Git bisect
Git bisect is a command-line tool that helps identify the commit that introduced a bug in your code. It is particularly useful when the bug is not easily reproducible, and you have a large number of commits to go through.
The idea behind git bisect is simple: it performs a binary search through your commit history, starting from a known good commit and a known bad commit, and it helps you identify the commit that introduced the bug.
Here is a step-by-step guide on how to use git bisect:
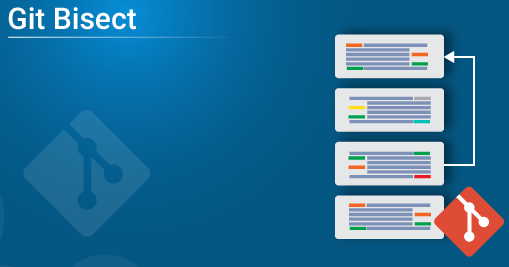
Why use git bisect?
Helps you identify the commit that introduced a bug in your code.
Saves time and effort by automating the process of searching through your commit history.
Improves the accuracy of identifying the bad commit by using a binary search algorithm.
Helps you find the root cause of the bug and fix it more efficiently.
How does ‘git bisect’ search?
Git bisect starts by dividing your commit history into two halves and checking out the commit in the middle. You then test your code to see if the bug is present. Based on the result, git bisect eliminates one half of the commit history and repeats the process with the other half until it identifies the bad commit.
Syntax
The syntax of the git bisect command is as follows:
ruby $ git bisect start $ git bisect bad <bad_commit> $ git bisect good <good_commit>
where <bad_commit>
is the commit where the bug was first detected, and <good_commit>
is a commit where you know the code was working correctly.
