It is challenging for freshers to find most asked python programming coding interview questions that commonly asked in interviews.
Here We have collected 35+ Most asked python coding Interview Questions that are recommended by Engineers of MNCs.
Python Coding Questions on Numbers
1. Write a program to reverse an integer in Python.
Here’s one way to reverse an integer in Python:
n = int(input("Please enter a number: ")) print("Before reverse:", n) reverse = 0 while n != 0: reverse = reverse * 10 + n % 10 n = n // 10 print("After reverse:", reverse
This code takes an input from the user and stores it in the variable n. Then, it prints the original number n. After that, it initializes a variable reverse to 0 and uses a while loop to continuously add the last digit of n to reverse while also updating n to be the integer division of n by 10, until n becomes zero. Finally, it prints the reversed number.
Output:
Please enter a number: 5642 Before reverse: 5642 After reverse: 2456
2. Write a program in Python to check whether an integer is Armstrong number or not.
What is Armstrong Number?
- It is a number which is equal to the sum of cube of its digits.
- For eg: 153, 370 etc.
Lets see it practically by calculating it,
Suppose I am taking 153 for an example
First calculate the cube of its each digits
1^3 = (1*1*1) = 1
5^3 = (5*5*5) = 125
3^3= (3*3*3) = 27
Now add the cube
1+125+27 = 153
It means 153 is an Armstrong number.
Program to check a number is Armstrong or not in python programming language:
i = 0 result = 0 n = int(input("Please enter a number: ")) number1 = n temp = n while n != 0: n = n // 10 i += 1 while number1 != 0: n = number1 % 10 result += pow(n, i) number1 = number1 // 10 if temp == result: print("The number is an Armstrong number") else: print("The number is not an Armstrong number
This code takes an input from the user and stores it in the variable n. Then, it initializes two variables i and result to 0 and creates a copy of n in the variable number1 and temp.
The first while loop calculates the number of digits in n by repeatedly dividing n by 10 until it becomes zero, and increments i by 1 for each iteration.
The second while loop calculates the sum of each digit of n raised to the power of i. It does this by first finding the last digit of number1 using the modulus operator and adding the result of raising it to the power of i to result. Then, it updates number1 to be the integer division of number1 by 10, until number1 becomes zero.
Finally, the code checks if temp is equal to result. If they are equal, it prints “The number is an Armstrong number”. Otherwise, it prints “The number is not an Armstrong number”.
If you entered 245 as the input, the code would produce the following output:
Please enter a number: 245 The number is not an Armstrong number
The number 245 is not an Armstrong number because 2^3 + 4^3 + 5^3 ≠ 245.
3. Write a program in Python to check given number is prime or not.
What is prime number?
In Mathematical term, A prime number is a number which can be divided by only 1 and number itself.
For example : 2, 3, 5, 7, 13,…
Here 2, 3 or any of the above number can only be divided by 1 or number itself.
How our program will behave?
Suppose if someone gives an input 2 then our program should give output “given number is a prime number”.
And if someone gives 4 as an input then our program should give output “given number is not a prime number”.
Python program to check given number is prime or not:
temp = 0 n = int(input("Please enter a number: ")) for i in range(2, n//2): if n % i == 0: temp = 1 break if temp == 1: print("The given number is not a prime number") else: print("The given number is a prime number"
Output:
Please enter a number: 7 The given number is a prime number
The number 7 is a prime number because it is only divisible by 1 and itself, so the for loop wouldn’t find any numbers in the range 2 to 7//2 = 3 that divide 7 evenly.
4.Write a program in Python to print the Fibonacci series using iterative method.
What is Fibonacci Series?
A Fibonacci series is a series in which next number is a sum of previous two numbers.
For example : 0, 1, 1, 2, 3, 5, 8 ……
In Fibonacci Series, first number starts with 0 and second is with 1 and then its grow like,
0
1
0 + 1 = 1
1 + 1 = 2
1 + 2 = 3
2 + 3 = 5 and
so on…
How our program will behave?
Suppose if someone gives an input 5 then our program should print first 5 numbers of the series.
Like if someone given 5 as a input then our Fibonacci series which is written in C should print output as,
0, 1, 1, 2, 3
Python program to print Fibonacci series program in using Iterative methods:
first, second = 0, 1 n = int(input("Please enter a number to generate the Fibonacci series: ")) print("The Fibonacci series is:") for i in range(0, n): if i <= 1: result = i else: result = first + second first = second second = result print(result
Output:
If you entered 7
as the input, the code would produce the following output:
Please enter a number to generate the Fibonacci series: 7 The Fibonacci series is: 0 1 1 2 3 5 8
The code generates the Fibonacci series up to the 7th term, which are the first 7 numbers in the Fibonacci sequence: 0, 1, 1, 2, 3, 5, and 8.
5. Write a program in Python to print the Fibonacci series using recursive method.
Python program to print Fibonacci series using recursive methods:
def fibonacci(num): if num == 0: return 0 elif num == 1: return 1 else: return fibonacci(num-1) + fibonacci(num-2) n = int(input("Please enter a number to generate the Fibonacci series: ")) print("The Fibonacci series is:") for i in range(0, n): print(fibonacci(i))
Output:
If you entered 5
as the input, the code would produce the following output:
Please enter a number to generate the Fibonacci series: 5 The Fibonacci series is: 0 1 1 2 3
The code generates the Fibonacci series up to the 5th term, which are the first 5 numbers in the Fibonacci sequence: 0, 1, 1, 2, and 3.
6. Write a program in Python to check whether a number is palindrome or not using iterative method.
What is Palindrome Number?
A Palindrome number is a number which reverse is equal to the original number means number itself.
For example : 121, 111, 1223221, etc.
In the above example you can see that 121 is a palindrome number. Because reverse of the 121 is same as 121.
How our program will behave?
Suppose if someone gives an input 121 then our program should print “the given number is a palindrome”.
And if someone given input 123 the our program should print “the given number is not a palindrome number”.
Python program for palindrome number using iterative method
n = int(input("Please enter a number: ")) temp = n # reverse the number reverse = 0 while temp != 0: reverse = reverse * 10 + temp % 10 temp = temp // 10 # check if the number is a palindrome if reverse == n: print("The number is a palindrome.") else: print("The number is not a palindrome.")
Output:
If you entered 121 as the input, the code would produce the following output:
Please enter a number: 121 The number is a palindrome.
The code first stores the entered number in the temp variable and initializes reverse to 0. Then, it uses a while loop to reverse the number. If the reversed number is equal to the original number, then the code prints “The number is a palindrome”. If the reversed number is not equal to the original number, then the code prints “The number is not a palindrome”.
7. Write a program in Python to check whether a number is palindrome or not using recursive method.
Python program for palindrome number using recursive method
def reverse(num): if num < 10: return num else: return int(str(num % 10) + str(reverse(num // 10))) def is_palindrome(num): if num == reverse(num): return True else: return False n = int(input("Please enter a number: ")) if is_palindrome(n): print("The number is a palindrome.") else: print("The number is not a palindrome.
Output: If you enter 535
as the input, the output will be:
Please enter a number: 535 The number is a palindrome.
8. Write a program in Python to find greatest among three integers.
