Java Collections Interview Questions
Generic – Java Collections Interview Questions
1. What are the advantages of the Collection Framework in Java?
The Java Collections Framework provides the following advantages:
- It provides a standard way of handling collections in Java, which makes it easier to work with multiple types of collections.
- It provides a set of interfaces and classes that can be used to implement various types of collections, such as lists, sets, and maps.
- It provides a set of algorithms that can be used to perform common operations on collections, such as searching, sorting, and shuffling.
- It provides a set of classes that implement the collections interfaces, such as ArrayList, HashSet, and HashMap, which can be used to store and manipulate data.
- It allows collections to be manipulated independently of the details of their representation, which makes it easier to change the implementation of a collection without affecting the code that uses it.
- It provides support for concurrent access to collections, which makes it easier to write thread-safe code.
- It provides support for generic types, which allows collections to be used with any type of object, making the code more reusable and flexible.
2. What do you understand by Collection Framework in Java?
The Java Collection
framework provides an architecture to store and manage a group of objects. It permits the developers to access prepackaged data structures as well as algorithms to manipulate data. The collection framework includes the following:
- Interfaces
- Classes
- Algorithm
All these classes and interfaces support various operations such as Searching, Sorting, Insertion, Manipulation, and Deletion which makes the data manipulation really easy and quick.
3. Describe the Collection hierarchy in Java.
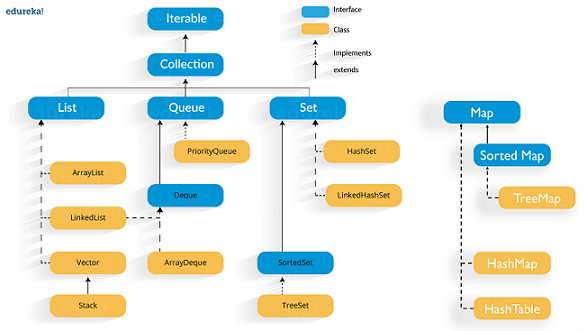
4. List down the primary interfaces provided by Java Collections Framework?
Below are the major interfaces
provided by the Collection Framework:
- Collection Interface: java.util.Collection is the root of the Java Collection framework and most of the collections in Java are inherited from this interface.
1 | public interface Collection<E>extends Iterable |
- List Interface: java.util.List is an extended form of an array that contains ordered elements and may include duplicates. It supports the index-based search, but elements can be easily inserted irrespective of the position. The List interface is implemented by various classes such as ArrayList, LinkedList, Vector, etc.
1 | public interface List<E> extends Collection<E> |
- Set Interface: java.util.Set refers to a collection class that cannot contain duplicate elements. Since it doesn’t define an order for the elements, the index-based search is not supported. It is majorly used as a mathematical set abstraction model. The Set interface is implemented by various classes such as HashSet, TreeSetand LinkedHashSet.
1 | public interface Set<E> extends Collection<E> |
- Queue Interface: java.util.Queue in Java follows a FIFO approach i.e. it orders the elements in First In First Out manner. Elements in Queue will be added from the rear end while removed from the front.
1 | public interface Queue<E> extends Collection<E> |
- Map Interface: java.util.Map is a two-dimensional data structure in Java that is used to store the data in the form of a Key-Value pair. The key here is the unique hashcode and value represent the element. Map in Java is another form of the Java Set but can’t contain duplicate elements.
5. Why Collection doesn’t extend the Cloneable and Serializable interfaces?
The Collection interface does not extend the Cloneable and Serializable interfaces because these interfaces are not necessarily applicable to all collections.
The Cloneable interface is used to mark a class as being eligible for cloning, which means that a copy of the object can be created using the clone()
method. However, it is not always possible or appropriate to create a deep copy of a collection and its elements. Therefore, the Collection interface does not extend the Cloneable interface.
The Serializable interface is used to mark a class as being serializable, which means that it can be converted to a sequence of bytes and written to a file or transmitted over a network. However, not all collections can be serialized, and the serialization process may not be the same for all collections. Therefore, the Collection interface does not extend the Serializable interface.
