Failure in Cloud Computing Projects
Introduction:
Cloud computing has become increasingly popular in recent years, as it allows organizations to access powerful and scalable computing resources on demand. However, despite the many benefits of cloud computing, there are also a number of potential causes of failure in cloud computing projects. These include
This article focuses on the following pointers:
- Lack of proper Planning and Design
- Insufficient Testing and Quality Assurance
- Inadequate Security
- Inadequate Monitoring and Management
- Lack of proper training and Education
- Lack of integration with existing Systems
- Cost Over-Runs
- Vendor Lock-In:
- Limited flexibility
Lack of proper Planning and Design:
One of the most common causes of failure in cloud computing projects is a lack of proper planning and design. This can include failing to properly assess the organization’s needs, failing to properly design the architecture and infrastructure, and failing to properly plan for scalability and security.
Insufficient Testing and Quality Assurance:
Another common cause of failure in cloud computing projects is insufficient testing and quality assurance. This can include failing to properly test the system’s performance and scalability, failing to properly test security and data integrity, and failing to properly test the system’s compatibility with other systems.
Inadequate Security:
A third common cause of failure in cloud computing projects is inadequate security. This can include failing to properly secure the system, failing to properly secure data, and failing to properly implement security best practices.
Inadequate Monitoring and Management:
A fourth common cause of failure in cloud computing projects is inadequate monitoring and management. This can include failing to properly monitor the system’s performance and availability, failing to properly manage and maintain the system, and failing to properly handle and respond to incidents.
Lack of proper training and Education:
A fifth common cause of failure in cloud computing projects is the lack of proper training and education. This can include failing to properly train users and administrators on how to use the system, failing to properly educate users and administrators on the system’s capabilities and limitations, and failing to properly educate users and administrators on security best practices.
Here’s a sample code that shows how to create a function to check the available disk space on a cloud storage service, like AWS S3
import boto3 def check_disk_space(bucket_name): # create an S3 client s3 = boto3.client('s3') # get the bucket's usage statistics usage = s3.get_bucket_metrics_configuration( Bucket=bucket_name ) # extract the amount of used storage from the usage statistics used_storage = usage['Metrics']['Storage']['Value'] # check if the used storage is above a certain threshold if used_storage > threshold: print("The bucket's storage is above the threshold.") else: print("The bucket's storage is below the threshold.")
It’s important to keep in mind that these are just a few examples, and there can be many other causes of failure in cloud computing projects. Therefore, it’s essential to carefully plan and design your cloud computing project, test it thoroughly, implement strong security measures, monitor and manage the system effectively, and provide proper training and education to users and administrators.
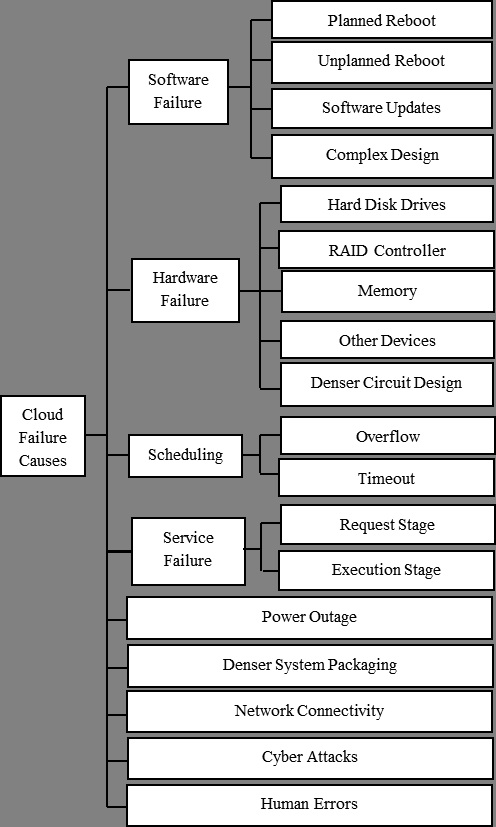
fig: Causes of Failure in Cloud Computing
Lack of integration with existing Systems:
Another common cause of failure in cloud computing projects is the lack of proper integration with existing systems. This can include failing to properly integrate the cloud-based system with on-premises systems, failing to properly integrate the cloud-based system with other cloud-based systems, and failing to properly integrate the cloud-based system with other third-party systems.
Cost Over-Runs:
Cloud computing can be very cost-effective, but it’s also important to keep an eye on costs. Some organizations may not have an accurate understanding of the costs associated with cloud computing, such as data transfer costs, storage costs, and costs associated with scaling resources. This can lead to cost overruns and unexpected expenses.
endor Lock-In:
Cloud computing often involves using third-party providers, but this can also lead to vendor lock-in. This means that an organization becomes dependent on a single provider and finds it difficult to switch to another provider or move back to on-premises systems.
Limited flexibility:
Cloud computing can be very flexible, but it’s also important to keep in mind that there can be limitations. For example, some cloud-based systems may not be able to handle certain types of workloads, or may not have the same level of customization options as on-premises systems.
Compliance and regulatory issues:
Organizations are also responsible for ensuring that cloud-based systems comply with relevant laws, regulations, and standards. Failure to comply with these requirements can result in penalties and legal issues.
