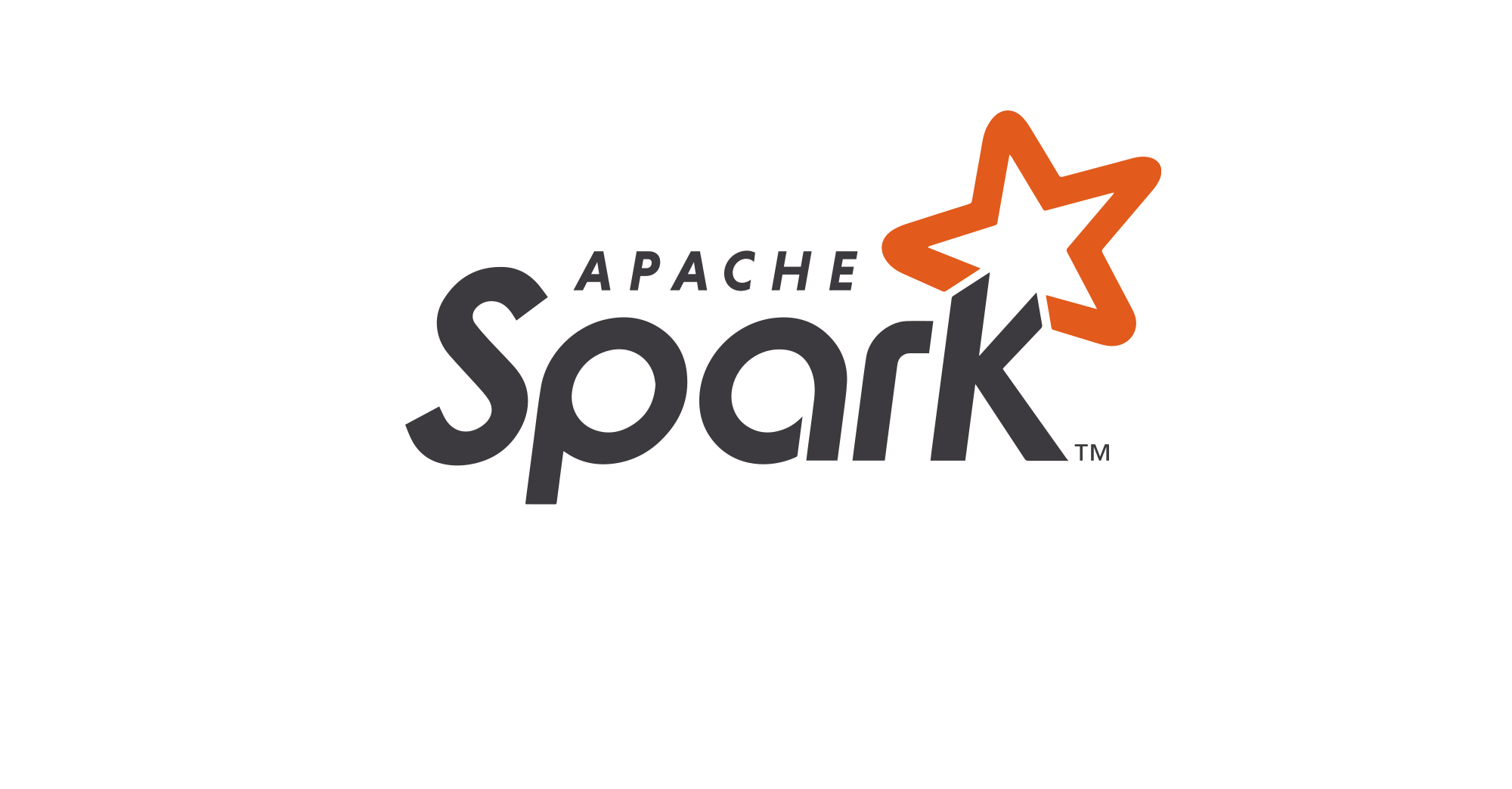
Apache Spark A Comprehensive Guide
Apache Spark is a popular open-source distributed computing system designed for large-scale data processing. It provides an efficient and powerful engine for processing big data workloads with an easy-to-use API. In this comprehensive guide, we’ll cover everything you need to know to get started with Apache Spark.
