Ansible Playbooks
Ansible is a popular open-source automation tool that helps in configuration management, application deployment, and task automation. Ansible uses a simple YAML-based syntax to define tasks and playbooks, making it easy for system administrators and DevOps engineers to automate complex tasks.
In this tutorial, I will guide you through the steps to write Ansible Playbooks with some coding examples.
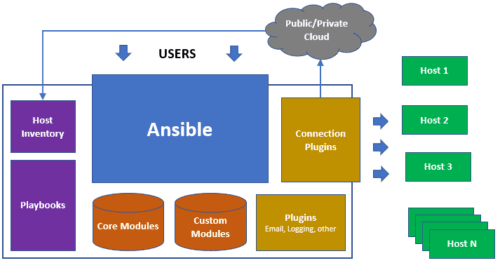
How to write Ansible Playbooks?
To write an Ansible playbook, follow these steps:
- Define the hosts: Define the hosts that the playbook will target. This can be a single host or a group of hosts defined in the inventory file.
- Define variables: Define the variables that will be used in the playbook. Variables can be defined at different levels, such as playbook level, role level, or task level.
- Write tasks: Write the tasks that the playbook will execute. Tasks are defined using Ansible modules and can be used to install packages, copy files, configure services, and more.
- Use conditionals and loops: Use conditionals and loops to execute tasks conditionally or repetitively based on specific conditions.
- Use roles: Use roles to organize and reuse your playbook code.
Here’s an example of a simple Ansible playbook that installs and starts the Apache web server on a group of hosts:
yaml ---- name: Install and start Apachehosts: webserversbecome: truetasks:- name: Install Apacheyum:name: httpdstate: present- name: Start Apacheservice:name: httpdstate: started
In this example, we have defined a playbook named “Install and start Apache”. The playbook targets a group of hosts named “webservers” and runs with elevated privileges using the become
keyword.
The playbook contains two tasks. The first task installs the Apache web server using the yum
module, and the second task starts the Apache web server using the service
module.
You can run this playbook using the ansible-playbook
command:
ansible-playbook apache.yml
This will execute the tasks defined in the playbook on the hosts defined in the inventory file.
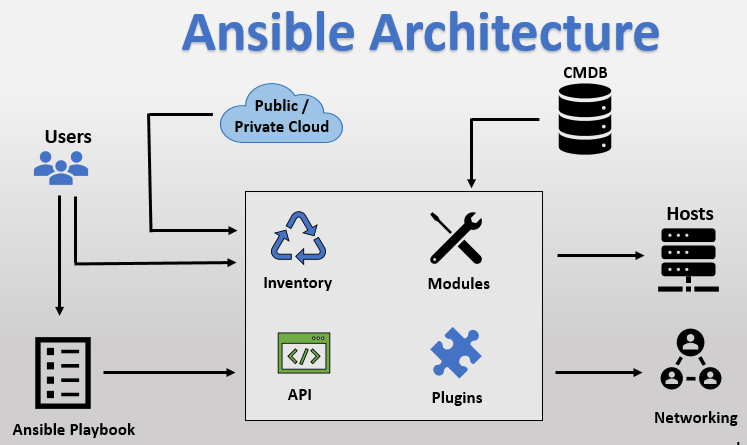
Prerequisites
Before we start with the tutorial, let’s ensure that you have the following prerequisites:
- A Linux-based system for running Ansible.
- Ansible installed on your system.
- Basic knowledge of YAML syntax.
Step 1: Understanding Ansible Playbooks
Ansible Playbooks are files containing a set of tasks to be executed on remote hosts. Each playbook consists of one or more plays, where a play is a collection of tasks to be executed on a specific group of hosts. Ansible Playbooks are written in YAML format and are easy to read and understand.
Step 2: Writing Your First Ansible Playbook
To get started, create a new file with the .yml
extension and add the following code:
yaml ---- name: Install and start Apachehosts: allbecome: yestasks:- name: Install Apacheapt:name: apache2state: present- name: Start Apacheservice:name: apache2state: started
Let’s break down this playbook:
- The
---
at the beginning of the file indicates that this is a YAML file. - The
name
field is used to give the playbook a descriptive name. - The
hosts
field specifies the group of hosts on which the tasks will be executed. - The
become
field is used to specify that we need to become a privileged user (i.e.,sudo
) to execute the tasks. - The
tasks
section contains a list of tasks to be executed.
In this playbook, we have two tasks:
- The first task installs Apache using the
apt
module. - The second task starts the Apache service using the
service
module.
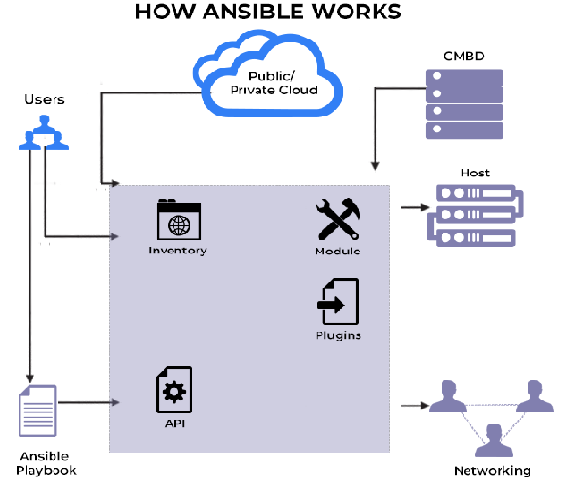
Step 3: Running the Ansible Playbook
To run the playbook, use the following command:
perl ansible-playbook my-playbook.yml
Replace my-playbook.yml
with the name of your playbook file.