Suppose if someone give 3 numbers as input 12, 15 and 10 then our program should return 15 as a output because 15 is a greatest among three.
Python program to find greatest of three numbers
n1 = int(input("Please enter the first number (n1): ")) n2 = int(input("Please enter the second number (n2): ")) n3 = int(input("Please enter the third number (n3): ")) if n1 >= n2 and n1 >= n3: print("n1 is the greatest") elif n2 >= n1 and n2 >= n3: print("n2 is the greatest") else: print("n3 is the greatest")
Output:
If you enter 20
, 30
, and 10
as the inputs for n1
, n2
, and n3
respectively, the output will be:
Please enter the first number: 20 Please enter the second number: 30 Please enter the third number: 10 n2 is the greatest
9. Write a program in Python to check if a number is binary?
In the below program if someone give any input in 0 and 1 format then our program will run and give output as given number is in binary format.
And if someone give another number different from 0 and 1 like 2, 3 or any other then our program will give output as given number is not in a binary format.
Program to check given number representation is in binary or not:
def is_binary(num): while num > 0: digit = num % 10 if digit not in [0, 1]: return False num = num // 10 return True num = int(input("Please enter a number: ")) if is_binary(num): print("The number is binary.") else: print("The number is not binary.")
Output:
Please enter a number:12345 num is not binary
10. Write a program in Python to find sum of digits of a number using recursion?
def sum_of_digits(num): if num<10: return num else: return (num%10) + sum_of_digits(num//10) number = int(input("Enter a number: ")) print("Sum of digits of the number is: ", sum_of_digits(number))
Output:
If you enter 10 as the input, the output would be:
Enter a number: 10 Sum of digits of the number is: 1
11. Write a program in Python to swap two numbers without using third variable?
The swapping program without using third variable we will assign two different value to the different variables.
Python program to swap two number without using third variable
a = int(input("please give first number a: ")) b = int(input("please give second number b: ")) a=a-b b=a+b a=b-a print("After swapping") print("value of a is : ", a); print("value of b is : ", b);
Output:
If the input is a=5 and b=7,
After swapping value of a is : 7 value of b is : 5
12. Write a program in Python to swap two numbers using third variable?
Swapping of two numbers using third variable in Python language
a = int(input("please give first number a: ")) b = int(input("please give second number b: ")) tempvar=a a=b b=tempvar print("After swapping") print("value of a is : ", a); print("value of b is : ", b);
Output:
If the input is “10”, “20”, the output will be:
After swapping value of a is : 20 value of b is : 10
13. Write a program in Python to find prime factors of a given integer.
Finding prime factors of a given integer:
def prime_factors(num): i = 2 factors = [] while i * i <= num: if num % i: i += 1 else: num //= i factors.append(i) if num > 1: factors.append(num) return factors num = int(input("Enter a number: ")) result = prime_factors(num) print("The prime factors of", num, "are: ", result)
Output:
Enter a number: 20 The prime factors of 20 are: [2, 2, 5].
14. Write a program in Python to add two integer without using arithmetic operator?
This program will take two number as a Input. And After performing some operation as per program, it will return addition.
Suppose if we give input 35 and 20. Our program will return 55 as an output without using of an addition operator.
Below is a program to add two numbers without using + operator in Java
import java.util.Scanner; public class Main { public static void main(String[] arg) { int x, y; Scanner sc = new Scanner(System.in); System.out.print("Please give first number: "); x = sc.nextInt(); System.out.print("Please give second number: "); y = sc.nextInt(); while (y != 0) { int temp = x & y; x = x ^ y; y = temp << 1; } System.out.println("Sum = " + x); }
Output:
Please give first number: 20 Please give second number: 10 Sum = 30;
15. Write a program in Python to find given number is perfect or not?
What is Perfect Number?
A perfect number is a positive integer that is equal to the sum of its positive divisors, excluding the number itself.
Lets understand it with example
6 is a positive number and its divisor is 1,2,3 and 6 itself.
But we should not include 6 as by the definition of perfect number.
Lets add its divisor excluding itself
1+2+3 = 6 which is equal to number itself.
It means 6 is a Perfect Number.
Below is a program to check a given number is perfect or not in Python
num = int(input("please give first number a: ")) sum=0 for i in range(1,(num//2)+1): remainder = num % i if remainder == 0: sum = sum + i if sum == num: print("given no. is perfect number") else: print("given no. is not a perfect number")
Output:
please give first number a: 6 given no. is perfect number please give first number a: 8 given no. is not a perfect number
16. Python Program to find the Average of numbers with explanations
The average of a set of numbers is simply the sum of the numbers divided by the total count of numbers.
Here’s an example of a Python program to calculate the average of a set of numbers:
size=int(input("Enter the number of elements you want in array: ")) arr=[] #taking input of the list for i in range(0,size): elem=int(input("Please give value for index "+str(i)+": ")) arr.append(elem) #taking average of the elements of the list avg=sum(arr)/size print("Average of the array elements is ",avg)
Explanations:
- Define a function
average
that takes a list of numbers as input. - Initialize two variables
total
andcount
to store the sum of numbers and the number of items respectively. - Loop through the list of numbers and add each number to the
total
and incrementcount
by 1. - Return the result of dividing
total
bycount
which is the average. - Call the function with a list of numbers, and store the result in a variable
result
. - Print the result using the print statement.
Output:
Enter the number of elements you want in array: 3 Please give value for index 0:10 Please give value for index 1:20 Please give value for index 2:30 Average of the array elements is 20.0
17. Python Program to calculate factorial using iterative method.
A factorial of a number n is the product of all positive integers less than or equal to n. It is denoted as n!. For example, the factorial of 5 is 5! = 5 x 4 x 3 x 2 x 1 = 120.
In this article, we will write a Python program to calculate the factorial of a given number using an iterative method.
Here’s the code to calculate the factorial of a number:
def factorial(n): fact = 1for i in range(1, n+1): fact = fact * i return fact num = int(input("Enter a number: ")) result = factorial(num) print("The factorial of", num, "is", result)
Output:
Enter a number: 5 The factorial of 5 is 120
Let’s break down the code to understand the implementation.
- We have defined a function
factorial(n)
that takes an integer n as an argument. - Inside the function, we have initialized a variable
fact
to 1, which will store the factorial of the number. - Using a
for
loop, we are iterating over the range from 1 ton+1
, and at each iteration, we are multiplyingfact
with the current value ofi
. - The
fact
variable will keep updating its value at each iteration untili
becomes equal ton+1
. - Finally, the function returns the
fact
variable, which is the factorial of the numbern
. - After defining the function, we are taking an input of a number from the user, and calling the
factorial
function by passing the input number as an argument. - The returned result is then printed on the screen.
This is how the iterative method can be used to calculate the factorial of a number in Python.
18. Python Program to calculate factorial using recursion.
Python Program to find factorial of a number using recursion:
def factorial(n): if n == 0: return 1 else: return n * factorial(n-1) num = int(input("Enter a number: ")) print("The factorial of", num, "is", factorial(num))
Output:
Enter the number: 6 The factorial of 6 is 720
19.Python Program to check a given number is even or odd.
A program to check whether a given number is even or odd can be written in Python using simple conditional statements.
Here is the code:
def even_odd(num): if num % 2 == 0: return "The number is Even" else: return "The number is Odd" # Taking input from the user num = int(input("Enter a number: ")) print(even_odd(num))
Output:
Enter a number: 5 The number is Odd
Explanation:
- In the first line, we have defined a function named
even_odd
that takes a single argumentnum
. - Inside the function, we use an if-else statement to check if the remainder of the division of
num
by 2 is equal to 0. - If the remainder is equal to 0, the number is even, and the function returns “The number is Even”.
- If the remainder is not equal to 0, the number is odd, and the function returns “The number is Odd”.
- After defining the function, we take input from the user and store it in the variable
num
. - Finally, we call the function
even_odd
and pass the value ofnum
as an argument. The result is printed using the print statement.
20. Python program to print first n Prime Number with explanation.
The following program demonstrates how to print first n Prime Numbers using a for loop and a while loop in Python:
def is_prime(num): if num > 1: for i in range(2, num): if (num % i) == 0: return Falseelse: return Trueelse: return Falsedef print_first_n_prime(n): prime_list = [] count = 0 num = 2while count < n: if is_prime(num): prime_list.append(num) count += 1 num += 1return prime_list n = int(input("Enter the value of n: ")) print("First", n, "prime numbers are:", print_first_n_prime(n))
Output:
Enter the value of n: 5 First 5 prime numbers are: [2, 3, 5, 7, 11]
In this program, we have defined two functions – is_prime and print_first_n_prime. The is_prime function takes a number as an argument and returns True if the number is prime, otherwise False. The print_first_n_prime function takes the number of prime numbers we want to print and returns a list of those prime numbers.
In the main program, we first ask the user for the value of n, which is the number of prime numbers we want to print. Then, we call the print_first_n_prime function, which returns a list of prime numbers, and finally, we print that list.
21. Python Program to print Prime Number in a given range.
The following program demonstrates how to print Prime Number in a given range in Python:
def is_prime(n): if n < 2: return False for i in range(2, int(n**0.5) + 1): if n % i == 0: return False return True def prime_in_range(lower, upper): primes = [] for num in range(lower, upper + 1): if is_prime(num): primes.append(num) return primes lower = int(input("Enter the lower range: ")) upper = int(input("Enter the upper range: ")) result = prime_in_range(lower, upper) if result == []: print("No prime number found in the given range.") else: print("Prime numbers in the given range:", resu
Explanation:
- The program first defines a helper function
is_prime
to check if a number is prime or not. is_prime
function checks if the number is less than 2, in that case it returns False as 1 and 0 are not prime numbers.- If the number is greater than or equal to 2, it checks the number by dividing it with all numbers between 2 and the square root of the number. If the number is divisible by any of these numbers, it returns False, meaning the number is not prime.
- The function
prime_in_range
takes two inputslower
andupper
which are the lower and upper limits of the range of numbers we want to find prime numbers in. - The function loops through all numbers from
lower
toupper
and calls theis_prime
function to check if the number is prime or not. If the number is prime, it appends it to the listprimes
. - At the end, the function returns the list of prime numbers.
- Finally, the program takes the lower and upper limits as inputs from the user and calls the
prime_in_range
function to get the list of prime numbers in the given range. If the list is empty, it prints “No prime number found in the given range.”, otherwise, it prints the list of prime numbers.
22. Python Program to find Smallest number among three.
Here is a simple Python program to find the smallest number among three:
def find_smallest(num1, num2, num3): smallest = num1 if num2 < smallest: smallest = num2 if num3 < smallest: smallest = num3 return smallest num1 = int(input("Enter the first number: ")) num2 = int(input("Enter the second number: ")) num3 = int(input("Enter the third number: ")) smallest = find_smallest(num1, num2, num3) print("The smallest number is", smalles
Example Output:
Enter the first number: 25 Enter the second number: 12 Enter the third number: 36 The smallest number is 12
Explanation:
- The function
find_smallest()
takes three numbers as arguments and returns the smallest number among them. - Inside the function,
smallest
is set tonum1
initially. - The next two
if
statements comparenum2
andnum3
withsmallest
and updates the value ofsmallest
if eithernum2
ornum3
is smaller. - Finally, the smallest number is returned.
- In the main part of the code, the three numbers are taken as input from the user and stored in the variables
num1
,num2
, andnum3
. - The smallest number is found using the
find_smallest()
function and stored in the variablesmallest
. - The value of
smallest
is then printed.
23. Python program to calculate the power using the POW method.
The pow method in Python can be used to calculate the power of a number. The function pow(base, exponent) returns the base raised to the power of exponent. Here is an example of how to use the pow method in Python to calculate the power:
# input the base and exponent base = int(input("Enter the base number: ")) exponent = int(input("Enter the exponent: ")) # calculate the power using the pow method result = pow(base, exponent) # print the result print("The result of {} raised to the power of {} is {}".format(base, exponent, result)
In this example, we take the base and exponent as input from the user and then use the pow
method to calculate the power of the base raised to the exponent. Finally, we print the result.
Enter the base number: 2 Enter the exponent: 3 The result of 2 raised to the power of 3 is 8
24. Python Program to calculate the power without using POW function.(using for loop).
Python code to calculate the power using ‘for-loop’
#taking 3 integer as input from the user base = int(input("Enter the value for base :")) exponent = int(input("Enter the value for exponent :")) result=1; print("The result of ",base,"raised to the power of ",exponent," is ",end = ' ') #using ‘for’ loop with ‘range’ function for exponent in range(exponent, 0, -1): result *= base print(result)
Output:
Enter the value for base : 5 Enter the value for exponent : 4 The result of 5 raised to the power of 4 is 625
Explanation:
For the input from the user, the base number is 5, and the exponent number is 4. The ‘base exponent’ will be 54, which is 5x5x5x5 i.e. 625.
25. Python Program to calculate the power without using POW function.(using while loop).
Python Code to calculate the power
base = int(input("Enter the value for base :")) exponent = int(input("Enter the value for exponent :")) result=1; print(base,"to power ",exponent,"=",end = ' ') #using while loop with a condition that come out of while loop if exponent is 0 while exponent != 0: result = base * result exponent-=1 print(result)
Output:
Enter the value for base : 5 Enter the value for exponent : 4 5 to power of 4 = 625
Explanation:
For the input from the user, the base number is 5, and the exponent number is 4. The ‘base e exponent’ will be 54, which is 5x5x5x5 i.e. 625.
26. Python Program to calculate the square of a given number.
Python Program to calculate the square of a given number
#take input from the user num = int(input("Enter a number to calculate square : ")) print("square =",num*num)
Output:

Explanation:
For input 7, the output generated by our program is 7*7 i.e. equals 49.
27. Python Program to calculate the cube of a given number.
Python Program to calculate the cube of a given number
#take input from the user num = int(input("Enter a number to calculate cube : ")) print("square =",num*num*num)
Output:

Explanation:
For input 7, the output generated by our program is 5*5*5 i.e. equals 125.
28. Python Program to calculate the square root of a given number.
Python program to calculate the square root of a given number
import math #take input from the user num = int(input("Enter a number to find square root : ")) #check if the input number is negative if num<0: print("Negative numbers can't have square roots") else: print("square roots = ",math.sqrt(num))
Output 1:

Explanation:
For input 81, the output generated by our program is math.sqrt(81) which is equal to 9.0.
29. Python program to calculate LCM of given two numbers.
Python code to find l.c.m. of two numbers
num1 = int(input("Enter first number: ")) num2 = int(input("Enter second number: ")) if num1 > num2: greater = num1 else: greater = num2 while(True): if((greater % num1 == 0) and (greater % num2 == 0)): lcm = greater break greater += 1 print("LCM of",num1,"and",num2,"=",greater)
Output 1:
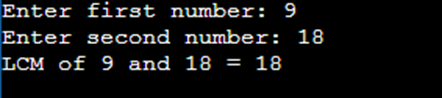
Explanation:
For inputs 9 and 18, we are dividing the ‘greater’ with both num1 and num2 to find the common multiple of 9 and 18. First greater assigned with value 18 and then in while loop it checking continuously that if ‘18/9 == 0 and 18/18 == 0’ which is true so the value of greater is returned as the L.C.M. of 9 and 18.
30. Python Program to find GCD or HCF of two numbers.
Python code to find h.c.f. of two numbers
#taking two inputs from the user num1 = int(input("Enter first number: ")) num2 = int(input("Enter second number: ")) #checking for smaller number if num1 > num2: minimum = num2 else: minimum = num1 #finding highest factor of the numbers for i in range(1, minimum+1): if((num1 % i == 0) and (num2 % i == 0)): hcf = i print("hcf/gcd of",num1,"and",num2,"=",hcf)
Output :
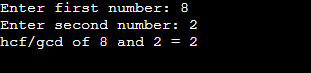
Explanation:
For inputs 8 and 2, the program is finding the highest factor that is common in both 8 and 2.
The factor of 8 is 1,2,4,8 and the factor of 2 is 1,2. The highest factor common in both is 2.
31. Python Program to find GCD of two numbers using recursion
Python code to find GCD of two numbers using recursion
def gcd(num1,num2): if num2 == 0: return num1; return gcd(num2, num1 % num2) #taking inputs from the user num1 = int(input("Enter first number: ")) num2 = int(input("Enter second number: ")) print("hcf/gcd of",num1,"and",num2,"=",gcd(num1,num2))
Output :
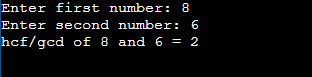
Explanation:
For the inputs 8 and 6, which are then passed as an argument in recursive function gcd().
The recursive function behaves in such way:-
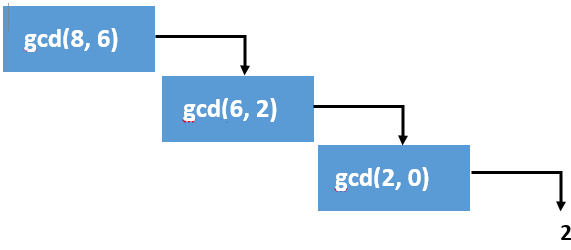
32. Python Program to Convert Decimal Number into Binary.
Problem statement
Given a number we need to convert into a binary number.
Approach 1 − Recursive Solution
DecToBin(num): if num > 1: DecToBin(num // 2) print num % 2
Example
def DecimalToBinary(num):if num > 1:DecimalToBinary(num // 2)print(num % 2, end = '')# mainif __name__ == '__main__': dec_val = 35DecimalToBinary(dec_val)
Output
100011
Approach 2 − Built-in Solution
Example
def decimalToBinary(n):return bin(n).replace("0b", "")# Driver codeif __name__ == '__main__':print(decimalToBinary(35))
Output
100011
33.Python Program to convert Decimal number to Octal number.
Decimal to Octal in Python Using Loop:
The common method of calculating the octal representation of a decimal number is to divide the number by 8 until it becomes 0. After the division is over, we print the array storing the remainders at each division point in reverse order.
def DecimalToOctal(n): # array to store octal number octal = [0] * 100 # counter for octal digits i = 0 # run until n is 0 while (n != 0): # Storing Remainder in Octal Array octal[i] = n % 8 # updating value of n to n/8 n = int(n / 8) # Increasing the Counter i += 1 # Traversing Octal Array in Reverse Order for j in range(i - 1, -1, -1): print(octal[j], end=""
Output:
41
34. Python Program to check the given year is a leap year or not.
# Default function to implement conditions to check leap year def CheckLeap(Year): # Checking if the given year is leap year if((Year % 400 == 0) or (Year % 100 != 0) and (Year % 4 == 0)): print("Given Year is a leap Year"); # Else it is not a leap year else: print ("Given Year is not a leap Year") # Taking an input year from user Year = int(input("Enter the number: ")) # Printing result CheckLeap(Year)
Output:
Enter the number: 1700 Given year is not a leap Year
Explanation:
We have implemented all the three mandatory conditions (that we listed above) in the program by using the ‘and’ and ‘or’ keyword inside the if else condition. After getting input from the user, the program first will go to the input part and check if the given year is a leap year. If the condition satisfies, the program will print ‘Leap Year’; else program will print ‘Not a leap year.’
35. Python Program to convert Celsius to Fahrenheit.
celsius_1 = float(input("Temperature value in degree Celsius: " )) # For Converting the temperature to degree Fahrenheit by using the above # given formula Fahrenheit_1 = (celsius_1 * 1.8) + 32 # print the result print('The %.2f degree Celsius is equal to: %.2f Fahrenheit' %(celsius_1, Fahrenheit_1)) print("----OR----") celsius_2 = float (input("Temperature value in degree Celsius: " )) Fahrenheit_2 = (celsius_2 * 9/5) + 32 # print the result print ('The %.2f degree Celsius is equal to: %.2f Fahrenheit' %(celsius_2, Fahrenheit_2))
Output:
Temperature value in degree Celsius: 34 The 34.00 degree Celsius is equal to: 93.20 Fahrenheit ----OR---- Temperature value in degree Celsius: 23 The 23.00 degree Celsius is equal to: 73.40 Fahrenheit
36. Python Program to convert Fahrenheit to Celsius.
Python code to convert fahrenheit into celsius
#taking input from the user fahrenheit = float(input("Please give the Hahrenheit Temperature : ")) #converting Celsius into Fahrenheit celsius = ((fahrenheit-32)*5)/9 print("Celcius= ",celsius)
Output 1:

Explanation:
For the input Fahrenheit temperature 96.8.
Celsius temperature = ((96.8 -32)*5)/9 = 36.0.
37. Python program to calculate Simple Interest with explanation.
Python code to calculate simple interest
#taking the values of principal, rate of interest and time from the user principal = int(input("Enter the principal amount: ")) rate = int(input("Enter the rate of interest: ")) time = int(input("Enter the time of interest in year: ")) #using the input values calculate simple interest simpleInterest = (principal*rate*time)/100 print("Simple Interest = ",simpleInterest)
Output :
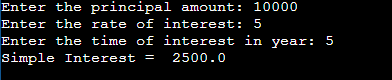
Explanation:
For the input value of the principal, the rate of interest and the time simple interest is calculated using the formula
Simple interest = (10000 * 5 *5)/100
Simple interest = 2500.0
Python Coding Questions on String
1. Python program to remove given character from String.
Remove character from string using replace() method in python
def remove_char(s1,s2): print(s1.replace(s2, '')) s1 = input("please give a String : ") s2 = input("please give a Character to remove : ") remove_char(s1,s2)
Output:

Remove character from string using translate() method in python
def remove_char(s1,s2): dict = {ord(s2) : None} print(s1.translate(dict)) s1 = input("please give a String : ") s2 = input("please give a Character to remove : ") remove_char(s1,s2)
Output:

2. Python Program to count occurrence of a given characters in string.
Python Program to count occurrence of given character in string.
Using for Loop
string = input("Please enter String : ") char = input("Please enter a Character : ") count = 0 for i in range(len(string)): if(string[i] == char): count = count + 1 print("Total Number of occurence of ", char, "is :" , count)
Output:

Using while Loop
string = input("Please enter String : ") char = input("Please enter a Character : ") index, count = 0, 0 while(index < len(string)): if(string[index] == char): count = count + 1 index = index + 1 print("Total Number of occurence of ", char, "is :" , count)
Output:

Using Method
def countOccur(char, string): count = 0 for i in range(len(string)): if(string[i] == char): count = count + 1 return count string = input("Please enter String : ") char = input("Please enter a Character : ") count = countOccur(char, string) print("Total Number of occurence of ", char, "is :" , count)
Output:

3. Python Program to check if two Strings are Anagram.
Anagrams program in Python:
def anagramCheck(str1, str2): if (sorted(str1) == sorted(str2)) : return True else : return False str1 = input("Please enter String 1 : ") str2 = input("Please enter String 2 : ") if anagramCheck(str1,str2): print("Anagram") else: print("Not an anagram")
Output:
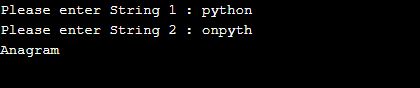
4. Python program to check a String is palindrome or not.
Palindrome program of String in Python
Here we have declared one method isPalindrome(string).
def isPalindrome(string): for i in range(0, int(len(string)/2)): if string[i] != string[len(string)-i-1]: return False return True string = input("Please give a String : ") if (isPalindrome(string)): print("Given String is a Palindrome") else: print("Given String is not a Palindrome")
Output:

5.Python program to check given character is vowel or consonant.
Python code to check given character is vowel or consonant
Ch=input(“Enter a character to check vowel or consonant :”) if(ch == 'a' or ch == 'e' or ch == 'i' or ch == 'o' or ch == 'u' or ch == 'A' or ch == 'E' or ch == 'I' or ch == 'O' or ch == 'U'): #if ‘if’ condition satisfy print(“Given character”, ch ,”is vowel”) else: #if ‘if’ condition does not satisfy the condition print(“Given character”,ch, “is consonant”)
Output :

