Object Oriented Programming in Python
Object-oriented programming (OOP) is a method of structuring a program by bundling related properties and behaviors into individual objects. In this tutorial, you’ll learn the basics of object oriented programming in Python.
Conceptually, objects are like the components of a system. Think of a program as a factory assembly line of sorts. At each step of the assembly line a system component processes some material, ultimately transforming raw material into a finished product.
An object contains data, like the raw or preprocessed materials at each step on an assembly line, and behavior, like the action each assembly line component performs.
In this tutorial, you’ll learn how to:
- Introduction to Object Oriented Programming in Python
- Difference between object and procedural oriented programming
- What are Classes and Objects?
- Object-Oriented Programming methodologies:
- Inheritance
- Polymorphism
- Encapsulation
- Abstraction
- Object-oriented vs. Procedure-oriented Programming languages
Introduction to Object Oriented Programming in Python
Object-oriented programming (OOP) is a programming paradigm that is based on the concept of “objects”, which can contain data and code that manipulates the data. Python is an object-oriented programming language, which means that its design is based on the OOP principles. In Python, objects are instances of classes, and classes define the structure and behavior of objects.
In OOP, classes encapsulate data and the methods that operate on that data, and objects are instances of classes. This encapsulation helps to keep the data safe from accidental modification, and it provides a clear and concise way to define and access an object’s behavior.
A Python class is defined using the “class” keyword, followed by the class name and a colon. The class definition usually includes the “init” method, which is the constructor for the class. This method is called when an object is created from the class and it is used to initialize the object’s attributes. The class can also include other methods that define the object’s behavior.
Creating an object in Python is done by calling the class as if it were a function. The new object is then assigned to a variable, which is used to access the object’s attributes and behavior.
Example:
class Dog: def __init__(self, name, breed): self.name = name self.breed = breed def bark(self): print("Woof!") dog = Dog("Fido", "Labrador") print(dog.name) # Output: "Fido" dog.bark() # Output: "Woof!
In the example above, we have defined a class “Dog” with a constructor that takes two arguments, “name” and “breed”. We have also defined a method “bark” that prints “Woof!” when called. We then created an object “dog” from the class, and accessed its name and called its bark method.
Difference between object and procedural oriented programming
Object-oriented programming (OOP) is a programming paradigm based on the concept of “objects”, which can contain data and code that manipulates that data. It is based on the ideas of encapsulation, inheritance, and polymorphism.
Procedural programming, on the other hand, is a programming paradigm that is based on a sequence of procedures or functions. It focuses on dividing the overall solution into smaller, manageable functions. The primary unit in procedural programming is a procedure, which can take input and produce output.
In summary, OOP focuses on objects and their interactions, while procedural programming focuses on procedures and functions.
What are Classes and Objects?
Classes and objects are fundamental concepts in object-oriented programming (OOP). A class is a blueprint or a blueprint that defines the characteristics and behaviors (methods) of a certain type of object. An object is an instance of a class, created at runtime. It contains the data (attributes) that define the object and the behavior (methods) that can be performed on the object. Classes and objects provide a way to model real-world objects and their behaviors in a program, making it easier to write and understand complex software systems.
Object-Oriented Programming methodologies:
Like other general-purpose programming languages, Python is also an object-oriented language since its beginning. It allows us to develop applications using an Object-Oriented approach. In Python, we can easily create and use classes and objects.
An object-oriented paradigm is to design the program using classes and objects. The object is related to real-word entities such as book, house, pencil, etc. The oops concept focuses on writing the reusable code. It is a widespread technique to solve the problem by creating objects.
Major principles of object-oriented programming system are shown in below Picture .
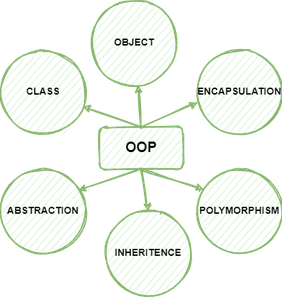
Class
The class can be defined as a collection of objects. It is a logical entity that has some specific attributes and methods. For example: if you have an employee class, then it should contain an attribute and method, i.e. an email id, name, age, salary, etc.
Syntax
- class ClassName: <statement-1> . . <statement-N>
Object
The object is an entity that has state and behavior. It may be any real-world object like the mouse, keyboard, chair, table, pen, etc.
Everything in Python is an object, and almost everything has attributes and methods. All functions have a built-in attribute __doc__, which returns the docstring defined in the function source code.
When we define a class, it needs to create an object to allocate the memory. Consider the following example.
Example:
class car: def __init__(self,modelname, year): self.modelname = modelname self.year = year def display(self): print(self.modelname,self.year) c1 = car("Toyota", 2016) c1.display()
Output:
Toyota 2016
In the above example, we have created the class named car, and it has two attributes modelname and year. We have created a c1 object to access the class attribute. The c1 object will allocate memory for these values. We will learn more about class and object in the next tutorial.