If everything goes well, you should see the output indicating that Apache has been installed and started on the remote hosts.
Step 4: Adding Variables to Your Playbook
In Ansible, variables can be used to store values that can be used across the playbook. You can define variables at the playbook level or at the task level.
Let’s modify our playbook to use variables:
yaml ---- name: Install and start Apachehosts: allbecome: yesvars:apache_port: 80tasks:- name: Install Apacheapt:name: apache2state: present- name: Configure Apachetemplate:src: apache.conf.j2dest: /etc/apache2/apache2.confowner: rootgroup: rootmode: '0644'- name: Start Apacheservice:name: apache2state: started
Here, we have defined a variable named apache_port
with a value of 80
. We have also added a new task to configure Apache using a template file (apache.conf.j2
).
Step 5: Using Loops in Your Playbook
Loops can be used in Ansible Playbooks to perform repetitive tasks
For example, let’s say you want to create multiple users on a remote host. Instead of writing a separate task for each user, you can use a loop to create all the users in a single task.
Let’s modify our playbook to create multiple users using a loop:
yaml ---- name: Create Usershosts: allbecome: yesvars:users:- name: user1password: password1- name: user2password: password2- name: user3password: password3tasks:- name: Create Usersuser:name: "{{ item.name }}"password: "{{ item.password }}"state: presentwith_items: "{{ users }}"
In this example, we have defined a variable named users
that contains a list of users to be created, along with their passwords.
We have then added a new task to create the users using the user
module. The with_items
keyword is used to loop through each item in the users
list and create the users.
Step 6: Using Conditionals in Your Playbook
Ansible Playbooks also support conditionals that allow you to execute tasks based on specific conditions. For example, you may want to execute a task only if a specific file exists on the remote host.
Let’s modify our playbook to use conditionals:
yaml ---- name: Copy File if Existshosts: allbecome: yesvars:source_file: /path/to/local/filedestination_file: /path/to/remote/filetasks:- name: Check if File Existsstat:path: "{{ destination_file }}"register: file_stat- name: Copy Filecopy:src: "{{ source_file }}"dest: "{{ destination_file }}"when: file_stat.stat.exists
In this example, we have defined two variables source_file
and destination_file
. We have then added a task to check if the destination_file
exists on the remote host using the stat
module. The result of this task is stored in the file_stat
variable using the register
keyword.
We have then added a second task to copy the source_file
to the destination_file
only if the file exists on the remote host. We have used the when
keyword to specify the condition.
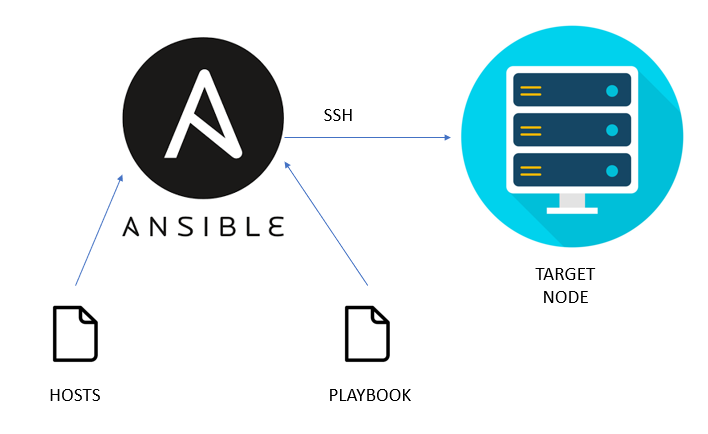
Step 7: Using Roles in Your Playbook
Roles in Ansible are a way of organizing and reusing your playbooks. A role consists of a set of tasks, files, templates, and variables that can be used across multiple playbooks.
To create a role, create a new directory with the role name under the roles
directory in your Ansible project. For example, if you want to create a role named webserver
, create a directory named webserver
under the roles
directory.
A typical role directory structure looks like this:
css webserver/ ├── tasks/ │ ├── main.yml │ └── other-task.yml ├── files/ │ ├── file1 │ └── file2 ├── templates/ │ ├── template1.j2 │ └── template2.j2 ├── vars/ │ ├── main.yml │ └── other-vars.yml ├── defaults/ │ ├── main.yml │ └── other-defaults.yml ├── handlers/ │ ├── main.yml │ └── other-handler.yml └── meta/ └── main.yml
The tasks
directory contains the main tasks that will be executed by the role. The files
directory contains files that will be copied to the remote host. The templates
directory contains Jinja2 templates that will be rendered and copied to the remote host. The vars
directory contains variables that will be used by the role. The defaults
directory contains default variables that can be overridden by the user. The handlers
directory contains handlers that will be triggered by events. The meta
directory contains metadata about the role, such as its author and dependencies.
Once you have created a role, you can use it in your playbook using the roles
keyword.
For example, if you want to use the webserver
role in your playbook, you can do it like this:
yaml ---- name: Example Playbookhosts: allbecome: yesroles:- webserver
In this example, we have added the webserver
role to our playbook using the roles
keyword. Ansible will automatically look for the webserver
role under the roles
directory and execute its tasks.
Conclusion
Ansible Playbooks are a powerful tool for automating tasks on remote hosts. In this tutorial, we have covered the basics of Ansible Playbooks and shown you how to create your own playbook. We have covered variables, loops, conditionals, and roles, which are essential concepts in Ansible Playbooks.
By mastering Ansible Playbooks, you can automate repetitive tasks, reduce errors, and save time. You can also use Ansible Playbooks to provision servers, configure software, and deploy applications.
I hope this tutorial has been helpful in getting you started with Ansible Playbooks. Happy automating!