Initial Project Setup
First, you need to set up your project for git bisect. Make sure you have a clean working directory, and all changes are committed.
ruby $ git checkout master $ git pull origin master
Test the application
Next, you need to test your application to see if the bug is present. You can use any method that you like, such as running tests or manually testing the code. Once you have determined whether the bug is present or not, you can tell git bisect whether the current commit is good or bad.
ruby $ git bisect start $ git bisect bad HEAD$ git bisect good <good_commit>
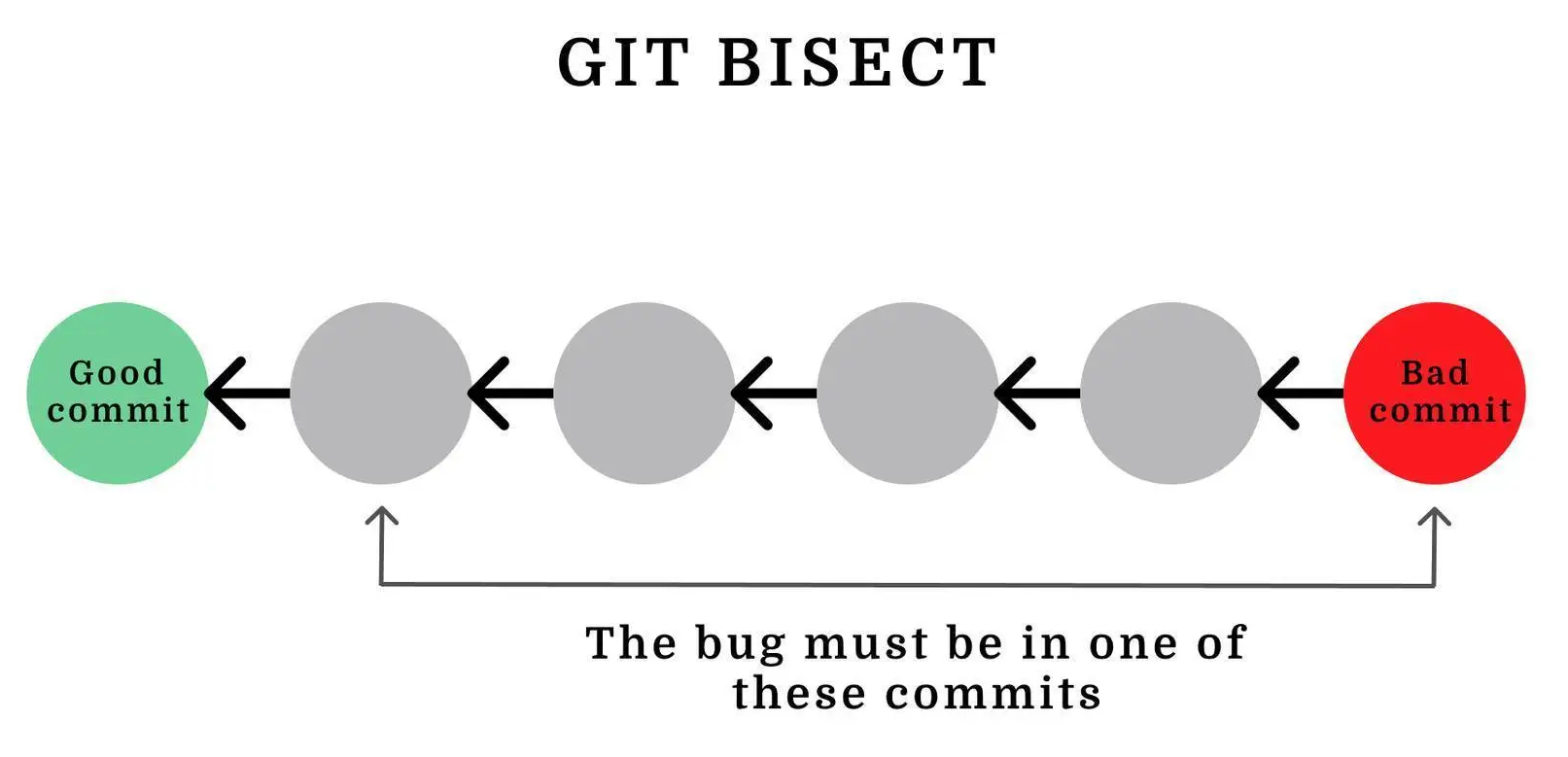
Identification of bad commit
After the initial setup, git bisect will start searching for the bad commit. It will divide your commit history into two halves and check out the commit in the middle. You will then need to test your code to see if the bug is present.
If the bug is present, you will run the following command:
ruby $ git bisect bad
If the bug is not present, you will run the following command:
ruby $ git bisect good
Git bisect will then repeat the process by dividing the remaining commit history into two halves and checking out the commit in the middle.
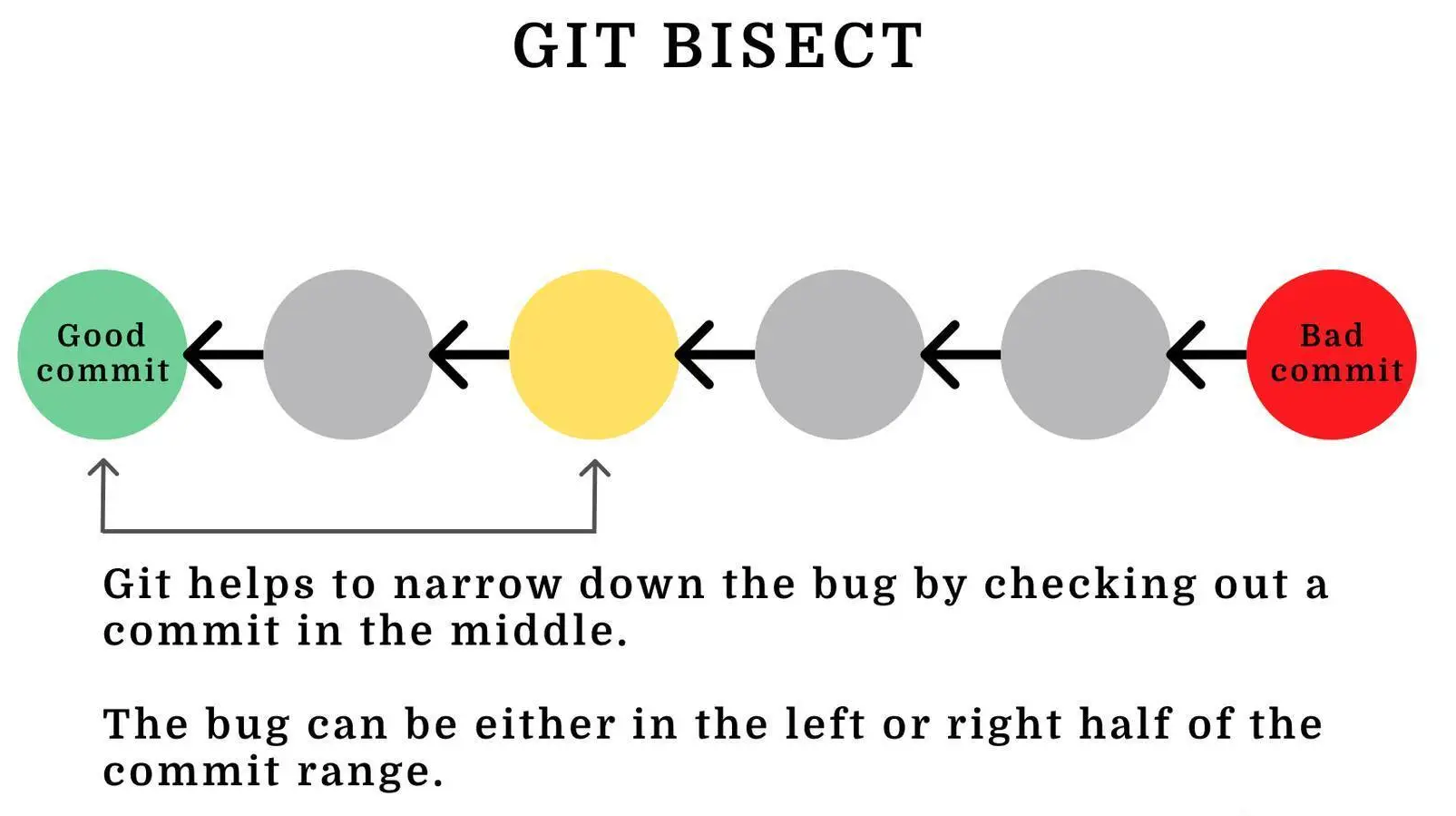
Found the bad commit
Once git bisect identifies the bad commit, it will print out the commit hash and message. You can then use git diff to see what changes were made in that commit.
sql $ git bisect bad $ git bisect good <good_commit> Bisecting: 6 revisions left to test after this (roughly 2 steps) [commit hash] [commit message]
Understand what file had the bug
You can use git show to see the changes that were made in the bad commit.
shell $ git show [commit hash]
Once you have identified the changes that introduced the bug, you can start debugging and fixing the code.
Stop the bisection
Once you have identified the bad commit and fixed the bug, you can stop git bisect by running the following command:
perl $ git bisect reset
This will reset your working directory to the state it was in before you started git bisect.
How to fix/debug the code?
Once you have identified the bad commit, you can use git show to see the changes that were made in that commit. You can then use any debugging tool that you like to find and fix the bug.
Here are some general tips for debugging:
- Start by reproducing the bug in a controlled environment, such as a unit test or a small test case.
- Use a debugger to step through the code and identify the root cause of the bug.
- Add debug output to your code to help you understand what’s going on.
- Check the documentation and the codebase to see if there are any known issues that could be causing the bug.
- Use code reviews and pair programming to get feedback from other developers.
Once you have identified the root cause of the bug, you can fix it and create a new commit. Make sure to write a good commit message that describes the changes you made and why you made them.