Method
The method is a function that is associated with an object. In Python, a method is not unique to class instances. Any object type can have methods.
Mainly Object-Oriented Programming methodologies deal with the following concepts.
- Inheritance
- Polymorphism
- Encapsulation
- Abstraction
Inheritance
Ever heard of this dialogue from relatives “you look exactly like your father/mother” the reason behind this is called ‘inheritance’. From the Programming aspect, It generally means “inheriting or transfer of characteristics from parent to child class without any modification”. The new class is called the derived/child class and the one from which it is derived is called a parent/base class.
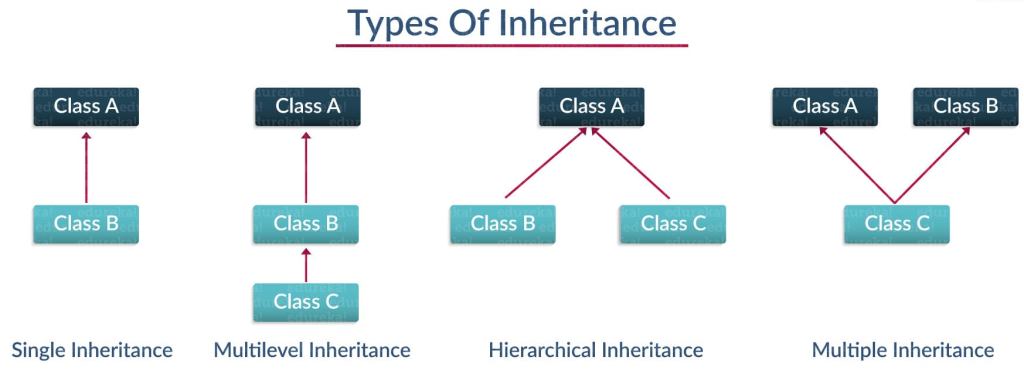
Single Inheritance:
Single-level inheritance enables a derived class to inherit characteristics from a single-parent class.
Multilevel Inheritance:
Multi-level inheritance enables a derived class to inherit properties from an immediate parent class which in turn inherits properties from his parent class.
Hierarchical Inheritance:
Hierarchical level inheritance enables more than one derived class to inherit properties from a parent class.
Multiple Inheritance:
Multiple level inheritance enables one derived class to inherit properties from more than one base class.
Example: Inheritance in Python
# Python code to demonstrate how parent constructors # are called. # parent class class Person(object): # __init__ is known as the constructor def __init__(self, name, idnumber): self.name = name self.idnumber = idnumber def display(self): print(self.name) print(self.idnumber) def details(self): print("My name is {}".format(self.name)) print("IdNumber: {}".format(self.idnumber)) # child class class Employee(Person): def __init__(self, name, idnumber, salary, post): self.salary = salary self.post = post # invoking the __init__ of the parent class Person.__init__(self, name, idnumber) def details(self): print("My name is {}".format(self.name)) print("IdNumber: {}".format(self.idnumber)) print("Post: {}".format(self.post)) # creation of an object variable or an instance a = Employee('Rahul', 886012, 200000, "Intern") # calling a function of the class Person using # its instance a.display() a.details()
Output:
Rahul 886012 My name is Rahul IdNumber: 886012 Post: Intern
In the above Example, we have created two classes i.e. Person (parent class) and Employee (Child Class). The Employee class inherits from the Person class. We can use the methods of the person class through employee class as seen in the display function in the above code. A child class can also modify the behavior of the parent class as seen through the details() method.
Polymorphism
You all must have used GPS for navigating the route, Isn’t it amazing how many different routes you come across for the same destination depending on the traffic, from a programming point of view this is called ‘polymorphism’. It is one such OOP methodology where one task can be performed in several different ways. To put it in simple words, it is a property of an object which allows it to take multiple forms.
Polymorphism is of two types:
- Compile-time Polymorphism
- Run-time Polymorphism
Compile-time Polymorphism:
A compile-time polymorphism also called as static polymorphism which gets resolved during the compilation time of the program. One common example is “method overloading”. Let me show you a quick example of the same.
Example:
class employee1(): def name(self): print("Harshit is his name") def salary(self): print("3000 is his salary") def age(self): print("22 is his age") class employee2(): def name(self): print("Rahul is his name") def salary(self): print("4000 is his salary") def age(self): print("23 is his age") def func(obj)://Method Overloading obj.name() obj.salary() obj.age() obj_emp1 = employee1() obj_emp2 = employee2() func(obj_emp1) func(obj_emp2)
Output:
Harshit is his name 3000 is his salary 22 is his age Rahul is his name 4000 is his salary 23 is his age
Explanation:
- In the above Program, I have created two classes ’employee1′ and ’employee2′ and created functions for both ‘name’, ‘salary’ and ‘age’ and printed the value of the same without taking it from the user.