Here’s an example of how to use the AWS SDK for Python (boto3) to encrypt and decrypt data stored in an S3 bucket:
import boto3 def encrypt_data(bucket_name, object_name): # create an S3 client s3 = boto3.client('s3') # encrypt the data using the S3 encryption client s3.put_object( Bucket=bucket_name, Key=object_name, Body=data, ServerSideEncryption='AES256' ) def decrypt_data(bucket_name, object_name): # create an S3 client s3 = boto3.client('s3') # download the encrypted data response = s3.get_object( Bucket=bucket_name, Key=object_name ) encrypted_data = response['Body'].read() # decrypt the data using the AWS KMS service kms = boto3.client('kms') decrypted_data = kms.decrypt( CiphertextBlob=encrypted_data ) return decrypted_d
In conclusion, it’s important to be aware of the potential causes of failure in cloud computing projects and take steps to mitigate them. Proper planning, design, testing, security, monitoring, management, education, integration, cost management, flexibility, compliance, and vendor selection are all key factors that can help ensure the success of a cloud computing project.
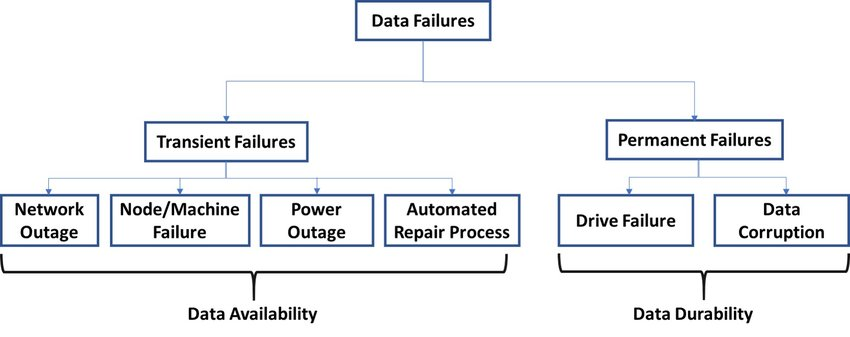
fig: Data failures in cloud storage
Limited Scalability:
Cloud computing is often touted as being highly scalable, but there can be limitations to this scalability. For example, some cloud-based systems may not be able to handle sudden traffic spikes or may not have the ability to scale resources quickly enough to meet demand
Limited Availability:
Cloud-based systems are often built to be highly available, but there can be situations where the system becomes unavailable, such as during maintenance or due to network or power outages. This can be a problem if the system is critical to an organization’s operations.
Limited Customization:
Cloud-based systems can be highly customizable, but there may be limitations to what can be customized. For example, some cloud-based systems may not have the ability to add custom features or may not allow for certain types of customization.
Limited Control:
Organizations may not have the same level of control over cloud-based systems as they would over on-premises systems. For example, organizations may not have direct access to the underlying hardware and may not be able to configure the system as they would like.
Limited Data Sovereignty:
Data sovereignty refers to the legal and regulatory requirements that organizations must comply with when it comes to data storage and processing. In some cases, organizations may not be able to store or process data in certain locations or may not be able to move data to different locations as needed.
An example of how to use AWS Elastic Container Service(ECS) and CloudFormation to handle a scalable and highly available containerized application:
import boto3 def deploy_containerized_app(app_name, container_image, desired_count): # create an ECS client ecs = boto3.client('ecs') # create a task definition for the containerized app task_definition = ecs.register_task_definition( family=app_name, containerDefinitions=[ { 'name': app_name, 'image': container_image, 'memory': 512, 'cpu': 256, 'portMappings': [ { 'containerPort': 80, 'hostPort': 80 } ] } ] ) # create a CloudFormation stack for the containerized app cloudformation = boto3.client('cloudformation') stack_name = app_name + '-stack' stack = cloudformation.create_stack( StackName=stack_name, TemplateBody=''' { "Resources": { "TaskDefinition": { "Type": "AWS::ECS::TaskDefinition", "Properties": { "Family": "''' + app_name + '''", "ContainerDefinitions": [ { "Name": "''' + app_name + '''", "Image": "''' + container_image + '''", "Memory": 512, "Cpu": 256, "PortMappings": [ { "ContainerPort": 80, "HostPort": 80 } ] } ] } }, "Service": { "Type": "AWS::ECS::Service", "Properties": {
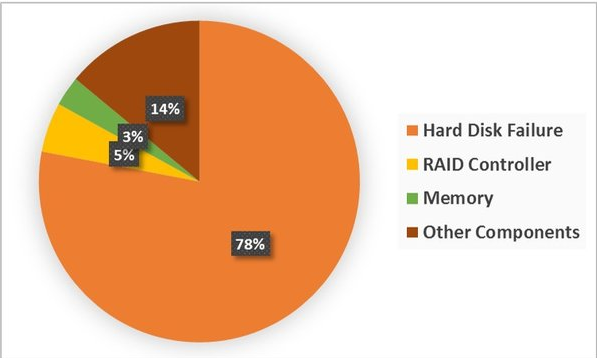
fig: Causes of server failures in cloud computing systems
Cluster": { "Ref": "ECSCluster" }, "TaskDefinition": { "Ref": "TaskDefinition" }, "DesiredCount": '''+ desired_count + ''', "LaunchType": "FARGATE", "LoadBalancer": { "ContainerName": "''' + app_name + '''", "ContainerPort": 80 } } } } } ''' ) print("Stack created with name: " + stack_name)
The above code creates a task definition for a containerized application and a CloudFormation stack for the application, which creates an ECS service to launch the task definition on Fargate with a desired count and a load balancer.
In summary, cloud computing can provide many benefits, but it’s important to be aware of the potential causes of failure in cloud computing projects and take steps to mitigate them. Proper planning, design, testing, security, monitoring, management, education, integration, cost management, flexibility, scalability, availability, customization, control, data sovereignty, compliance, and vendor selection are all key factors that can help ensure the success of a cloud computing project.