Here’s an example of how to fix a bug using git bisect:
ruby $ git bisect start $ git bisect bad HEAD$ git bisect good <good_commit> # git bisect identifies the bad commit$ git bisect bad $ git show [commit hash] # identify the root cause of the bug and fix it$ vim [filename] $ git add [filename] $ git commit -m "fix bug in [filename]"# reset the bisect and verify that the bug is fixed$ git bisect reset $ git checkout <good_commit> $ [test your application to verify the bug is fixed
In summary, git bisect is a powerful tool that helps you identify the commit that introduced a bug in your code. By automating the process of searching through your commit history, git bisect saves you time and effort and helps you fix bugs more efficiently.
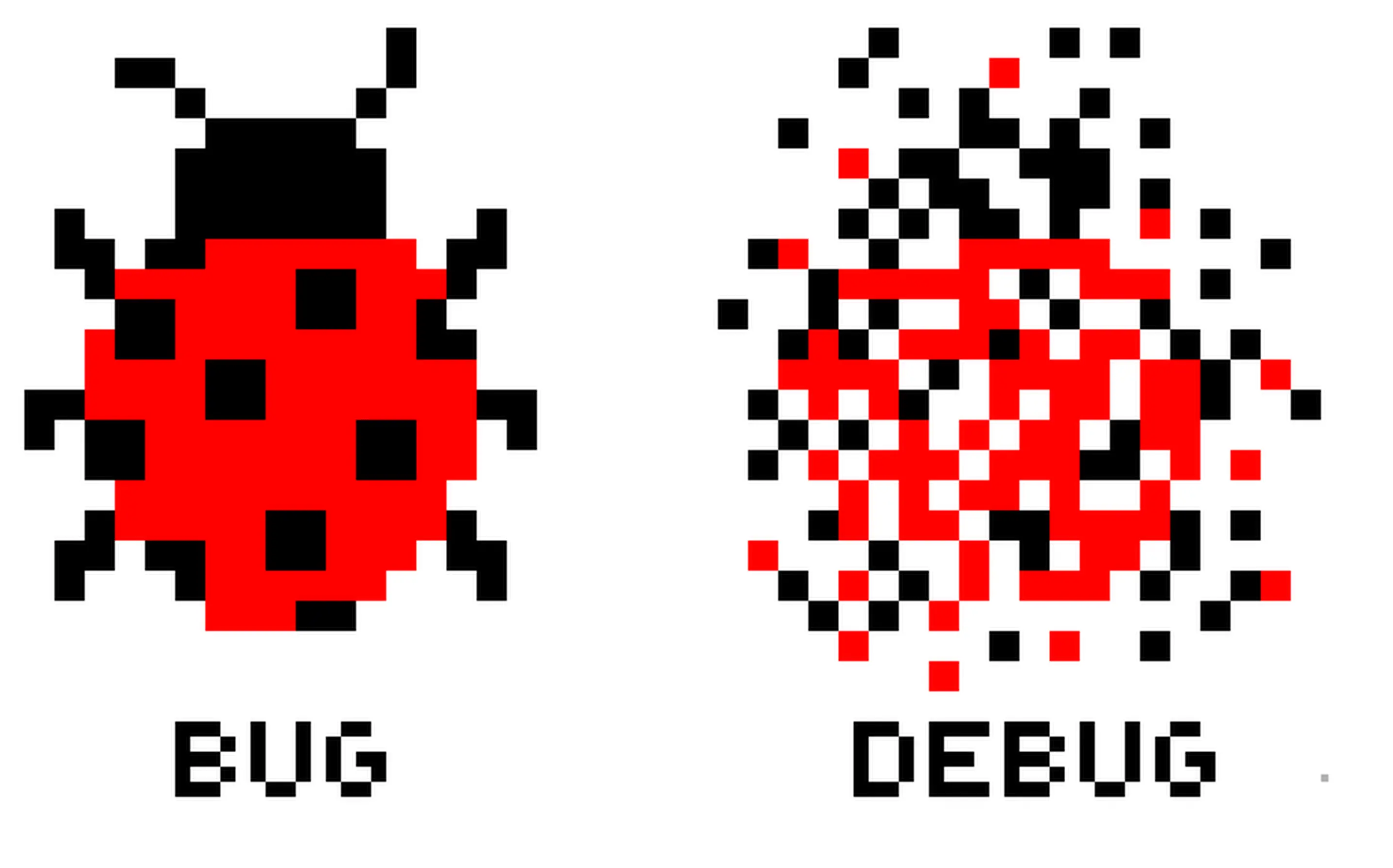
It is important to note that git bisect can be used not only for identifying bugs but also for identifying the commit that introduced a specific feature or caused a performance regression.