Instead of extending these interfaces, the Collection interface defines methods for creating a copy of a collection and for serializing and deserializing a collection. This allows individual collection implementations to decide how to implement these operations, if at all.
6. List down the major advantages of the Generic Collection.
The major advantages of the generic collection are:
- Type safety: The generic collection ensures that the elements stored in a collection are of a specific type. This prevents the risk of ClassCastException at runtime when trying to retrieve an element from a collection and casting it to an inappropriate type.
- Improved readability: The generic collection makes the code more readable by specifying the type of the elements stored in a collection. This helps to reduce confusion and makes it easier to understand the code.
- Enhanced efficiency: The generic collection allows the compiler to generate more efficient code by eliminating the need for type casting and type checking at runtime.
- Increased reusability: The generic collection allows the same collection to be used with different types of elements, which increases the reusability of the collection.
- Enhanced type inference: The type inference feature of the Java compiler allows the type of the elements in a collection to be inferred from the context, which simplifies the code and makes it more concise.
- Improved error detection: The generic collection allows the compiler to detect errors at compile-time rather than at runtime, which makes it easier to identify and fix errors in the code.
7. What is the main benefit of using the Properties file?
The main advantage of using the properties file in Java is that in case the values in the properties file is changed it will be automatically reflected without having to recompile the java class. Thus it is mainly used to store information which is liable to change such as username and passwords. This makes the management of the application easy and efficient. Below is an example of the same:
1234567891011 | import java.util.*; import java.io.*; public class PropertiesDemo{ public static void main(String[] args)throws Exception{ FileReader fr=new FileReader( "db.properties" ); Properties pr=new Properties(); pr.load(fr); System.out.println(pr.getProperty( "user" )); System.out.println(pr.getProperty( "password" )); } } |
8. What do you understand by Iterator in the Java Collection Framework?
Iterator in Java is an interface of the Collection framework present in java.util package. It is a Cursor in Java which is used to iterate a collection of objects. Below are a few other major functionalities provided by the Iterator interface:
- Traverse a collection object elements one by one
- Known as Universal Java Cursor as it is applicable for all the classes of the Collection framework
- Supports READ and REMOVE Operations.
- Iterator method names are easy to implement
9. What is the need for overriding equals() method in Java?
The equals()
method is used to determine whether two objects are equal. In Java, the equals()
method is defined in the Object class and is used to compare the identity of two objects. By default, the equals()
method returns true if and only if the two objects being compared are the same object, which is determined by comparing their memory addresses.
However, in many cases, it is necessary to override the equals()
method to provide a different definition of equality. For example, two objects may be considered equal if they have the same values for their fields, even if they are not the same object.
Overriding the equals()
method allows the programmer to customize the definition of equality for a particular class. This is useful when creating classes that represent complex data structures, such as collections, and when creating classes that represent entities in a domain model.
In addition to the equals()
method, it is also necessary to override the hashCode()
method when overriding the equals()
method. The hashCode()
method is used to generate a numerical value that represents an object, and it is used by various Java classes, such as HashMap and HashSet, to store and retrieve objects efficiently. Overriding the hashCode()
method ensures that two objects that are equal according to the equals()
method have the same hash code.
10. How the Collection objects are sorted in Java?
Sorting in Java Collections is implemented via Comparable and Comparator interfaces. When Collections.sort() method is used the elements get sorted based on the natural order that is specified in the compareTo() method. On the other hand when Collections.sort(Comparator) method is used it sorts the objects based on compare() method of the Comparator interface.
List – Java Collections Interview Questions
11. What is the use of the List interface?
The List interface is a part of the Java Collections Framework and represents an ordered collection of elements. It extends the Collection interface and adds additional methods for manipulating the elements in a list.
The main uses of the List interface are:
- Storing a sequence of elements: The List interface allows you to store a series of elements in a specific order. You can add elements to the list, remove elements from the list, and access the elements by their position in the list.
- Performing operations on elements: The List interface provides methods for inserting and deleting elements, as well as searching for specific elements. It also provides methods for sorting and shuffling the elements in the list.