Explanation:
As we can see for the character ‘o’ output generated is “Vowel”, which is obvious as o is a vowel and satisfying the ‘if ’ statement
6. Python program to check given character is digit or not.
Python code to check given character is digit or not
#taking input from user ch = input("Enter a character : ") if ch >= '0' and ch <= '9': #comparing the value of ‘ch’ # if the condition holds true then this block will execute print("Given Character ", ch, "is a Digit") else: #this block will execute if the condition will not satisfies print("Given Character ", ch, "is not a Digit")
Output :

Explanation:
Here , the output generated is “ Given Character 8 is a Digit” for input 8 , as the 8>=’0’ and 8<=’9’ , so both condition satisfy well hence the if block of our program is executed.
7.Python program to check given character is digit or not using isdigit() method.
Python code to check given character is digit or not using ‘isdigit()’ function:
# taking input from user ch = input("Enter a character : ") # checks if character ‘ch’ is digit or not if(ch.isdigit()): #if ‘ch.digit()’ returns “True” print("The Given Character ", ch, "is a Digit") else: #if ‘ch.digit()’ returns “False” print("The Given Character ", ch, "is not a Digit")
Output :

Explanation :
In this case, the user has given 5 as an inputs, and the code generate the output as “The Given Character 5 is a Digit”. This shows the 5 is a numerical value, which is true. In the ‘condition’ block of our program, ch.isdigit() returned true for character ‘5’. So the ‘if’ block executes and the desired output is generated.
8. Python program to replace the string space with a given character.
Here’s one way to replace spaces with a given character in a string in Python:
def replace_space(string, char): return string.replace(" ", char) input_string = "end of the day" replacement_char = "-" output_string = replace_space(input_string, replacement_char) print(output_string
Output:
end-of-the-day
Explanation :
The code replaces spaces in a given string with a specified character. It defines a function replace_space that takes a string string and a character char as input and returns a new string with spaces replaced by the char input.
The string.replace() method is used to replace occurrences of a specified string in the input string with another string. In this case, we replace each occurrence of the space character ” ” with the char input.
The input string “end of the day” and the replacement character “-” are defined and passed as arguments to the replace_space function. The output string is stored in the output_string variable and printed.
9. Python program to replace the string space with a given character using replace() method.
Here’s one way to replace spaces with a given character in a string using the replace()
method in Python:
def replace_space(string, char): return string.replace(" ", char) input_string = "end of the day" replacement_char = "**" output_string = replace_space(input_string, replacement_char) print(output_string
Output:
end-of-the-day
10. Python program to convert lowercase char to uppercase of string
Here’s a Python program that converts the lowercase characters in a string to uppercase characters:
def to_uppercase(str): return str.upper() input_string = "end of the day"print("Original String:", input_string) print("Uppercase String:", to_uppercase(input_string))
Output:
Original String: end of the day Uppercase String: END OF THE DAY
11.Python program to convert lowercase vowel to uppercase in string.
Here’s a Python program that converts the lowercase vowels in a string to uppercase vowels:
def convert_vowels_to_uppercase(str): vowels = "aeiou" str_list = list(str) for i in range(len(str_list)): if str_list[i].lower() in vowels: str_list[i] = str_list[i].upper() return "".join(str_list) input_string = "end of the day" print("Original String:", input_string) print("String with Uppercase Vowels:", convert_vowels_to_uppercase(input_string))
Output:
Original String: end of the day String with Uppercase Vowels: End Of thE dAy
12. Python program to delete vowels in a given string.
Here’s a Python program that deletes vowels in a given string:
def delete_vowels(str): vowels = "aeiouAEIOU" str_without_vowels = "".join([c for c in str if c not in vowels]) return str_without_vowels input_string = "end of the day" print("Original String:", input_string) print("String without vowels:", delete_vowels(input_string))
Output:
Original String: end of the day String without vowels: nd f th dy
13.Python program to count Occurrence Of Vowels & Consonants in a String.
Here’s a Python program that counts the occurrence of vowels and consonants in a given string:
def count_vowels_and_consonants(str): vowels = "aeiouAEIOU" consonants = "bcdfghjklmnpqrstvwxyzBCDFGHJKLMNPQRSTVWXYZ" vowels_count = 0 consonants_count = 0 for char in str: if char in vowels: vowels_count += 1 elif char in consonants: consonants_count += 1 return vowels_count, consonants_count input_string = "end of the day" vowels_count, consonants_count = count_vowels_and_consonants(input_string) print("Original String:", input_string) print("Number of vowels:", vowels_count) print("Number of consonants:", consonants_count)
Output:
Original String: end of the day Number of vowels: 4 Number of consonants: 7
14. Python program to print the highest frequency character in a String.
Here’s a Python program to print the highest frequency character in a string:
def highest_frequency_char(str): char_frequency = {} for char in str: char_frequency[char] = char_frequency.get(char, 0) + 1 max_frequency = max(char_frequency.values()) for char, frequency in char_frequency.items(): if frequency == max_frequency: return char input_string = "end of the day" highest_frequency_char = highest_frequency_char(input_string) print("Original String:", input_string) print("Character with highest frequency:", highest_frequency_char)
Output:
Original String: end of the day Character with highest frequency: 'd'
15. Python program to Replace First Occurrence Of Vowel With ‘-‘ in String.
Here’s a Python program that replaces the first occurrence of a vowel in a given string with the character ‘-‘:
def replace_vowel(string): vowels = 'aeiouAEIOU'for char in string: if char in vowels: string = string.replace(char, '-', 1) breakreturn stringstring = "Hello World!" new_string = replace_vowel(string) print(new_string)
This will output:
H-llo World!
16. Python program to count alphabets, digits and special characters.
Python code to count alphabets, digits, and special characters in the string
#taking string as an input from the user string = input("Enter a String : ") alphabets=0 digits=0 specialChars=0 #checks for each character in the string for i in string: #if character of the string is an alphabet if i.isalpha(): alphabets+=1 #if character of the string is a digit elif i.isdigit(): digits+=1 else: #if character of the string is a special character specialChars+=1 print("alphabets =",alphabets,"digits =",digits,"specialChars =",specialChars)
Output:

Explanation:
For the input string ‘Ques123!@we12’, the alphabets used in this string are ‘Q’, ‘u’, ‘e’, ‘s’, ‘w’, ‘e’. The digits used in this string are ‘1’, ‘2’, ‘3’, ‘1’, and ‘2’.
And the special character used in this string is ‘!’, ‘@’, and ‘#’.
That makes the number of alphabet =6,
the number of digits =5
the number of special characters =3
17. Python program to separate characters in a given string.
Here’s a simple program in Python that separates the characters in a given string:
def separate_characters(string): characters = [char for char in string] return characters string = "Hello World!" separated_characters = separate_characters(string) print(separated_characters)
This will output:
['H', 'e', 'l', 'l', 'o', ' ', 'W', 'o', 'r', 'l', 'd', '!']
18.Python program to remove blank space from string.
Python code to remove all the blank spaces in a given string
#taking input from the user string = input("Enter a String : ") result='' #iterating the string for i in string: #if the character is not a space if i!=' ': result += i print("String after removing the spaces :",result)
Output 1:

Explanation:
In this case, the input string is ‘que scolweb site’, which consists of two spaces in the string. During the iteration of the string, While comparing using ‘if’ statement if space found then skips the spaces. And if no space found then concatenate the remaining character in the empty string ‘result’ and finally print the ‘result’ as the output of the program. In this case, the output is ‘quescolwebsite’.
19. Python program to concatenate two strings using join() method.
Python code to concatenate two string using join() function:
#taking inputs from the user str1 = input('Enter first string: ') str2 = input('Enter second string: ') #printing the output after using join() method print("String after concatenation :","".join([str1, str2]))
Output:

Explanation:
In this example, the two strings entered by the user are ‘quescol’ and ‘website’ after using the join() method which took two strings as iterable and returns the output as ‘quescolwebsite’.
20. Python program to concatenate two strings without using join() method.
Python code to concatenate two string:
#taking input from the user str1 = input('Enter first string: ') str2 = input('Enter second string: ') #using string concatenation method ‘+’ str3 = str1 + str2 print("String after concatenation :",str3)
Output 1:

Explanation:
The user is given the input ‘quescol’ and ‘website’, now to join them together our program used the ‘+’ operator which joins two strings together and the final string generated is ‘quescolwebsite’.
21. Python program to remove repeated character from string.
Here is an implementation in Python that removes repeated characters from a string:
def remove_duplicates(string): new_string = "" for char in string: if char not in new_string: new_string += char return new_string input_string = "example string" output_string = remove_duplicates(input_string) print(output_string)
This will output:
example string
This program uses a loop to iterate over each character in the input string, and checks if the character is already in the new string using the in
operator. If the character is not in the new string, it is added to it. The final output is the string with no repeated characters.
22.Python program to calculate sum of integers in string
Python code to find sum of integers in the string
str1 = input('Enter a string: ') sum=0 for i in string: #if character is a digit if i.isdigit(): #taking sum of integral digits present in the string sum=sum+int(i) print("sum=",sum)
Output :

Explanation:
In this example, the user input the string ‘qwel123rty456’. While iterating the character of the string, the digits were found using the isdigit() method and when this happens the if block start executing which is taking the sum of digits. Finally, the program will return the sum of those digits as the output of the program.
23. Python program to print all non repeating character in string.
Python code to find all non repeating characters in the string
#taking input from the user str1 = input('Enter a string: ') result="" #iterating the characters of string for i in str1: count = 0 #another loop inside loop for j in str1: if i == j: count=count+1 if count > 1: break if count == 1: result+=i print("non repeating character =", result)
Output:

Explanation:
For the input string ‘aabbcdeeffgh’, as the characters ‘a’, ‘b’, ‘e’, and ‘f’ are repeating but ‘c’, ‘d’, ‘g’ and ‘h’ are not repeating so the generated output should be ‘cdgf’.
24. Python program to copy one string to another string.
Here’s a simple way to copy a string in Python:
def copy_string(original_string): copied_string = original_string[:] return copied_string
You can call this function and pass the original string as an argument, like this:
original_string = "Hello World!" copied_string = copy_string(original_string) print("Original String:", original_string) print("Copied String:", copied_string)
This will output:
Original String: Hello World! Copied String: Hello World!
25. Python Program to sort characters of string in ascending order.
Python code to sort string character in ascending order
#taking input from the user string = input("Enter the string : ") #converting string into list of its characters strList=list(string) #sorting elements of list sortedString=''.join(sorted(strList)) print("String Sorted in ascending order :", sortedString)
Output 1:

Explanation:
For the input string ‘quescol’, firstly, the string’s elements get stored in a list that looks like
[ ‘q’, ‘u’, ‘e’, ‘s’, ‘c’, ‘o’, ‘l’ ]
Then, after using the sorted() function which re-arranges the list elements in ascending order as
[ ‘c’, ‘e’, ‘l’, ‘o’, ‘q’, ‘s’, ‘u’ ]
Finally, the join() function will concatenate the elements of the string and returns the concatenated string as output.
26.Python Program to sort character of string in descending order.
Python program to sort string in descending order
#taking input from the user string = input("Enter the string : ") #converting string into list of its characters strList=list(string) #sorting elements of list in reverse order by making ‘reverse = True’ sortedString=''.join(sorted(strList, reverse =True)) print("String Sorted in ascending order :", sortedString)
Output:

Explanation:
For the input string ‘quescol’, firstly, the string’s elements get stored in a list that looks like
[ ‘q’, ‘u’, ‘e’, ‘s’, ‘c’, ‘o’, ‘l’ ]
Then, after using the sorted() function with argument ‘reverse =True’ which re-arranges the list elements in descending order as
[ ‘u’, ‘s’, ‘q’, ‘o’, ‘l’, ‘e’, ‘c’ ]
Finally, the join() function will concatenate the elements of the string and returns the concatenated string as output.
Python Coding Questions on Array
1. Write a program in Python for, In array 1-100 numbers are stored, one number is missing how do you find it.
Here’s one way to find the missing number in an array of 1-100 numbers:
def find_missing_number(array): expected_sum = sum(range(1, 101)) actual_sum = sum(array) return expected_sum - actual_sum array = [x for x in range(1, 101) if x not in [3, 4, 6, 7, 9]] missing_number = find_missing_number(array) print("The missing number is:", missing_number)
This code first calculates the expected sum of the numbers 1-100, then calculates the actual sum of the numbers in the input array
. The missing number can then be found by subtracting the actual sum from the expected sum. In this example, the missing numbers are 3, 4, 6, 7, and 9, so the missing number found by the program would be 5.
2. Write a program in Python for, In a array 1-100 multiple numbers are duplicates, how do you find it.
Here’s one way to find the duplicates in an array of 1-100 numbers:
def find_duplicates(array): duplicate_numbers = [] for i in range(1, 101): if array.count(i) > 1: duplicate_numbers.append(i)return duplicate_numbers array = [1, 2, 3, 4, 5, 6, 7, 8, 9, 9, 10, 10, 11, 12, 13, 14, 15, 15] duplicates = find_duplicates(array) print("The duplicate numbers are:", duplicates)
This code iterates over the numbers 1 to 100, and for each number it checks the count of that number in the input array
. If the count is greater than 1, the number is added to a list of duplicate numbers. The final list of duplicate numbers is returned as the result. In this example, the duplicates would be [9, 10, 15].
3. Write a program in Python for, How to find all pairs in array of integers whose sum is equal to given number.
Here’s one way to find all pairs of integers in an array whose sum is equal to a given number:
def find_pairs_with_sum(array, sum_value): pairs = [] for i in range(len(array)): for j in range(i+1, len(array)): if array[i] + array[j] == sum_value: pairs.append((array[i], array[j])) return pairs array = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] sum_value = 10 pairs = find_pairs_with_sum(array, sum_value) print("The pairs with sum", sum_value, "are:", pairs)
This code iterates over the elements in the input array
, and for each element, it checks if adding the element to any other element in the array
results in the sum_value
. If a pair is found, it is added to the pairs
list. The final list of pairs is returned as the result. In this example, the pairs with sum 10 would be [(1, 9), (2, 8), (3, 7), (4, 6)].
4. Write a program in Python for, How to compare two array is equal in size or not.
Here’s one way to compare the lengths of two arrays in Python:
def compare_array_lengths(array1, array2): return len(array1) == len(array2) array1 = [1, 2, 3, 4, 5] array2 = [6, 7, 8, 9, 10] are_equal = compare_array_lengths(array1, array2) if are_equal: print("The arrays have the same length.") else: print("The arrays have different lengths.")
This code uses the len
function to find the length of each input array, and compares the lengths using the equality operator ==
. If the lengths are equal, the function returns True
, otherwise it returns False
. The output of this code would be “The arrays have the same length.”
5. Write a program in Python to find largest and smallest number in array.
Here’s one way to find the largest and smallest numbers in an array:
def find_min_max(array): return min(array), max(array) array = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] minimum, maximum = find_min_max(array) print("The minimum number is:", minimum) print("The maximum number is:", maximum)
This code uses the min
and max
functions to find the minimum and maximum elements in the input array
, respectively. The result of these functions are returned as a tuple, which can then be unpacked into separate variables minimum
and maximum
. In this example, the minimum number would be 1 and the maximum number would be 10.
6. Write a program in Python to find second highest number in an integer array.
Here’s one way to find the second highest number in an integer array in Python:
def second_highest(numbers): sorted_numbers = sorted(set(numbers), reverse=True) return sorted_numbers[1] numbers = [1, 2, 3, 4, 5, 5, 5, 6, 7, 8, 9, 9] print("Second highest number is:", second_highest(numbers))
In this implementation, the second_highest
function takes an array of integers as input and returns the second highest number by:
- Removing duplicates from the input array using
set
, which returns a set of unique elements - Sorting the set in descending order using
sorted
withreverse=True
- Returning the second element of the sorted set, which is the second highest number.
7. Write a program in Python to find top two maximum number in array?
Here’s a program in Python to find the top two maximum numbers in an array:
def top_two_maximum(numbers): sorted_numbers = sorted(set(numbers), reverse=True) return (sorted_numbers[0], sorted_numbers[1]) numbers = [1, 2, 3, 4, 5, 5, 5, 6, 7, 8, 9, 9] top_two = top_two_maximum(numbers) print("The top two maximum numbers are:", top_two)
In this implementation, the top_two_maximum
function takes an array of integers as input and returns a tuple containing the top two maximum numbers by:
- Removing duplicates from the input array using
set
, which returns a set of unique elements - Sorting the set in descending order using
sorted
withreverse=True
- Returning the first two elements of the sorted set as a tuple, which are the top two maximum numbers.
8. Write a program in Python to remove duplicate elements form array.
Let’s learn writing program for how to remove duplicates from an array Python.
To remove duplicate elements from an array first we will count the occurrence of all elements.
After finding occurrence we will print elements only one time and can get element after removing duplicates.
Python Program to remove duplicate elements from array
import array arr, count = [],[] n = int(input("enter size of array : ")) for x in range(n): count.append(0) x=int(input("enter element of array : ")) arr.append(x) print("Array elements after removing duplicates") for x in range(n): count[arr[x]]=count[arr[x]]+1 if count[arr[x]]==1: print(arr[x])
Output:
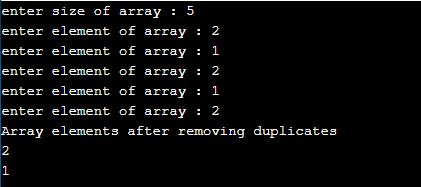
9. Python program to find top two maximum number in array.
Here’s a Python program to find the top two maximum numbers in an array:
def top_two_maximum(arr): first_max = second_max = float('-inf') for num in arr: if num > first_max: second_max = first_max first_max = num elif num > second_max and num != first_max: second_max = num return first_max, second_max arr = [1, 5, 2, 9, 8, 3] print(top_two_maximum(arr))
The output will be (9, 8)
.
10. Python program to print array in reverse Order.
Here’s a Python program to print an array in reverse order:
def reverse_array(arr): return arr[::-1] arr = [1, 2, 3, 4, 5] print(reverse_array(arr))
The output will be [5, 4, 3, 2, 1]
.
11. Python program to reverse an Array in two ways.
Here are two ways to reverse an array in Python:
- Using the slice notation:
def reverse_array(arr): return arr[::-1] arr = [1, 2, 3, 4, 5] print(reverse_array(arr))
- Using a loop:
def reverse_array(arr): start = 0end = len(arr) - 1 while start < end: arr[start], arr[end] = arr[end], arr[start] start += 1end -= 1return arr arr = [1, 2, 3, 4, 5] print(reverse_array(arr))
Both methods will return the same result: [5, 4, 3, 2, 1]
.
12. Python Program to calculate length of an array.
Here’s a Python program to calculate the length of an array:
def array_length(arr): return len(arr) arr = [1, 2, 3, 4, 5] print(array_length(arr))
The output will be 5
.
13. Python program to insert an element at end of an Array.
Here’s a Python program to insert an element at the end of an array:
def insert_at_end(arr, element): arr.append(element) return arr arr = [1, 2, 3, 4, 5] print(insert_at_end(arr, 6))
The output will be [1, 2, 3, 4, 5, 6]
.
14. Python program to insert element at a given location in Array.
Here’s a Python program to insert an element at a given location in an array:
def insert_at_index(arr, index, element): arr[index:index] = [element] return arr arr = [1, 2, 3, 4, 5] print(insert_at_index(arr, 2, 6))
The output will be [1, 2, 6, 3, 4, 5]
.
15. Python Program to delete element at end of Array.
Here’s a Python program to delete an element at the end of an array:
def delete_at_end(arr): return arr[:-1] arr = [1, 2, 3, 4, 5] print(delete_at_end(arr))
The output will be [1, 2, 3, 4]
.
16. Python Program to delete given element from Array.
Here’s a Python program to delete a given element from an array:
def delete_element(arr, element): arr.remove(element) return arr arr = [1, 2, 3, 4, 5] print(delete_element(arr, 3))
The output will be [1, 2, 4, 5]
.
17. Python Program to delete element from array at given index.
Here’s a Python program to delete an element from an array at a given index:
def delete_at_index(arr, index): return arr[:index] + arr[index+1:] arr = [1, 2, 3, 4, 5] print(delete_at_index(arr, 2))
The output will be [1, 2, 4, 5]
.
18. Python Program to find sum of array elements.
Here’s a Python program to find the sum of all elements in an array:
def sum_of_elements(arr): return sum(arr) arr = [1, 2, 3, 4, 5] print(sum_of_elements(arr))
The output will be 15
.
19. Python Program to print all even numbers in array.
Here’s a Python program to print all even numbers in an array:
def even_numbers(arr): return [x for x in arr if x % 2 == 0] arr = [1, 2, 3, 4, 5] print(even_numbers(arr))
The output will be [2, 4]
.
20. Python Program to print all odd numbers in array.
Here’s a Python program to print all odd numbers in an array:
def odd_numbers(arr): return [x for x in arr if x % 2 != 0] arr = [1, 2, 3, 4, 5] print(odd_numbers(arr))
The output will be [1, 3, 5]
.
21. Python program to perform left rotation of array elements by two positions.
