Need To Know About Python Dictionary
In this article, we will start from the basics and dive deep into everything that a dictionary can do in Python
Dictionaries in Python, or hash tables in other programming languages, are one of the most efficient data structures for storing and retrieving values. They store each value against a key making a key-value pair. In this article, we will be learning everything that one should know about dictionaries to get started in Python.
Here’s a list of topics we will be covering regarding dictionaries today:
- Intro to Dictionaries
- How to define a dictionary
- How to access a dictionary
- How to assign values in a dictionary
- Methods on Dictionaries
- Restrictions on Dictionary Keys/Values
- Conclusion
Intro to Dictionaries:
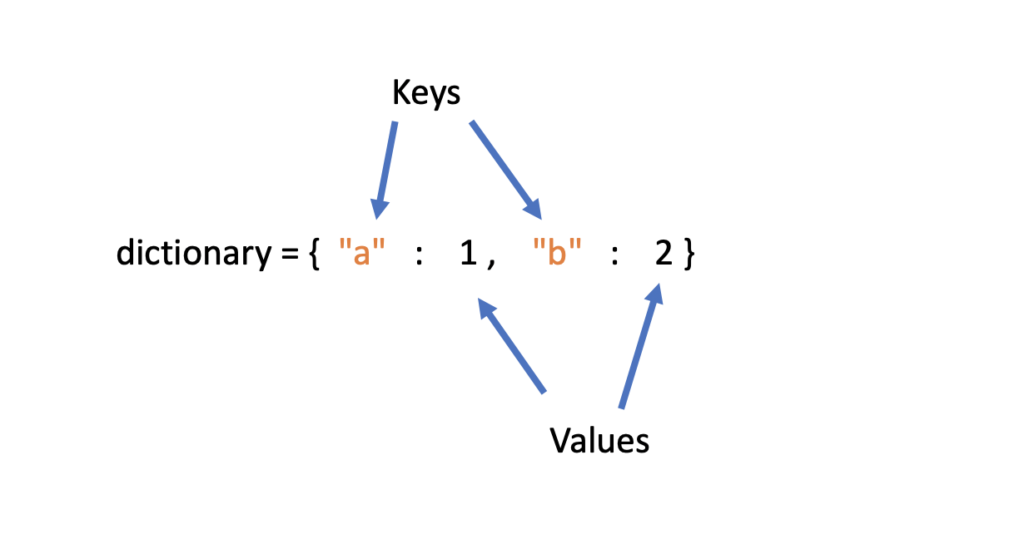
A dictionary in Python is a collection of key-value pairs, where each key is unique and associated with a value. Dictionaries are unordered and mutable, meaning you can change the values stored in a dictionary. They are defined using curly braces {} and the key-value pairs are separated by a colon :, for example:
d = {'key1': 'value1', 'key2': 'value2'}
You can access the values stored in a dictionary by using the keys as indices, for example:
print(d['key1']) # Output: 'value1'
If you try to access a key that doesn’t exist in the dictionary, a KeyError will be raised. You can use the get method to avoid this, which returns None if the key is not found:
print(d.get('key3')) # Output: None
You can add, modify, or delete items in a dictionary by using the assignment operator =
and the square brackets []. For example:
d['key3'] = 'value3' # add an item d['key2'] = 'new value2' # modify an itemdel d['key1'] # delete an item
Dictionaries are very useful for storing and organizing data in a flexible way, and are widely used in many applications.
How to Define a Dictionary:
A dictionary in Python is defined using curly braces {}
and key-value pairs separated by a colon :
.
Here is an example:
# Define a dictionary person = {'name': 'John Doe', 'age': 30, 'gender': 'male'} # Print the dictionaryprint(person)
This would output:
{'name': 'John Doe', 'age': 30, 'gender': 'male'}
In this example, ‘name’, ‘age’, and ‘gender’ are keys and ‘John Doe’, 30, and ‘male’ are their associated values. You can access the values stored in a dictionary by using the keys as indices, for example:
# Access a value using the keyprint (person['name']) # Output: 'John Doe'
How to access the Dictionary values:
To access the values stored in a dictionary, you can use the keys as indices. For example:
# Define a dictionary person = {'name': 'John Doe', 'age': 30, 'gender': 'male'} # Access a value using the key print(person['name']) # Output: 'John Doe
You can also use the get method to access the values. This method is useful when you want to avoid a KeyError in case the key is not found in the dictionary:
# Access a value using the get method print(person.get('email')) # Output: None
You can specify a default value to return if the key is not found using the get
method:
# Access a value using the get method with a default value print(person.get('email', 'Not found')) # Output: 'Not found'
How to assign values in a dictionary:
We can assign new values to a key or even update the values of existing keys in a dictionary by simply accessing the dictionary with the key and setting it to the desired value like this:
football_players_club['Luis Suarez'] = 'Athletico Madrid' football_players_club['Harry Kane'] = 'Manchester City'
Methods on Dictionaries:
Dictionaries in Python have several built-in methods that you can use to perform various operations. Here are some of the most commonly used methods:
- clear: Removes all items from the dictionary.
