Python Data Types
In Python programming language, everything is an object. Thus, data types are treated as classes and variables are an instance or object of these classes. There are various data types in Python to represent the types of value.Â
Python has several built-in data types that are used to store different kinds of values. These data types are essential for working with data in Python and play a crucial role in any Python program.
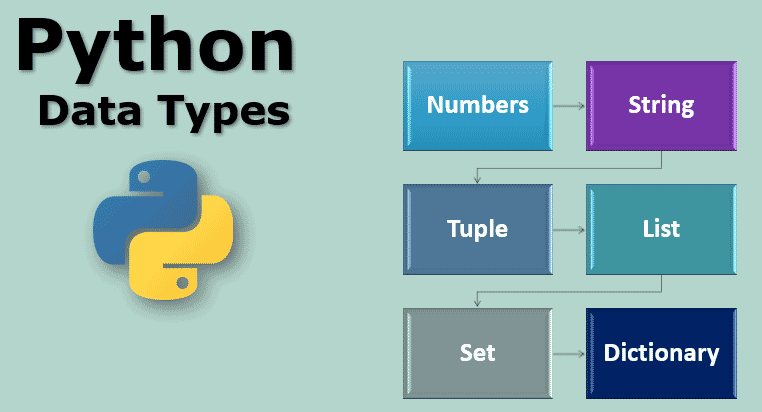
Python Data Types
Variables are used to hold values for different data types. As Python is a dynamically typed language, you don’t need to define the type of the variable while declaring it. The interpreter implicitly binds the value with its type. Python enables us to check the type of the variable used in the program. With the help of the type() function, you can find out the type of the variable passed.
In Python, data can be classified into the following data types:
In Python, unlike statically typed languages like C or Java, there is no need to specifically declare the data type of the variable. In dynamically typed languages such as Python, the interpreter itself predicts the data type of the Python Variable based on the type of value assigned to that variable.
Numeric Types:
- int (integer)
- float (floating point number)
- complex (complex number)
Sequence Types:
- list (ordered, mutable)
- tuple (ordered, immutable)
- range (ordered, immutable)
Text Type:
- str (string of characters)
Mapping Type:
- dict (unordered, mutable)
Set Types:
- set (unordered, mutable, unique elements)
- frozenset (unordered, immutable, unique elements)
Boolean Type:
- bool (True or False)
Binary Types:
- bytes (immutable sequence of bytes)
- bytearray (mutable sequence of bytes)
Others:
- NoneType (represents the absence of a value)
Note: Python is a dynamically-typed language, meaning the type of a variable can change during runtime.
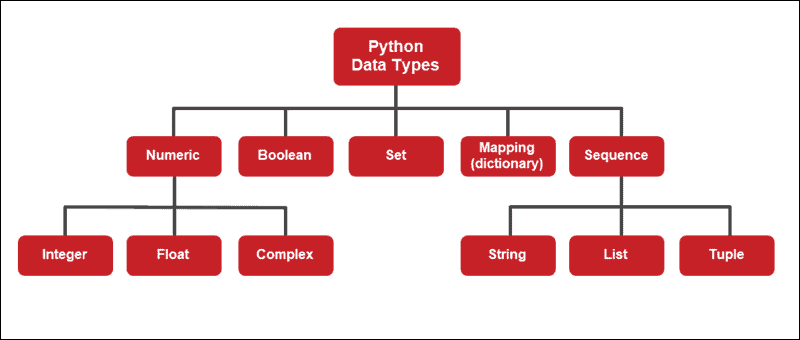
Here are some of the most important data types you need to know:
Numbers:
This data type is used to store numerical values and includes integers, floating-point numbers, and complex numbers.
makefile # Integers x = 10 y = -5 # Floating-point numbers a = 10.5 b = -3.14 # Complex numbers z = 3 + 4
Strings:
This data type is used to store sequences of characters, such as words or sentences. Strings are enclosed in quotes, either single or double quotes.
makefile name = "John Doe" message = 'Hello World'
Lists:
This data type is used to store collections of values that can be of any data type, including numbers and strings. Lists are ordered and mutable, meaning you can add or remove items after they have been created.
css fruits = ["apple", "banana", "cherry"] numbers = [1, 2, 3, 4, 5]
Tuples:
This data type is similar to lists, but tuples are immutable, meaning their values cannot be changed after they have been created.
makefile coordinates = (10, 20) colors = ("red", "green", "blue")
Dictionaries:
This data type is used to store collections of key-value pairs, where each key maps to a unique value. Dictionaries are unordered and mutable.
python person = {"name": "John Doe", "age": 30, "city": "New York"} # Accessing valuesprint(person["name"]) # John Doe
Sets:
This data type is used to store collections of unique values. Sets are unordered and mutable.
makefile numbers = {1, 2, 3, 4, 5} fruits = {"apple", "banana", "cherry"}
These are some of the most important data types in Python. Understanding these data types is crucial for working with data in Python and writing efficient programs.