- Implementing stack and queue data structures: The List interface can be used to implement stack and queue data structures by using its methods to add and remove elements at the appropriate end of the list.
- Storing data in a more flexible way: The List interface allows you to store data in a more flexible way than an array, as it allows you to add and remove elements at any position in the list, and it automatically adjusts the size of the list as needed.
- Providing support for generic types: The List interface supports generic types, which allows you to specify the type of the elements stored in the list. This helps to ensure type safety and improves the readability of the code.
12. What is ArrayList in Java?
ArrayList is a class in the Java Collections Framework that implements the List interface. It is an ordered collection of elements that allows duplicate elements and provides methods for inserting and deleting elements, as well as searching for specific elements. ArrayList is implemented as an array, which means that it has a fixed size that can be changed as needed.
Syntax:
Copy codeArrayList<Type> list = new ArrayList<Type>();
Here, Type
is the type of the elements that will be stored in the list. For example, to create an ArrayList of integers, you would use the following syntax:
Copy codeArrayList<Integer> list = new ArrayList<Integer>();
13. How would you convert an ArrayList to Array and an Array to ArrayList?
An Array can be converted into an ArrayList by making use of the asList() method provided by the Array class. It is a static method that accepts List objects as a parameter.
Syntax:
1 | Arrays.asList(item) |
Whereas an ArrayList can be converted into an Array using the toArray() method of the ArrayList class.
Syntax:
1 | List_object.toArray(new String[List_object.size()]) |
14. How will you reverse an List?
ArrayList can be reversed using the reverse() method of the Collections class.
Syntax:
1 | public static void reverse(Collection c) |
For Example:
1234567891011121314 | public class ReversingArrayList { public static void main(String[] args) { List<String> myList = new ArrayList<String>(); myList.add( "AWS" ); myList.add( "Java" ); myList.add( "Python" ); myList .add( "Blockchain" ); System.out.println( "Before Reversing" ); System.out.println(myList.toString()); Collections.reverse(myList); System.out.println( "After Reversing" ); System.out.println(myList); } } |
15. What do you understand by LinkedList in Java? How many types of LinkedList does Java support?
LinkedList in Java is a data structure that contains a sequence of links. Here each link contains a connection to the next link.
Syntax:
1 | Linkedlist object = new Linkedlist(); |
Java LinkedList class uses two types of LinkedList to store the elements:
- Singly Linked List: In a singly LinkedList, each node in this list stores the data of the node and a pointer or reference to the next node in the list.
- Doubly Linked List: In a doubly LinkedList, it has two references, one to the next node and another to the previous node.
16. What is a Vector in Java?
Vectors are similar to arrays, where the elements of the vector object can be accessed via an index into the vector. Vector implements a dynamic array. Also, the vector is not limited to a specific size, it can shrink or grow automatically whenever required. It is similar to ArrayList, but with two differences :
- Vector is synchronized.
- Vector contains many legacy methods that are not part of the collections framework.
Syntax:
1 | Vector object = new Vector(size,increment); |
Queue – Java Collections Interview Questions
17. What are the various methods provided by the Queue interface?
The Queue interface is a part of the Java Collections Framework and represents a queue, which is a first-in, first-out data structure. It extends the Collection interface and adds methods for inserting, retrieving, and deleting elements from the head and tail of the queue.
Here are the various methods provided by the Queue interface:
Method | Description |
---|---|
boolean add(E e) | Inserts the specified element into this queue if it is possible to do so immediately without violating capacity restrictions, returning true upon success and throwing an IllegalStateException if no space is currently available. |
boolean offer(E e) | Inserts the specified element into this queue if it is possible to do so immediately without violating capacity restrictions. |
E remove() | Retrieves and removes the head of this queue. |
E poll() | Retrieves and removes the head of this queue, or returns null if this queue is empty. |
E element() | Retrieves, but does not remove, the head of this queue. |
E peek() | Retrieves, but does not remove, the head of this queue, or returns null if this queue is empty. |
The add()
and offer()
methods are used to insert elements into the queue. The remove()
and poll()
methods are used to remove elements from the head of the queue. The element()
and peek()
methods are used to access the head of the queue without removing it.