Here are some additional tips for using git bisect:
Make sure to use a binary search strategy to minimize the number of commits that need to be tested.
Use a consistent testing method to ensure that each commit is tested in the same way.
Use descriptive commit messages to make it easier to understand the changes that were made in each commit.
If you are working in a team, make sure to communicate your use of git bisect and coordinate with your team members to avoid conflicts.
In conclusion, git bisect is a powerful tool that can save you a lot of time and effort when it comes to identifying the commit that introduced a bug in your code. By automating the process of searching through your commit history, git bisect makes it easier to fix bugs and improve the quality of your codebase.
How git bisect Works Using git bisect to Quickly Find Bugs?
Git bisect is a tool provided by Git that allows developers to quickly identify the commit that introduced a bug in their code. The tool works by performing a binary search through the commit history of a repository, testing each commit until the commit that introduced the bug is found.
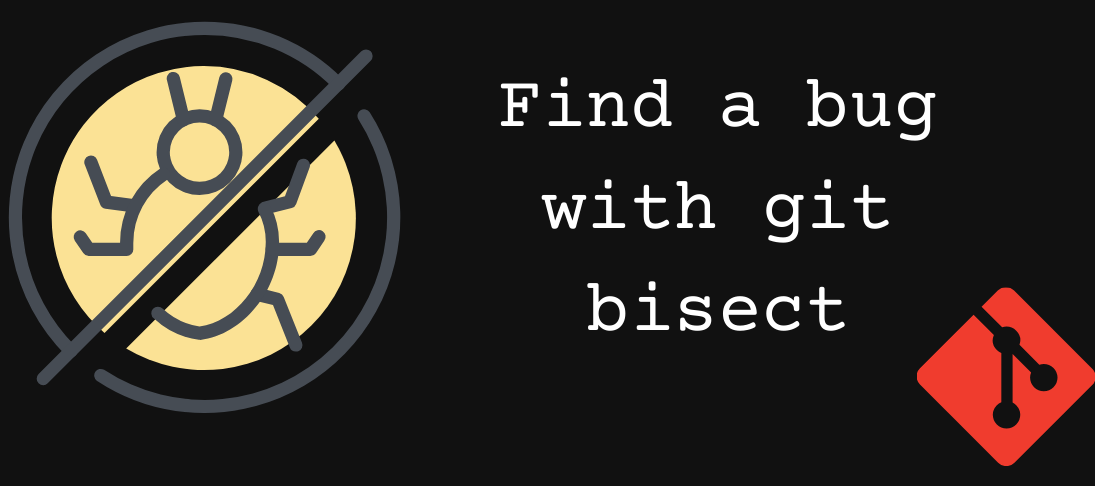
Here’s how git bisect works:
First, you need to start the bisect process by running the command:
sql git bisect start
Next, you need to specify a “good” commit and a “bad” commit. The “good” commit is a commit that you know does not have the bug, while the “bad” commit is a commit that you know has the bug. For example, you might use the following commands to mark the current commit as “bad” and an earlier commit as “good”:
php git bisect bad HEAD git bisect good <good_commit>
Git bisect will now select a commit between the “good” and “bad” commits and ask you to test it. You should test the commit and then tell git bisect whether the commit is “good” or “bad”. For example, you might use the following commands to test the commit and tell git bisect that it is “bad”:
git bisect bad
- Git bisect will then select another commit between the “good” and “bad” commits and ask you to test it. This process will continue until git bisect has identified the commit that introduced the bug.