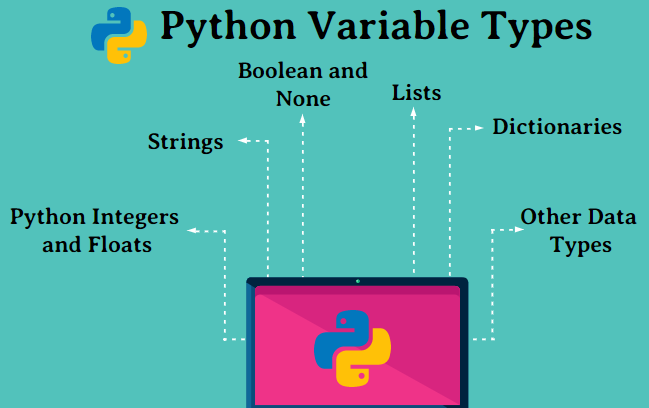
let’s continue exploring Python data types.
Booleans:
This data type is used to represent truth values, either True
or False
. They are often used in conditional statements and looping constructs to control the flow of a program.
python flag = True is_valid = False
Arrays:
This data type is used to store collections of values of the same data type, such as numbers. Unlike lists and tuples, arrays are created using the array
module in Python, and they are more efficient for numerical computations.
c import array numbers = array.array("i", [1, 2, 3, 4, 5])
Bytes:
This data type is used to store binary data, such as images or audio files. Bytes are similar to strings, but they are immutable and can only store values in the range 0-255.
makefile binary_data = b"Hello World" image_data = b'\x89PNG\r\n\x1a\n\x00\x00\x00\rIHDR\x00\x00\x00\x05\x00\x00\x00\x05\x08\x02\x00\x00\x00\x02\xd5\x1c\xb0'
None:
This data type is used to represent the absence of a value. It is often used as a default value for function arguments or to represent the result of a function that does not return anything.
python result = Nonedef do_something(): pass result = do_something() print(result) # None
These are the most common data types in Python. Depending on the task at hand, you may need to use additional data types, such as functions, modules, or classes. However, these data types provide a solid foundation for working with data in Python and building complex programs.
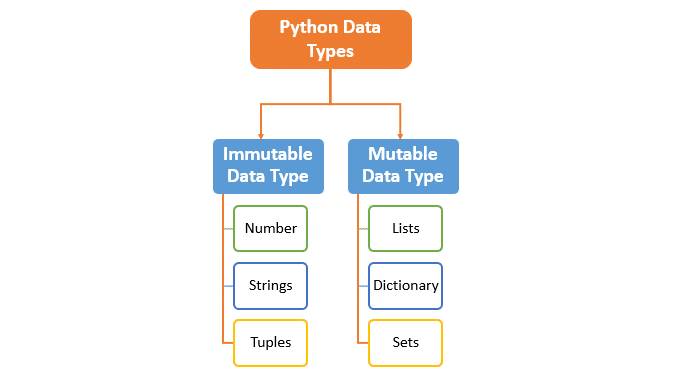
Core Data Types in Python:
In Python, the core data types are:
Numeric Types:
- int (integer)
- float (floating point number)
- complex (complex number)
Sequence Types:
- list (ordered, mutable)
- tuple (ordered, immutable)
- range (ordered, immutable)
Text Type:
- str (string of characters)
Mapping Type:
- dict (unordered, mutable)
Set Types:
- set (unordered, mutable, unique elements)
- frozenset (unordered, immutable, unique elements)
Boolean Type:
- bool (True or False)
None Type:
- None (represents the absence of a value)
Note: These data types are the basic building blocks of Python, and all other data types are built using these core data types.
