking to get started with machine learning in Python. Additionally, it can be easily integrated with other libraries and tools to extend its capabilities and make it even more powerful.
What Can we achieve using Python Scikit-Learn?
Scikit-learn is a versatile library that can be used to perform a wide range of machine learning tasks, including:
- Classification: predict the class of an instance based on its features. Examples include logistic regression, k-nearest neighbors, decision trees, and random forests.
- Regression: predict a continuous value based on the input features. Examples include linear regression and support vector regression.
- Clustering: group similar instances together based on their features. Examples include k-means and hierarchical clustering.
- Dimensionality reduction: reduce the number of features in a dataset while preserving as much information as possible. Examples include principal component analysis (PCA) and t-SNE.
- Model selection: choose the best model for a given dataset and task by comparing the performance of different models using techniques such as cross-validation.
- Ensemble methods: combining multiple models to improve performance. Examples include bagging, boosting, and stacking.
Scikit-learn also provides many tools for working with different types of data, such as text and images. For example, it has functions for loading and manipulating image data, and for extracting features such as edges and textures.
Scikit-learn makes it easy to evaluate the performance of a model by providing various evaluation metrics and utilities. This helps to fine-tune the model and optimize its performance.
Scikit-learn is also well-integrated with other popular libraries such as pandas, NumPy, and Matplotlib, which allows you to use it as part of a larger data science workflow.
Overall, scikit-learn is a powerful and versatile library that makes it easy to apply machine learning techniques to real-world problems. With its wide range of models, preprocessing and feature extraction tools, and utilities for model selection and evaluation, it is an excellent choice for anyone looking to get started with machine learning in Python.