- Once git bisect has identified the bad commit, you can use git show to examine the changes that were made in that commit and debug the code to fix the bug.
Using git bisect to quickly find bugs can save you a lot of time and effort compared to manually testing each commit in your repository. By automating the process of searching through your commit history, git bisect allows you to quickly identify the commit that introduced the bug and focus your efforts on debugging the code.
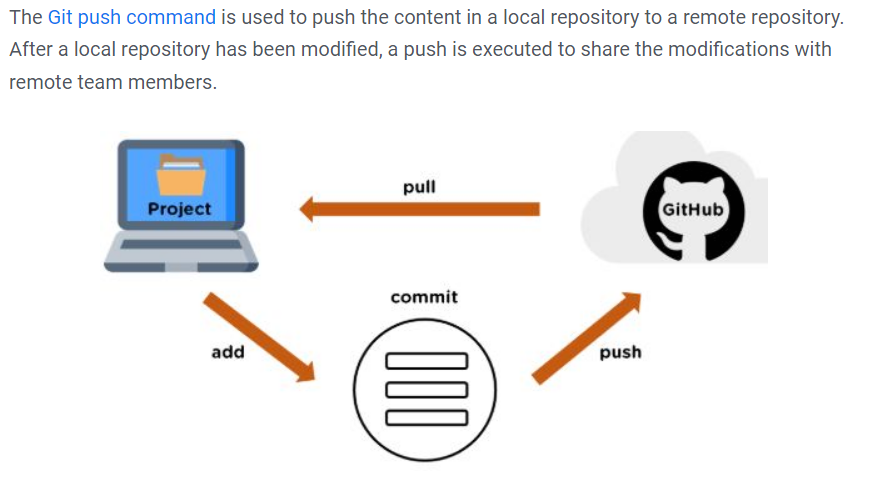
Here are some tips for using git bisect effectively to quickly find bugs:
1. Use a binary search strategy:
Git bisect uses a binary search algorithm to quickly narrow down the range of commits that need to be tested. By testing the “middle” commit between the “good” and “bad” commits, git bisect can quickly eliminate half of the remaining commits in the range. This means that the number of commits that need to be tested grows logarithmically with the size of the range, rather than linearly. Make sure to take advantage of this by using a consistent testing method and quickly determining whether a commit is “good” or “bad”.
2. Use automated tests:
Automated tests are an essential part of any modern development workflow. By using automated tests to test each commit in your repository, you can quickly identify the commit that introduced a bug without having to manually test each commit. Make sure to run your automated tests on each commit during the git bisect process to quickly identify the bad commit.
3. Use descriptive commit messages:
Descriptive commit messages can make it easier to identify the commit that introduced a bug. Make sure to write detailed commit messages that describe the changes that were made in each commit and why they were made. This can help you identify the root cause of the bug and fix it more quickly.
4. Coordinate with your team:
If you are working in a team, make sure to coordinate with your team members to avoid conflicts during the git bisect process. Communicate your use of git bisect and make sure that everyone is on the same page. You may also want to use a separate branch or repository for the git bisect process to avoid conflicts with ongoing development.
5. Know when to stop:
Git bisect can be a powerful tool, but it is not always necessary to test every commit in your repository. If you have a large repository with many commits, it may be more efficient to identify the bad commit manually by examining the changes that were made in each commit. Similarly, if you are able to reproduce the bug in a controlled environment, you may not need to use git bisect at all. Make sure to use git bisect when it is the most efficient and effective tool for the job.
Using git bisect to quickly find bugs can save you a lot of time and effort compared to manually testing each commit in your repository. By using a binary search strategy, automated tests, descriptive commit messages, and coordination with your team, you can make the most of git bisect and improve the quality of your codebase.