Spark is designed to handle batch processing, real-time data processing, machine learning, and graph processing, making it a versatile and powerful tool for data engineers, data scientists, and big data professionals.
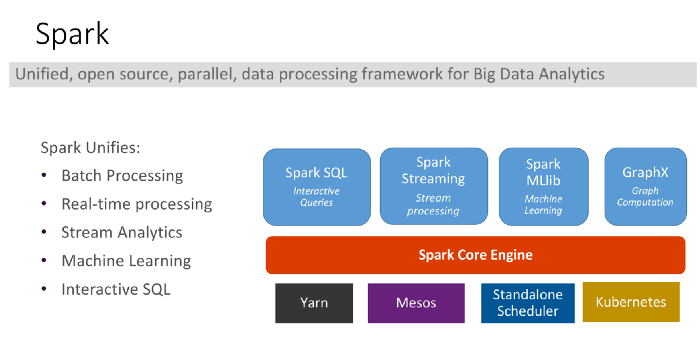
This article aims to introduce the reader to Apache Spark and provide a basic understanding of its key features, architecture, and use cases. Whether you are a beginner looking to learn about Apache Spark or an experienced professional looking to expand your knowledge, this guide will provide a comprehensive introduction to help you get started with Spark.
What is Apache Spark?
Apache Spark is a fast and powerful open-source distributed computing system used for big data processing and analytics. It provides a unified engine for processing large amounts of data in a distributed manner across multiple nodes in a cluster.
Spark allows developers to write code in multiple languages, including Java, Scala, Python, and R. It also provides a range of libraries for various tasks such as SQL processing, machine learning, graph processing, and streaming data processing.
Spark’s key features include its ability to cache data in-memory, which makes it faster than traditional Hadoop MapReduce jobs, and its ability to process data in real-time using its streaming capabilities. Spark also offers a variety of deployment options, including running on a cluster manager like Apache Mesos or Hadoop YARN, or running on a standalone cluster.
Apache Spark was developed at the University of California, Berkeley’s AMPLab in 2009 and donated to the Apache Software Foundation in 2013. Since then, it has become one of the most widely used big data processing frameworks.
Architecture of Apache Spark
1. The Spark driver
The driver is the process “in the driver seat” of your Spark Application. It is the controller of the execution of a Spark Application and maintains all of the states of the Spark cluster (the state and tasks of the executors). It must interface with the cluster manager in order to actually get physical resources and launch executors.
At the end of the day, this is just a process on a physical machine that is responsible for maintaining the state of the application running on the cluster.
2. The Spark executors
Spark executors are the processes that perform the tasks assigned by the Spark driver. Executors have one core responsibility: take the tasks assigned by the driver, run them, and report back their state (success or failure) and results. Each Spark Application has its own separate executor processes.
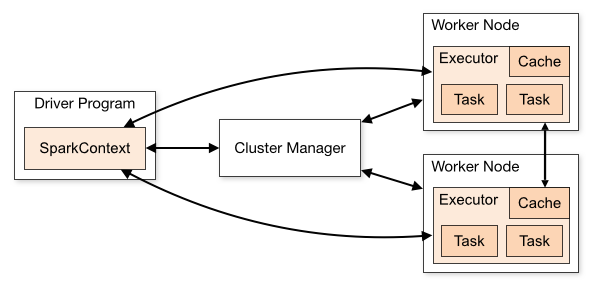
3. The cluster manager
The Spark Driver and Executors do not exist in a void, and this is where the cluster manager comes in. The cluster manager is responsible for maintaining a cluster of machines that will run your Spark Application(s). Somewhat confusingly, a cluster manager will have its own “driver” (sometimes called master) and “worker” abstractions.