Note that the add()
and remove()
methods throw an exception if the operation cannot be performed, while the offer()
and poll()
methods return a special value (null or false) if the operation cannot be performed. This allows the programmer to decide how to handle the case where the queue is full or empty.
The Queue interface also provides other methods, such as size()
, isEmpty()
, and clear()
, which are inherited from the Collection interface. These methods can be used to manipulate the elements in the queue.
18. What do you understand by BlockingQueue?
BlockingQueue interface belongs to the java.util.concurrent package. This interface enhances flow control by activating blocking, in case a thread is trying to dequeue an empty queue or enqueue an already full queue. While working with the BlockingQueue interface in Java, you must remember that it does not accept a null value. In case you try to do that it will instantly throw a NullPointerException. The below figure represents the working of the BlockingQueue interface in Java.
19. What is a priority queue in Java?
A priority queue in Java is an abstract data type similar to a regular queue or stack data structure but has a special feature called priority associated with each element. In this queue, a high priority element is served before a low priority element irrespective of their insertion order. The PriorityQueue is based on the priority heap. The elements of the priority queue are ordered according to the natural ordering, or by a Comparator provided at queue construction time, depending on which constructor is used.
20. What is the Stack class in Java and what are the various methods provided by it?
The Stack class is a class in the Java Collections Framework that represents a stack, which is a last-in, first-out data structure. It is implemented as a subclass of the Vector class and provides methods for pushing and popping elements from the top of the stack, as well as for accessing the elements in the stack.
Here are the various methods provided by the Stack class:
Method | Description |
---|---|
boolean empty() | Tests if this stack is empty. |
E peek() | Looks at the object at the top of this stack without removing it from the stack. |
E pop() | Removes the object at the top of this stack and returns that object as the value of this function. |
E push(E item) | Pushes an item onto the top of this stack. |
int search(Object o) | Returns the 1-based position where an object is on this stack. |
The push()
method is used to insert an element at the top of the stack, and the pop()
method is used to remove and return the element at the top of the stack. The peek()
method is used to access the element at the top of the stack without removing it.
The empty()
method is used to test if the stack is empty, and the search()
method is used to search for an element in the stack and return its position.
Note that the Stack class is not part of the Java Collections Framework, and it is not recommended for use in new code. Instead, it is recommended to use a class that implements the Deque interface, such as ArrayDeque, to implement a stack data structure.
Set – Java Collections Interview Questions
21. What is Set in Java Collections framework and list down its various implementations?
Set is an interface in the Java Collections Framework that represents a collection of elements that does not allow duplicate elements. It extends the Collection interface and adds methods for testing the presence of elements and for determining the number of occurrences of an element.
There are several implementations of the Set interface in the Java Collections Framework, including:
- HashSet: This is a Set implementation that uses a hash table to store the elements. It provides constant-time performance for the basic operations of adding, removing, and testing the presence of an element.
- LinkedHashSet: This is a Set implementation that uses a hash table and a linked list to store the elements. It maintains the elements in the order in which they were added, which allows the elements to be iterated in the order in which they were inserted.
- TreeSet: This is a Set implementation that uses a tree data structure to store the elements. It maintains the elements in ascending order, based on their natural ordering or based on a Comparator provided at the time the set is created.
- EnumSet: This is a Set implementation that is specialized for use with enum types. It is a compact and efficient implementation that is well-suited for storing large numbers of enum values.
- CopyOnWriteArraySet: This is a Set implementation that uses a copy-on-write array to store the elements. It is designed for use in concurrent environments and provides thread-safe access to the elements.
22. What is the HashSet class in Java and how does it store elements?
java.util.HashSet class is a member of the Java collections framework which inherits the AbstractSet class and implements the Set interface. It implicitly implements a hashtable for creating and storing a collection of unique elements. Hashtable is an instance of the HashMap class that uses a hashing mechanism for storing the information within a HashSet. Hashing is the process of converting the informational content into a unique value that is more popularly known as hash code. This hashcode is then used for indexing the data associated with the key. The entire process of transforming the informational key into the hashcode is performed internally.
