Know about Substring in Python
A substring in python is considered as a continous portion of a string. The complete string is also considered as a substring. We can generate a substring in python in several ways which we obviously discussed in the article. But one of the most widely used method for generation of substring in python is with the help of the slice operation.
Topics that Hear going to cover in this blog:
- What is a substring in Python?
- Slicing Strings in Python
- Validate if a Substring is Present within another String
What is Substring in Python ?
A substring is a continous segment of character within a string. In simpler words, Substring is a continous portion of a string. The Python string specifies several techniques for constructing a substring, checking if it includes a substring, and much more.
Slicing Strings in Python
- Slicing using start-index and end-index
s= s[ startIndex : endIndex]
Please Note: index start from 0 in a string.
- Slicing using start-index without end-index
s= s[ startIndex :]
Example
In this example we are slicing the original string by passing both start and end value.
originalString = 'datacademy' subString = originalString[1:7] print('originalString: ', originalString) print('subString: ', subString
Output
originalString: datacademy subString: atacad
Explanation
- Firstly, we have created an original string.
- After that, we used slicing operator in which we pass startIndex and the endIndex.
- Finally, we got the output where the character at startIndex is included while character at endIndex is excluded.
Using start index without end index ([start:])
When we specify the start index but don’t specify the end index, in the slicing operator then this will generate a substring which includes the starting index and create substring till the end of the string. Let’s check the example of this case.
Example
In this example we are slicing the original string by only passing start value.
originalString = 'pythonArticles'subString = originalString[5:] print('originalString: ', originalString) print('subString: ', subString)
Output
originalString: pythonArticles subString: nArticles
Explanation
- Firstly, we have created an original string.
- After that, we used slicing operator in which we pass startIndex.
- Finally, we got the output where the character at startIndex is included and the substring is generated till the end of the string.
Using end index without start index ([])
When we specify the end index but don’t specify the start index, in the slicing operator then this will generate a substring from the start of the string and end the substring where the end index is specified(which is excluded from the substring). Let’s check the example of this case.
Example
In this example we are slicing the original string by only passing end value.
originalString = 'datacademy' subString = originalString[:10] print('originalString: ', originalString) print('subString: ', subString)
Output
originalString: datacademy subString: datacademy
Explanation
- Firstly, we have created an original string.
- After that, we used slicing operator in which we pass the endIndex.
- Finally, we got the output where the substring starts from the beginning of the string and ends at the position where endIndex is specified.
Using complete string ([:])
When we don’t specify the start index and the end index, in the slicing operator then this will generate a substring beginning to the end of the string. In other words, it will slice the complete string. Let’s check the example of this case.
Example
In this example we are slicing the original string by passing no value in the slicing operator.
originalString = 'pythonArticles' subString = originalString[:] print('originalString: ', originalString) print('subString: ', subString)
Output
originalString: pythonArticles subString: pythonArticles
Explanation
- Firstly, we have created an original string.
- After that, we used slicing operator in which don’t pass any of the parameter.
- Finally, we got the output where the whole string is sliced.
Using single character from a string ([index])
When we just specify the single index , in the slicing operator then this will return a single character which is present at that particular index. Let’s check the example of this case.
Example
In this example we are slicing the original string by only passing a single index position.
originalString = 'Datacademy' subString = originalString[5] print('originalString: ', originalString) print('subString: ', subString)
Output
originalString: Datacademy subString: a
Explanation
- Firstly, we have created an original string.
- After that, we used slicing operator in which we pass a single index.
- Finally, we got the output where the character at the specified index is printed.
Using start index, end index and step (start : end : step)
When we specify the start index, end index and the steps then this will generate a substring from start index (inclusive) to the end index (exclusive) where every character is at an interval of steps which are passed in the parameter. By default value of steps is 1.
Example
In this example we are slicing the original string by passing start, end and the steps value.
originalString = 'pythonArticles'subString = originalString[2:12:2] print('originalString: ', originalString) print('subString: ', subString)
Output
originalString: pythonArticles subString: toAtc
Explanation
- Firstly, we have created an original string.
- After that, we used slicing operator in which we pass startIndex and the endIndex and the step.