The core difference is that these are tied to physical machines rather than processes (as they are in Spark). The machine on the left of the illustration is the Cluster Manager Driver Node. The circles represent daemon processes running on and managing each of the individual worker nodes. There is no Spark Application running as of yet—these are just the processes from the cluster manager.
When the time comes to actually run a Spark Application, we request resources from the cluster manager to run it. Depending on how our application is configured, this can include a place to run the Spark driver or might be just resources for the executors for our Spark Application. Over the course of Spark Application execution, the cluster manager will be responsible for managing the underlying machines that our application is running on.
Key Features of Apache Spark
Apache Spark is a fast, open-source big data processing engine that allows developers to perform distributed data processing tasks with ease. Some of the key features of Apache Spark include:
- In-Memory Processing: Apache Spark stores data in memory, allowing it to perform fast and efficient data processing. This feature makes it faster than Hadoop when it comes to processing large datasets.
- Fault Tolerance: Apache Spark can recover from failures and continue processing without any data loss. This feature is essential for big data processing since there is always a risk of hardware or software failures.
- Data Processing: Apache Spark provides an extensive set of data processing APIs such as SQL, streaming, machine learning, and graph processing.
- Cluster Computing: Apache Spark provides a cluster computing framework for distributed processing. It allows developers to distribute data and processing tasks across multiple nodes, making it highly scalable.
- Integration with Hadoop: Apache Spark can be easily integrated with Hadoop, enabling developers to use Hadoop’s distributed file system (HDFS) and other Hadoop ecosystem tools.
- Easy to use: Apache Spark is easy to use and provides a user-friendly interface for developers. It provides APIs for various programming languages such as Python, Java, and Scala, making it accessible for a wide range of developers.
- Real-time Stream Processing: Apache Spark provides APIs for real-time stream processing, enabling developers to process data in real-time as it is generated.
Overall, Apache Spark provides a powerful set of features for big data processing that make it highly efficient, scalable, and easy to use.
Components of Apache Spark
Apache Spark is comprised of several components that work together to enable distributed data processing. The main components of Apache Spark include:
- Spark Core: This is the foundation of the Apache Spark architecture and provides APIs for distributed task scheduling, memory management, and fault recovery.
- Spark SQL: This component provides APIs for working with structured and semi-structured data using SQL-like queries. It allows developers to interact with structured data using familiar SQL syntax.
- Spark Streaming: This component provides APIs for real-time stream processing of data. It enables developers to process and analyze data as it is generated, making it useful for real-time data processing tasks.
- Spark MLlib: This is the machine learning library for Apache Spark that provides a set of APIs for performing machine learning tasks such as classification, regression, and clustering.
- GraphX: This component provides APIs for processing graph data and performing graph computations. It allows developers to build and analyze graph data structures efficiently.
- SparkR: This is the R programming language API for Apache Spark. It enables R developers to use Apache Spark for data processing and analysis tasks.
- PySpark: This is the Python programming language API for Apache Spark. It enables Python developers to use Apache Spark for data processing and analysis tasks.
- Spark Cluster Manager: Apache Spark can be deployed on various cluster managers such as Apache Mesos, Hadoop YARN, and Kubernetes.
Overall, these components work together to provide a comprehensive platform for distributed data processing and analysis.
Getting Started with Apache Spark
Getting started with Apache Spark requires a few key steps:
- Install Apache Spark: Apache Spark can be downloaded and installed from the official website. You will need to choose a distribution that matches your operating system and install it on your local machine.
- Set up a development environment: Once Apache Spark is installed, you will need to set up a development environment. This typically involves installing a suitable IDE or text editor for your programming language of choice and configuring it to work with Apache Spark.
- Choose a programming language: Apache Spark supports several programming languages, including Java, Scala, Python, and R. Choose the language that you are most comfortable with and start learning the relevant APIs.