23. Can you add a null element into a TreeSet or HashSet?
In HashSet, only one null element can be added but in TreeSet it can’t be added as it makes use of NavigableMap for storing the elements. This is because the NavigableMap is a subtype of SortedMap that doesn’t allow null keys. So, in case you try to add null elements to a TreeSet, it will throw a NullPointerException.
24. Explain the emptySet() method in the Collections framework?
The Collections.emptySet() is used to return the empty immutable Set while removing the null elements. The set returned by this method is serializable. Below is the method declaration of emptySet().
Syntax:
1 | public static final <T> Set<T> emptySet() |
25. What is LinkedHashSet in Java Collections Framework?
A java.util.LinkedHashSet is a subclass of the HashSet class and implements the Set interface. It is an ordered version of HashSet which maintains a doubly-linked List across all elements contained within. It preserves the insertion order and contains only unique elements like its parent class.
Syntax:
1 | LinkedHashSet<String> hs = new LinkedHashSet<String>(); |
Java Collections Interview Questions-Map
26. What is Map interface in Java?
The java.util.Map interface in Java stores the elements in the form of keys-values pairs which is designed for faster lookups. Here every key is unique and maps to a single value. These key-value pairs are known as the map entries. This interface includes method signatures for insertion, removal, and retrieval of elements based on a key. With such methods, it’s a perfect tool to use for key-value association mapping such as dictionaries.
27. Why Map doesn’t extend the Collection Interface?
The Map interface in Java follows a key/value pair structure whereas the Collection interface is a collection of objects which are stored in a structured manner with a specified access mechanism. The main reason Map doesn’t extend the Collection interface is that the add(E e) method of the Collection interface doesn’t support the key-value pair like Map interface’s put(K, V) method. It might not extend the Collection interface but still is an integral part of the Java Collections framework.
28. List down the different Collection views provided by the Map interface in the Java Collection framework?
The Map interface provides 3 views of key-value pairs which are:
- key set view
- value set view
- entry set view
All these views can be easily navigated through using the iterators.
29. What is the ConcurrentHashMap in Java and do you implement it?
ConcurrentHashMap is a Java class that implements ConcurrentMap as well as Serializable interfaces. This class is the enhanced version of HashMap as it doesn’t perform well in the multithreaded environment. It has a higher performance rate compared to the HashMap.
Below is a small example demonstrating the implementation of ConcurrentHashMap:
123456789101112131415161718192021222324252627282930313233 | package edureka; import java.util.concurrent.*; public class ConcurrentHashMapDemo { public static void main(String[] args) { ConcurrentHashMap m = new ConcurrentHashMap(); m.put(1, "Welcome" ); m.put(2, "to" ); m.put(3, "Edureka's" ); m.put(4, "Demo" ); System.out.println(m); // Here we cant add Hello because 101 key // is already present in ConcurrentHashMap object m.putIfAbsent(3, "Online" ); System.out.println( "Checking if key 3 is already present in the ConcurrentHashMap object: " + m); // We can remove entry because 101 key // is associated with For value m.remove(1, "Welcome" ); System.out.println( "Removing the value of key 1: " +m); // Now we can add Hello m.putIfAbsent(1, "Hello" ); System.out.println( "Adding new value to the key 1: " +m); // We cant replace Hello with For m.replace(1, "Hello" , "Welcome" ); System.out.println( "Replacing value of key 1 with Welcome: " + m); } } |
30. Can you use any class as a Map key?
Yes, any class can be used as Map Key as long as the following points are considered:
- The class overriding the equals() method must also override the hashCode() method
- The class should adhere to the rules associated with equals() and hashCode() for all instances
- The class field which is not used in the equals() method should not be used in hashCode() method as well
- The best way to use a user-defined key class is by making it immutable. It helps in caching the hashCode() value for better performance. Also if the class is made immutable it will ensure that the hashCode() and equals() are not changing in the future.