- Now, welcome to the main part where I have created a function with ‘obj’ as the parameter and calling all the three functions i.e. ‘name’, ‘age’ and ‘salary’.
- Later, instantiated objects emp_1 and emp_2 against the two classes and simply called the function. Such type is called method overloading which allows a class to have more than one method under the same name.
Run-time Polymorphism:
A run-time Polymorphism is also, called as dynamic polymorphism where it gets resolved into the run time. One common example of Run-time polymorphism is “method overriding”. Let me show you through an example for a better understanding.
Example:
class employee(): def __init__(self,name,age,id,salary): self.name = name self.age = age self.salary = salary self.id = id def earn(self): pass class childemployee1(employee): def earn(self)://Run-time polymorphism print("no money") class childemployee2(employee): def earn(self): print("has money") c = childemployee1 c.earn(employee) d = childemployee2 d.earn(employee)
Output:
no money, has money
Explanation: In the above example, I have created two classes ‘childemployee1’ and ‘childemployee2’ which are derived from the same base class ‘employee’.Here’s the catch one did not receive money whereas the other one gets. Now the real question is how did this happen? Well, here if you look closely I created an empty function and used Pass ( a statement which is used when you do not want to execute any command or code). Now, Under the two derived classes, I used the same empty function and made use of the print statement as ‘no money’ and ‘has money’.Lastly, created two objects and called the function.
Moving on to the next Object-Oriented Programming Python methodology, I’ll talk about encapsulation.
Encapsulation
In a raw form, encapsulation basically means binding up of data in a single class. Python does not have any private keyword, unlike Java. A class shouldn’t be directly accessed but be prefixed in an underscore.
Let me show you an example for a better understanding.
class employee(): def __init__(self): self.__maxearn = 1000000 def earn(self): print("earning is:{}".format(self.__maxearn)) def setmaxearn(self,earn)://setter method used for accesing private class self.__maxearn = earn emp1 = employee() emp1.earn() emp1.__maxearn = 10000 emp1.earn() emp1.setmaxearn(10000) emp1.earn()
Output:
earning is:1000000,earning is:1000000,earning is:10000
Explanation: Making Use of the setter method provides indirect access to the private class method. Here I have defined a class employee and used a (__maxearn) which is the setter method used here to store the maximum earning of the employee, and a setter function setmaxearn() which is taking price as the parameter.
Data Abstraction
Data abstraction and encapsulation both are often used as synonyms. Both are nearly synonyms because data abstraction is achieved through encapsulation.
Abstraction is used to hide internal details and show only functionalities. Abstracting something means to give names to things so that the name captures the core of what a function or a whole program does.
An abstract class cannot be instantiated which simply means you cannot create objects for this type of class. It can only be used for inheriting the functionalities.
Example:
from abc import ABC,abstractmethod class employee(ABC): def emp_id(self,id,name,age,salary): //Abstraction pass class childemployee1(employee): def emp_id(self,id): print("emp_id is 12345") emp1 = childemployee1() emp1.emp_id(id)
Output: emp_id is 12345
Explanation: As you can see in the above example, we have imported an abstract method and the rest of the program has a parent and a derived class. An object is instantiated for the ‘childemployee’ base class and functionality of abstract is being used.
Object-oriented vs. Procedure-oriented Programming languages
The difference between object-oriented and procedure-oriented programming is given below:
Index | Object-oriented Programming | Procedural Programming |
1. | Object-oriented programming is the problem-solving approach and used where computation is done by using objects. | Procedural programming uses a list of instructions to do computation step by step. |
2. | It makes the development and maintenance easier. | In procedural programming, It is not easy to maintain the codes when the project becomes lengthy. |
3. | It simulates the real world entity. So real-world problems can be easily solved through oops. | It doesn’t simulate the real world. It works on step by step instructions divided into small parts called functions. |
4. | It provides data hiding. So it is more secure than procedural languages. You cannot access private data from anywhere. | Procedural language doesn’t provide any proper way for data binding, so it is less secure. |
5. | Example of object-oriented programming languages is C++, Java, .Net, Python, C#, etc. | Example of procedural languages are: C, Fortran, Pascal, VB etc. |
FAQ’s
Q1. What is OOP in Python?
OOP is a programming paradigm that focuses on using objects and classes to design applications and computer programs. In Python, everything is an object, and you can use classes to create your own objects and encapsulate data and behavior.
Q2. What is the init method in Python OOP?
The __init__
method is a special method in Python classes, also known as the constructor. It is called automatically when an object is created from a class and is used to initialize the object’s attributes.
Q3. What is an object in Python?
An object is an instance of a class, created from a class blueprint. Objects have their own attributes and behaviors, as defined in the class definition.