- Learn the APIs: Apache Spark provides several APIs for different components, such as Spark SQL, Spark Streaming, and MLlib. Learn the APIs that are most relevant to your use case and start building applications.
- Use the documentation and resources: Apache Spark provides extensive documentation, tutorials, and examples on their website. Use these resources to learn more about Apache Spark and how to use it effectively.
- Start building applications: Once you are comfortable with the basics, start building applications with Apache Spark. Start with simple applications and gradually build more complex ones as you gain more experience.
Getting started with Apache Spark requires installing and setting up the platform, choosing a programming language, learning the relevant APIs, and building applications using the available resources and documentation.
Write your first Apache Spark program
Now that you’ve set up your environment, you’re ready to write your first Apache Spark program. Here’s an example of a simple Apache Spark program in Python that reads a CSV file and calculates the average of a specific column using Spark SQL:
# Import the necessary libraries
from pyspark.sql import SparkSession
# Create a SparkSession
spark = SparkSession.builder.appName("MyFirstSparkProgram").getOrCreate()
# Load the CSV file into a DataFrame
dataframe = spark.read.csv("file_path/file_name.csv", header=True, inferSchema=True)
# Register the DataFrame as a temporary table
dataframe.createOrReplaceTempView("my_table")
# Run a SQL query on the DataFrame
result = spark.sql("SELECT AVG(column_name) FROM my_table")
# Print the result
result.show()
# Stop the SparkSession
spark.stop()
In this example, we first import the necessary libraries and create a SparkSession with a given name. We then load the CSV file into a DataFrame using the spark.read.csv()
method and register the DataFrame as a temporary table using the createOrReplaceTempView()
method. We then run a SQL query on the DataFrame using the spark.sql()
method and calculate the average of a specific column. Finally, we print the result using the show()
method and stop the SparkSession using the spark.stop()
method.
Run your Apache Spark program
To run your Apache Spark program, you’ll need to use the spark-submit script that comes with Apache Spark. Here’s an example command:
$SPARK_HOME/bin/spark-submit --master local path/to/your
Difference between Apache spark and pyspark
Here’s a table outlining the main differences between Apache Spark and PySpark:
Feature | Apache Spark | PySpark |
---|---|---|
Programming languages | Java, Scala, Python, R | Python |
API | Core, SQL, Streaming, MLlib, GraphX, R | PySpark |
Deployment | Standalone, YARN, Mesos, Kubernetes, cloud | Standalone, YARN, Mesos, Kubernetes, cloud |
Ease of use | Requires knowledge of Java, Scala, or R | Easy to use and learn for Python developers |
Performance | High performance and scalability | Similar performance as Java and Scala APIs, but may be slower than native Python libraries |
Development environment | Requires installation of Java, Scala, or R environment | Only requires installation of Python environment |
Integration with Python libraries | Requires additional libraries or custom code | Seamless integration with Python libraries |
Community support | Large community with active development and support | Large community with active development and support |
Overall, while Apache Spark provides support for multiple programming languages and a comprehensive suite of APIs, PySpark is specifically designed for Python developers and offers ease of use, seamless integration with Python libraries, and a simpler development environment. However, PySpark may have slightly lower performance than the native Java or Scala APIs, and may require additional libraries or custom code to integrate with non-Python libraries.
Conclusion…
Apache Spark is a powerful distributed computing platform that is widely used for processing and analyzing large datasets. It provides a range of components, including Spark Core, Spark SQL, Spark Streaming, Spark MLlib, GraphX, SparkR, PySpark, and a cluster manager that work together to enable distributed data processing at scale. With its support for multiple programming languages, extensive documentation, and tutorials, Apache Spark has become a popular choice for data scientists and developers working on big data projects. As the volume and complexity of data continue to grow, Apache Spark will remain a valuable tool for processing and analyzing data efficiently and at scale.