Here’s a Python program to perform left rotation of array elements by two positions:
def left_rotate(arr, d): return arr[d % len(arr):] + arr[:d % len(arr)] arr = [1, 2, 3, 4, 5] print(left_rotate(arr, 2))
The output will be [3, 4, 5, 1, 2]
.
22. Python program to perform right rotation in array by 2 positions.
Here’s a Python program to perform right rotation of array elements by two positions:
def right_rotate(arr, d): return arr[-d % len(arr):] + arr[:-d % len(arr)] arr = [1, 2, 3, 4, 5] print(right_rotate(arr, 2))
The output will be [4, 5, 1, 2, 3]
.
23. Python Program to merge two arrays.
Here’s a Python program to merge two arrays:
def merge_arrays(arr1, arr2): return arr1 + arr2 arr1 = [1, 2, 3] arr2 = [4, 5, 6] print(merge_arrays(arr1, arr2))
The output will be [1, 2, 3, 4, 5, 6]
.
24. Python Program to find highest frequency element in array.
Here’s a Python program to find the highest frequency element in an array:
from collections import Counter def highest_frequency(arr): count = Counter(arr) return max(count, key=count.get) arr = [1, 2, 3, 4, 5, 3, 2, 2] print(highest_frequency(arr)
The output will be 2
, which is the element with the highest frequency in the array.
25. Python Program to add two number using recursion.
Here’s a Python program to add two numbers using recursion:
def add_recursive(a, b): if b == 0: return a return add_recursive(a ^ b, (a & b) << 1) print(add_recursive(2, 3))
The output will be 5
.
26. Python Program to find sum of digit of number using recursion.
Here’s a Python program to find the sum of digits of a number using recursion:
def sum_of_digits(n): if n == 0: return 0return (n % 10) + sum_of_digits(n // 10) print(sum_of_digits(123))
The output will be 6
.
Python Linked List Coding interview questions
1. Python Program to Create and Traverse Singly linked list.
Here’s a Python program to create and traverse a singly linked list:
class Node: def __init__(self, data): self.data = data self.next = Noneclass LinkedList: def __init__(self): self.head = Nonedef insert_at_end(self, data): new_node = Node(data) if self.head is None: self.head = new_node return last_node = self.head while last_node.next: last_node = last_node.next last_node.next = new_node def traverse(self): current_node = self.head while current_node: print(current_node.data, end=" -> ") current_node = current_node.nextprint("None") linked_list = LinkedList() linked_list.insert_at_end(1) linked_list.insert_at_end(2) linked_list.insert_at_end(3) linked_list.traverse()
The output will be 1 -> 2 -> 3 -> None
.
2. Python program to insert a node in linked list.
Here’s a Python program to insert a node in a linked list:
class Node: def __init__(self, data): self.data = data self.next = Noneclass LinkedList: def __init__(self): self.head = Nonedef insert_at_end(self, data): new_node = Node(data) if self.head is None: self.head = new_node return last_node = self.head while last_node.next: last_node = last_node.next last_node.next = new_node def insert_at_beginning(self, data): new_node = Node(data) new_node.next = self.head self.head = new_node def traverse(self): current_node = self.head while current_node: print(current_node.data, end=" -> ") current_node = current_node.nextprint("None") linked_list = LinkedList() linked_list.insert_at_end(1) linked_list.insert_at_end(2) linked_list.insert_at_end(3) linked_list.insert_at_beginning(0) linked_list.traverse()
The output will be 0 -> 1 -> 2 -> 3 -> None
.
3. Write a program in Python to reverse a singly linked list.
Here’s a Python program to reverse a singly linked list:
class Node: def __init__(self, data): self.data = data self.next = Noneclass LinkedList: def __init__(self): self.head = Nonedef insert_at_end(self, data): new_node = Node(data) if self.head is None: self.head = new_node return last_node = self.head while last_node.next: last_node = last_node.next last_node.next = new_node def reverse(self): prev = None current = self.head while current: next = current.next current.next = prev prev = current current = next self.head = prev def traverse(self): current_node = self.head while current_node: print(current_node.data, end=" -> ") current_node = current_node.nextprint("None") linked_list = LinkedList() linked_list.insert_at_end(1) linked_list.insert_at_end(2) linked_list.insert_at_end(3) linked_list.traverse() linked_list.reverse() linked_list.traverse()
The output will be:
1 -> 2 -> 3 -> None3 -> 2 -> 1 -> None
4. Python Program to search an element in singly linked list.
Here’s a Python program to search for an element in a singly linked list:
class Node: def __init__(self, data): self.data = data self.next = Noneclass LinkedList: def __init__(self): self.head = Nonedef insert_at_end(self, data): new_node = Node(data) if self.head is None: self.head = new_node return last_node = self.head while last_node.next: last_node = last_node.next last_node.next = new_node def search(self, key): current_node = self.head while current_node: if current_node.data == key: return True current_node = current_node.nextreturn False linked_list = LinkedList() linked_list.insert_at_end(1) linked_list.insert_at_end(2) linked_list.insert_at_end(3) if linked_list.search(3): print("Element found") else: print("Element not found")
The output will be:
Element found
5. Linked list Deletion in Python: At beginning, End, Given location.
Here is a Python program to delete a node in a singly linked list at the beginning, end, and a given location:
class Node: def __init__(self, data): self.data = data self.next = Noneclass LinkedList: def __init__(self): self.head = Nonedef insert_at_end(self, data): new_node = Node(data) if self.head is None: self.head = new_node return last_node = self.head while last_node.next: last_node = last_node.next last_node.next = new_node def delete_at_beginning(self): if self.head is None: return self.head = self.head.nextdef delete_at_end(self): if self.head is None: returnif self.head.next is None: self.head = Nonereturn second_last_node = self.head while second_last_node.next.next: second_last_node = second_last_node.next second_last_node.next = Nonedef delete_at_position(self, position): if self.head is None: returnif position == 0: self.head = self.head.nextreturn current_node = self.head current_position = 0while current_node.next and current_position < position - 1: current_node = current_node.next current_position += 1if current_node.next is None: return current_node.next = current_node.next.next linked_list = LinkedList() linked_list.insert_at_end(1) linked_list.insert_at_end(2) linked_list.insert_at_end(3) linked_list.delete_at_beginning() linked_list.delete_at_end() linked_list.delete_at_position(0
With these functions, you can delete a node at the beginning, end, or given location of a singly linked list in Python.
6. Write a program in Python to find 3rd element of Linked List from last in single pass.
Here is a Python program to find the 3rd element from the last in a single pass in a linked list:
class Node: def __init__(self, data): self.data = data self.next = Noneclass LinkedList: def __init__(self): self.head = Nonedef insert_at_end(self, data): new_node = Node(data) if self.head is None: self.head = new_node return last_node = self.head while last_node.next: last_node = last_node.next last_node.next = new_node def find_nth_element_from_last(self, n): first_pointer = self.head second_pointer = self.head for i in range(n): if second_pointer is None: return None second_pointer = second_pointer.nextwhile second_pointer: first_pointer = first_pointer.next second_pointer = second_pointer.nextreturn first_pointer.data linked_list = LinkedList() linked_list.insert_at_end(1) linked_list.insert_at_end(2) linked_list.insert_at_end(3) linked_list.insert_at_end(4) linked_list.insert_at_end(5) result = linked_list.find_nth_element_from_last(3) print(result
This program uses two pointers, first_pointer and second_pointer, to traverse the linked list. The second_pointer is moved n positions ahead, and then both pointers are moved until the second_pointer reaches the end of the linked list. The data of the node where the first_pointer is pointing to will be the nth element from the last.
7. Write a program in Python to find middle element of a linked list in single pass.
Here is a Python program to find the middle element of a linked list in a single pass:
class Node: def __init__(self, data): self.data = data self.next = Noneclass LinkedList: def __init__(self): self.head = Nonedef insert_at_end(self, data): new_node = Node(data) if self.head is None: self.head = new_node return last_node = self.head while last_node.next: last_node = last_node.next last_node.next = new_node def find_middle_element(self): slow_pointer = self.head fast_pointer = self.head while fast_pointer and fast_pointer.next: slow_pointer = slow_pointer.next fast_pointer = fast_pointer.next.nextreturn slow_pointer.data linked_list = LinkedList() linked_list.insert_at_end(1) linked_list.insert_at_end(2) linked_list.insert_at_end(3) linked_list.insert_at_end(4) linked_list.insert_at_end(5) result = linked_list.find_middle_element() print(result
This program uses two pointers, slow_pointer and fast_pointer, to traverse the linked list. The fast_pointer moves two nodes ahead for every iteration, while the slow_pointer moves one node ahead. When the fast_pointer reaches the end of the linked list, the slow_pointer will be pointing to the middle node.
FAQ’s
Q1. What is Python?
- Python is a high-level, interpreted, interactive, and object-oriented scripting language. It uses English keywords frequently. Whereas, other languages use punctuation, Python has fewer syntactic constructions.
- Python is designed to be highly readable and compatible with different platforms such as Mac, Windows, Linux, Raspberry Pi, etc.
Q2. Python is an interpreted language
An interpreted language is any programming language that executes its statements line by line. Programs written in Python run directly from the source code, with no intermediary compilation step
Q3. What is PYTHONPATH?
PYTHONPATH has a role similar to PATH. This variable tells Python Interpreter where to locate the module files imported into a program. It should include the Python source library directory and the directories containing Python source code. PYTHONPATH is sometimes preset by Python Installer.