Differences – Java Collections Interview Questions
31. Differentiate between Collection and Collections.
Collection | Collections |
java.util.Collection is an interface | java.util.Collections is a class |
Is used to represent a group of objects as a single entity | It is used to define various utility method for collection objects |
It is the root interface of the Collection framework | It is a utility class |
It is used to derive the data structures of the Collection framework | It contains various static methods which help in data structure manipulation |
32. Differentiate between an Array and an ArrayList.
Array | ArrayList |
java.util.Array is a class | java.util.ArrayList is a class |
It is strongly typed | It is loosely types |
Cannot be dynamically resized | Can be dynamically resized |
No need to box and unbox the elements | Needs to box and unbox the elements |
33. Differentiate between Iterable and Iterator.
Iterable | Iterator |
Iterable is an interface | Iterator is an interface |
Belongs to java.lang package | Belongs to java.util package |
Provides one single abstract method called iterator() | Provides two abstract methods called hasNext() and next() |
It is a representation of a series of elements that can be traversed | It represents the object with iteration state |
34. Differentiate between ArrayList and LinkedList.
ArrayList | LinkedList |
Implements dynamic array internally to store elements | Implements doubly linked list internally to store elements |
Manipulation of elements is slower | Manipulation of elements is faster |
Can act only as a List | Can act as a List and a Queue |
Effective for data storage and access | Effective for data manipulation |
35. Differentiate between Comparable and Comparator.
Comparable | Comparator |
Present in java.lang package | Present in java.util package |
Elements are sorted based on natural ordering | Elements are sorted based on user-customized ordering |
Provides a single method called compareTo() | Provides to methods equals() and compare() |
Modifies the actual class | Doesn’t modifies the actual class |
36. Differentiate between List and Set.
List | Set |
An ordered collection of elements | An unordered collection of elements |
Preserves the insertion order | Doesn’t preserves the insertion order |
Duplicate values are allowed | Duplicate values are not allowed |
Any number of null values can be stored | Only one null values can be stored |
ListIterator can be used to traverse the List in any direction | ListIterator cannot be used to traverse a Set |
Contains a legacy class called vector | Doesn’t contains any legacy class |
37. Differentiate between Set and Map.
Set | Map |
Belongs to java.util package | Belongs to java.util package |
Extends the Collection interface | Doesn’t extend the Collection interface |
Duplicate values are not allowed | Duplicate keys are not allowed but duplicate values are |
Only one null values can be stored | Only one null key can be stored but multiple null values are allowed |
Doesn’t maintain any insertion order | Doesn’t maintain any insertion order |
38. Differentiate between List and Map.
List | Map |
Belongs to java.util package | Belongs to java.util package |
Extends the Collection interface | Doesn’t extend the Collection interface |
Duplicate elements are allowed | Duplicate keys are not allowed but duplicate values are |
Multiple null values can be stored | Only one null key can be stored but multiple null values are allowed |
Preserves the insertion order | Doesn’t maintain any insertion order |
Stores elements based on Array Data Structure | Stores data in key-value pairs using various hashing techniques |
39. Differentiate between Queue and Stack.
Queue | Stack |
Based on FIFO (First-In-First-Out) principle | Based on LIFO (Last-In-First-Out) principle |
Insertion and deletion takes place from two opposite ends | Insertion and deletion takes place the same end |
Element insertion is called enqueue | Element insertion is called push |
Element deletion is called dequeue | Element deletion is called pop |
Two pointers are maintained one point to the first element and the other one points the last element on the list | Only one pointer is maintained which points to the top element on the stack |
40. Differentiate between PriorityQueue and TreeSet.
PriorityQueue | TreeSet |
It is a type of Queue | It is based on a Set data structure |
Allows duplicate elements | Doesn’t allows duplicate elements |
Stores the elements based on an additional factor called priority | Stores the elements in a sorted order |