- Finally, we got the output where the character at startIndex is included while character at endIndex is excluded and every character is at an interval of steps which are pass in the parameter.
Using negative index ([-index])
As we know, python also support -ve indexing. The letters of the string when traversed from right to left are indexed with negative numbers.
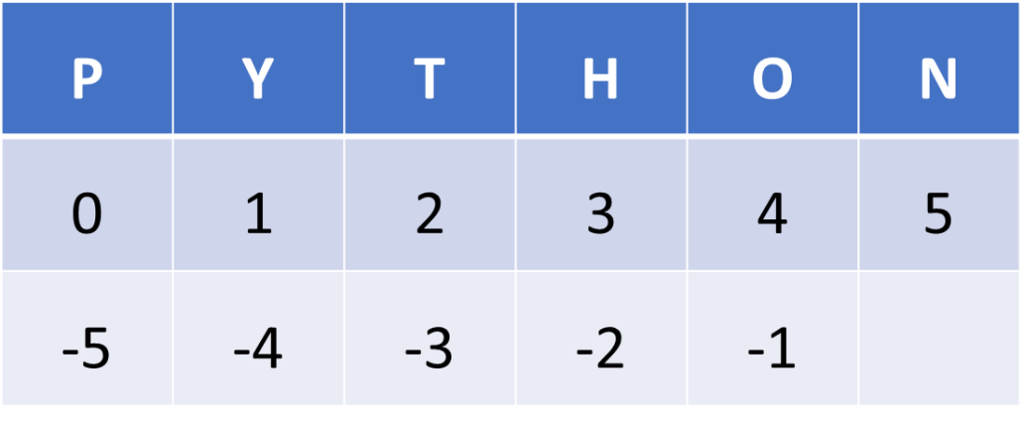
The index in string starts from 0 to 5, alternatively we can use a negative index as well.
# Slicing or substring a string using negative index. s= 'PYTHON' s[0:-3]
Output:
‘PYT’
The same string can be sliced using a positive index as well
- # Slicing or substring a string using positive index. s= ‘PYTHON’ s[0:3]
Output:
‘PYT’
Retrieving all substrings using list comprehension
# Get all substrings of string using list comprehension and string slicing s= 'PYTHON' str=[s[i: j] for i in range(len(s)) for j in range(i + 1, len(s) + 1)] print (str)
Output:
['P', 'PY', 'PYT', 'PYTH', 'PYTHO', 'PYTHON', 'Y', 'YT', 'YTH', 'YTHO', 'YTHON', 'T', 'TH', 'THO', 'THON', 'H', 'HO', 'HON', 'O', 'ON', 'N']
Retrieving all substrings using itertools.combinations() method
- # Get all substrings of string using list comprehension and string slicing s= ‘PYTHON’ str=[s[i: j] for i in range(len(s)) print (str)
Output:
['P', 'PY', 'PYT', 'PYTH', 'PYTHO', 'PYTHON', 'Y', 'YT', 'YTH', 'YTHO', 'YTHON', 'T', 'TH', 'THO', 'THON', 'H', 'HO', 'HON', 'O', 'ON', 'N']
Validate if a substring is present within another string
- Using in operator
# Check if string is present within another string using in operator s= 'This is to demonstrated substring functionality in python.' print("This" in s) print("Not present" in s)
Output:
True False
In operator will return true if a substring is present within another string else will return false.
- Using the find method
# Check if string is present within another string using find method s= 'This is to demonstrated substring functionality in python.' print(s.find("demonstrate")) print(s.find("Not present"))
Output:
11 -1
Add Your Heading Text Here
Q1. How do I extract a substring from a string in Python?
You can extract a substring from a string by slicing the string. For example:
string = “Hello World”
substring = string[0:5] # start at index 0 and end at index 5 (not included)
print(substring) # Output: “Hello”
Q2. How do I find the index of a substring in a string in Python?
You can use the .find()
method to find the index of a substring in a string. For example:
string = “Hello World”
index = string.find(“World”)
print(index) # Output: 6
Q3. How do I check if a substring is present in a string in Python?
You can use the in
operator to check if a substring is present in a string. For example:
string = “Hello World”
is_present = “World” in string
print(is_present) # Output: True