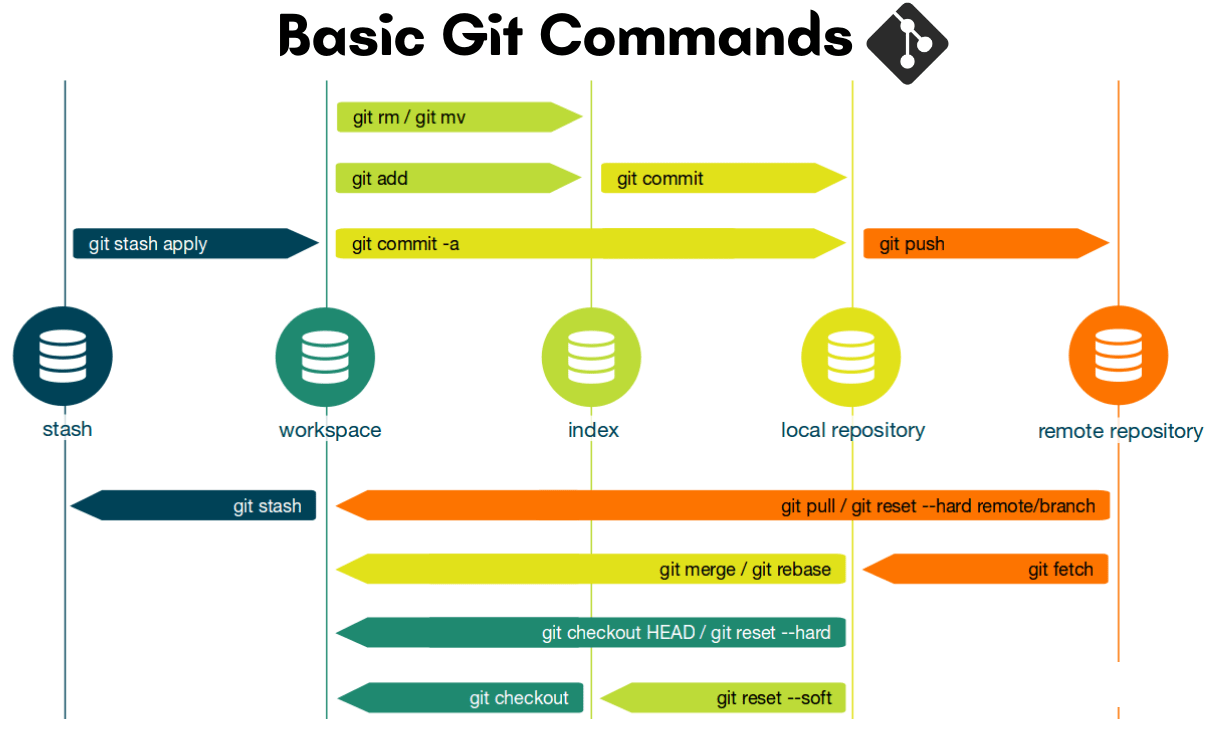
Git Bisect practical examples
Here are a few practical examples of how to use git bisect
:
Finding the Commit That Introduced a Bug:
Suppose you’re working on a project, and you’ve discovered a bug that wasn’t there before. You want to figure out which commit introduced the bug so that you can fix it. Here’s how you can use git bisect
to do that:
- Start the bisect process with
git bisect start
. - Identify a “good” commit (i.e., a commit where the bug doesn’t exist) with
git bisect good <commit>
. - Identify a “bad” commit (i.e., a commit where the bug exists) with
git bisect bad <commit>
. - Git will then checkout the middle commit between the good and bad commits for you to test. You can test the code and decide whether it’s good or bad.
- Depending on whether the code is good or bad, use
git bisect good
orgit bisect bad
to continue the process. - Git will continue bisecting until it has identified the commit that introduced the bug.