person = {'name': 'John Doe', 'age': 30, 'gender': 'male'} person.clear() print(person) # Output: {}
- copy: Returns a shallow copy of the dictionary.
person = {'name': 'John Doe', 'age': 30, 'gender': 'male'} person_copy = person.copy() print(person_copy) # Output: {'name': 'John Doe', 'age': 30, 'gender': 'male'}
- get: Returns the value of the specified key. If the key is not found, it returns None (or a default value if specified).
person = {'name': 'John Doe', 'age': 30, 'gender': 'male'} print(person.get('name')) # Output: 'John Doe'
- items: Returns a list of all the key-value pairs in the dictionary.
person = {'name': 'John Doe', 'age': 30, 'gender': 'male'} print(person.items()) # Output: [('name', 'John Doe'), ('age', 30), ('gender', 'male')]
- keys: Returns a list of all the keys in the dictionary.
person = {'name': 'John Doe', 'age': 30, 'gender': 'male'} print(person.keys()) # Output: ['name', 'age', 'gender']
- pop: Removes the specified key-value pair and returns its value.
person = {'name': 'John Doe', 'age': 30, 'gender': 'male'} print(person.pop('name')) # Output: 'John Doe'
- popitem: Removes and returns an arbitrary key-value pair from the dictionary.
person = {'name': 'John Doe', 'age': 30, 'gender': 'male'} print(person.popitem()) # Output: ('gender', 'male')
- update: Adds or updates key-value pairs in the dictionary.
person = {'name': 'John Doe', 'age': 30, 'gender': 'male'} person.update({'email': '[email protected]'}) print(person) # Output: {'name': 'John Doe', 'age': 30, 'gender': 'male', 'email': '[email protected]'}
- values: Returns a list of all the values in the dictionary.
person = {'name': 'John Doe', 'age': 30, 'gender': 'male'} print(person.values()) # Output: ['John Doe', 30, 'male']
Restrictions on Dictionary Keys/Values:
Dictionaries in Python have a few restrictions on the data types that can be used as keys and values:
- Keys:
- Must be hashable, i.e., they must have a hash value that never changes during their lifetime.
- Some common hashable data types include numbers (integers, floating-point numbers, etc.), strings, and tuples that contain only hashable elements.
- Unhashable data types like lists, dictionaries, and sets cannot be used as keys.
- Values:
- Can be of any data type, including lists, dictionaries, and other complex data structures.
- Key-Value Pairs:
- Each key in a dictionary must be unique. If you try to add a duplicate key to the dictionary, the new value will overwrite the previous one.
Here’s an example that demonstrates some of the restrictions on dictionary keys and values
# A list is an unhashable type and cannot be used as a key # person = {['name']: 'John Doe'} # This will raise a TypeError # A tuple can be used as a key because it is hashable person = {('name',): 'John Doe'} print(person) # Output: {('name',): 'John Doe'} # A dictionary value can be mutable, like a list person = {'name': 'John Doe', 'details': ['male', 30]} print(person) # Output: {'name': 'John Doe', 'details': ['male', 30]} # If a key is duplicated, the new value will overwrite the old value person = {'name': 'John Doe', 'name': 'Jane Doe'} print(person) # Output: {'name': '
Conclusion:
Dictionaries are an essential data structure in Python that allows you to store key-value pairs in an organized manner. They are versatile, fast, and can be used to store and retrieve data in a variety of scenarios.
You can create dictionaries using the {} syntax or by using the dict constructor. You can access, modify, and delete values in dictionaries using the key associated with that value. You can also perform various operations on dictionaries using built-in methods such as keys, values, items, get, and more.
It’s important to keep in mind the restrictions on dictionary keys and values, such as the requirement that keys must be hashable, and the fact that duplicate keys overwrite the previous value.
Overall, dictionaries are a powerful and useful tool in Python, and understanding how to use them effectively can greatly improve your coding skills.
FAQ’s
Q1. What is a dictionary in Python?
A dictionary is a built-in data type in Python that stores key-value pairs.
Q2. How to create a dictionary in Python?
You can create a dictionary by enclosing a comma-separated list of key-value pairs within curly braces {} or by using the dict() constructor.
Q3. How to add an element to a dictionary?
You can add a new element to a dictionary by specifying a new key in square brackets [] and assigning a value to it.