41. Differentiate between the Singly Linked List and Doubly Linked List.
Singly Linked List(SLL) | Doubly Linked List(DLL) |
Contains nodes with a data field and a next node-link field | Contains nodes with a data field, a previous link field, and a next link field |
Can be traversed using the next node-link field only | Can be traversed using the previous node-link or the next node-link |
Occupies less memory space | Occupies more memory space |
Less efficient in providing access to the elements | More efficient in providing access to the elements |
42. Differentiate between Iterator and Enumeration.
Iterator | Enumeration |
Collection element can be removed while traversing it | Can only traverse through the Collection |
Used to traverse most of the classes of the Java Collection framework | Used to traverse the legacy classes such as Vector, HashTable, etc |
Is fail-fast in nature | Is fail-safe in nature |
Is safe and secure | Is not safe and secure |
Provides methods like hasNext(), next() and remove() | Provides methods like hasMoreElements() and nextElement() |
43. Differentiate between HashMap and HashTable.
HashMap | HashTable |
It is non-synchronized in nature | It is synchronized in nature |
Allows only one null key but multiple null values | Doesn’t allow any null key or value |
Has faster processing | has slower processing |
Can be traversed by Iterator | Can be traversed by Iterator and Enumeration |
Inherits AbstractMap class | Inherits Dictionary class |
44. Differentiate between HashSet and HashMap.
HashSet | HasMap |
Based on Set implementation | Based on Map implementation |
Doesn’t allow any duplicate elements | Doesn’t allow any duplicate keys but duplicate values are allowed |
Allows only a single null value | Allows only one null key but any number of null values |
Has slower processing time | Has faster processing time |
Uses HashMap as an underlying data structure | Uses various hashing techniques for data manipulation |
45. Differentiate between Iterator and ListIterator.
Iterator | ListIterator |
Can only perform remove operations on the Collection elements | Can perform add, remove and replace operations the Collection elements |
Can traverse List, Sets and maps | Can traverse only Lists |
Can traverse the Collection in forward direction | Can traverse the collection in any direction |
Provides no method to retrieve the index of the element | Provides methods to retrieve the index of the elements |
iterator() method is available for the entire Collection Framework | listIterator() is only available for the collections implementing the List interface |
46. Differentiate between HashSet and TreeSet.
HashSet | TreeSet |
Uses HasMap to store elements | Uses Treemap to store elements |
It is unordered in nature | By default, it stores elements in their natural ordering |
Has faster processing time | Has slower processing time |
Uses hasCode() and equals() for comparing | Uses compare() and compareTo() for comparing |
Allows only one null element | Doesn’t allow any null element |
Takes up less memory space | Takes up more memory space |
47. Differentiate between Queue and Deque.
Queue | Deque |
Refers to single-ended queue | Refers to double-ended queue |
Elements can be added or removed from only one end | Elements can be added and removed from either end |
Less versatile | More versatile |
48. Differentiate between HashMap and TreeMap.
HashMap | TreeMap |
Doesn’t preserves any ordering | Preserves the natural ordering |
Implicitly implements the hashing principle | Implicitly implements the Red-Black Tree Implementation |
Can store only one null key | Cannot store any null key |
More memory usage | Less memory usage |
Not synchronized | Not synchronized |
49. Differentiate between ArrayList and Vector.
ArrayList | Vector |
Non-synchronized in nature | Synchronized in nature |
It is not a legacy class | Is a legacy class |
Increases size by 1/2 of the ArrayList | Increases size by double of the ArrayList |
It is not thread-safe | It is thread-safe |
50. Differentiate between failfast and failsafe.
failfast | failsafe |
Doesn’t allow modifications of a collection while iterating | Allows modifications of a collection while iterating |
Throws ConcurrentModificationException | Don’t throw any exceptions |
Uses the original collection to traverse over the elements | Uses a copy of the original collection to traverse over the elements |
Don’t require extra memory | Require extra memory |
So this brings us to the end of the Java Collections interview questions. The topics that you learned in this Java Collections Interview Questions are the most sought-after skill sets that recruiters look for in a Java Professional. These sets of Java Collection Interview Questions will definitely help you ace your job interview.
Good luck with your interview!