Finding the Commit That Fixed a Bug:
Suppose you’re working on a project, and you know that a bug existed at some point in the project’s history, but it’s been fixed. You want to figure out which commit fixed the bug so that you can understand how the bug was fixed. Here’s how you can use git bisect
to do that:
- Start the bisect process with
git bisect start
. - Identify a “bad” commit (i.e., a commit where the bug exists) with
git bisect bad <commit>
. - Identify a “good” commit (i.e., a commit where the bug doesn’t exist) with
git bisect good <commit>
. - Git will then checkout the middle commit between the good and bad commits for you to test. You can test the code and decide whether it’s good or bad.
- Depending on whether the code is good or bad, use
git bisect good
orgit bisect bad
to continue the process. - Git will continue bisecting until it has identified the commit that fixed the bug.
Identifying Performance Issues:
Suppose you’re working on a project, and you’ve noticed that the code is slower than it used to be. You want to figure out which commit introduced the performance issue so that you can optimize the code. Here’s how you can use git bisect
to do that:
- Start the bisect process with
git bisect start
. - Identify a “good” commit (i.e., a commit where the code was fast) with
git bisect good <commit>
. - Identify a “bad” commit (i.e., a commit where the code was slow) with
git bisect bad <commit>
. - Git will then checkout the middle commit between the good and bad commits for you to test. You can test the code and decide whether it’s fast or slow.
- Depending on whether the code is fast or slow, use
git bisect good
orgit bisect bad
to continue the process. - Git will continue bisecting until it has identified the commit that introduced the performance issue.
Finding the Source of a Regression:
Suppose you’re working on a project, and you’ve noticed that a particular feature used to work in an earlier version of the code, but it’s now broken. You want to figure out which commit introduced the regression so that you can fix it. Here’s how you can use git bisect
to do that:
- Start the bisect process with
git bisect start
. - Identify a “good” commit (i.e., a commit where the feature worked) with
git bisect good <commit>
. - Identify a “bad” commit (i.e., a commit where the feature is broken) with
git bisect bad <commit>
. - Git will then checkout the middle commit between the good and bad commits for you to test. You can test the code and decide whether the feature works or not.
- Depending on whether the feature works or not, use
git bisect good
orgit bisect bad
to continue the process. - Git will continue bisecting until it has identified the commit that introduced the regression.
Validating a New Feature:
Suppose you’re working on a project, and you’ve just added a new feature. You want to make sure that the feature works as intended and doesn’t break any existing functionality. Here’s how you can use git bisect
to do that:
- Start the bisect process with
git bisect start
. - Identify a “good” commit (i.e., a commit where the code worked before the new feature was added) with
git bisect good <commit>
. - Identify a “bad” commit (i.e., a commit with the new feature added) with
git bisect bad <commit>
. - Git will then checkout the middle commit between the good and bad commits for you to test. You can test the code and decide whether the feature works or not.
- Depending on whether the feature works or not, use
git bisect good
orgit bisect bad
to continue the process. - Git will continue bisecting until it has identified any commits that introduced issues with the new feature.
These are just a few practical examples of how git bisect
can be used to identify issues in a codebase. By using this tool, you can save a lot of time and effort when trying to track down bugs or performance issues.
Conclusion:
In conclusion, git bisect is a powerful tool for identifying bugs in your code by performing a binary search through the commit history of a repository. By specifying a “good” commit and a “bad” commit, and testing each commit in between, git bisect can quickly identify the commit that introduced the bug, allowing you to focus your debugging efforts on that commit.
To use git bisect effectively, it is important to use a consistent testing method, automate your tests, use descriptive commit messages, coordinate with your team, and know when to stop. By following these tips, you can make the most of git bisect and improve the quality of your codebase.
Overall, git bisect is a valuable tool for any developer looking to improve the quality of their code and streamline their debugging process. Whether you are working on a small personal project or a large team-based codebase, git bisect can help you quickly identify and fix bugs, saving you time and effort in the long run.