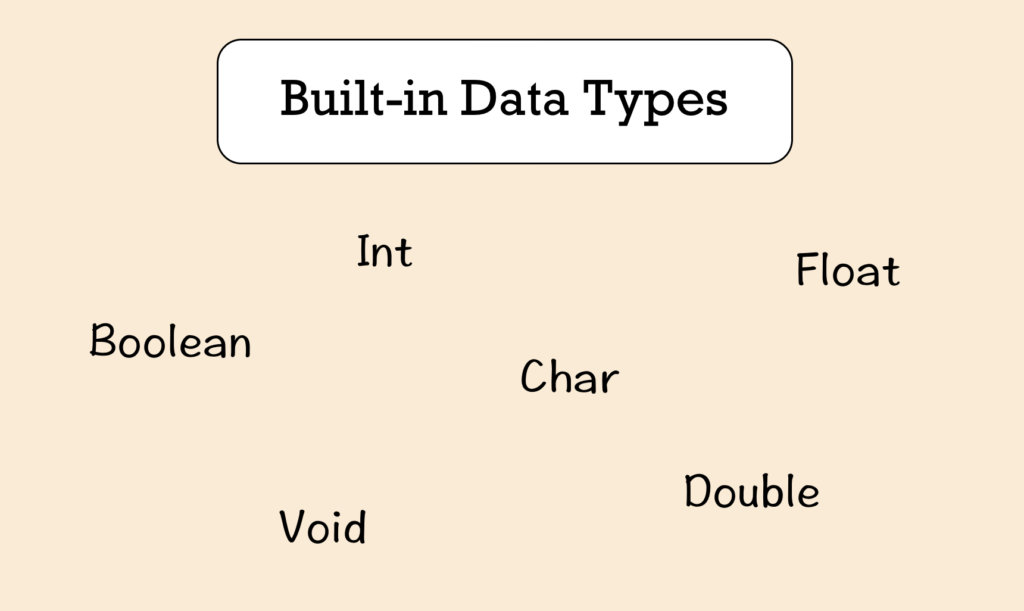
Built-in Data Types in Python:
In Python, the built-in data types are:
Numeric Types:
- int (integer)
- float (floating point number)
- complex (complex number)
Sequence Types:
- list (ordered, mutable)
- tuple (ordered, immutable)
- range (ordered, immutable)
Text Type:
- str (string of characters)
Mapping Type:
- dict (unordered, mutable)
Set Types:
- set (unordered, mutable, unique elements)
- frozenset (unordered, immutable, unique elements)
Boolean Type:
- bool (True or False)
Binary Types:
- bytes (immutable sequence of bytes)
- bytearray (mutable sequence of bytes)
None Type:
- None (represents the absence of a value)
These data types are built into the Python language and are always available to the programmer. They do not need to be imported from any external module or library.
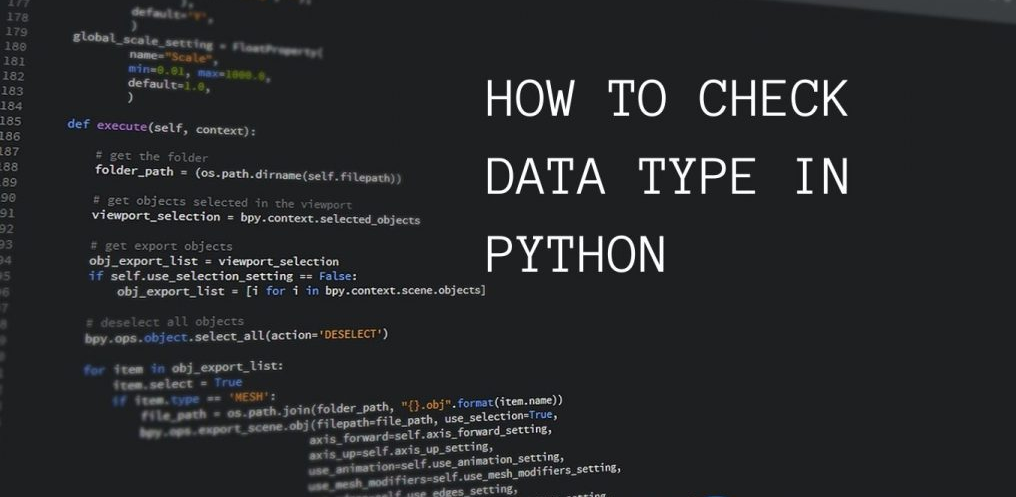
How to check Data Type in Python?
Now that you know about the built-in data types and the core data types in Python, let’s see how to check data types in Python.
You can get the data type of any object by using the type() function:
In Python, you can check the data type of a variable using the built-in type()
function.
For example:
python x = 10print(type(x)) # Output: <class 'int'> y = 3.14print(type(y)) # Output: <class 'float'> z = "Hello, World!"print(type(z)) # Output: <class 'str'> a = [1, 2, 3, 4] print(type(a)) # Output: <class 'list'> b = (1, 2, 3, 4) print(type(b)) # Output: <class 'tuple'> c = {"key1": "value1", "key2": "value2"} print(type(c)) # Output: <class 'dict'> d = Trueprint(type(d)) # Output: <class 'b
Conclusion:
In conclusion, understanding the different data types in Python is essential for working with data and building efficient programs. From the basic data types like numbers, strings, and booleans, to more complex data structures like lists, tuples, dictionaries, and arrays, each data type serves a specific purpose and has its own set of characteristics. Knowing when to use each data type and how to manipulate them is key to becoming a skilled Python programmer.