Arrays Python
What is an Array in Python and its Uses?
In Python, an array is a collection of items that are stored in a contiguous block of memory. The items in an array can be of any type, such as integers, strings, or other objects.
Arrays are useful for many purposes, such as:
- Storing a large number of data items of the same type
- Performing mathematical operations on the data (e.g. finding the average or standard deviation)
- Iterating over the data using a loop
- Implementing data structures such as stacks, queues, and heaps
Python provides the array module, which provides a convenient way to create and manipulate arrays of numerical data. However, since Python is a dynamically typed language, it doesn’t have a built-in array data structure like other languages. Alternative libraries such as numpy or pandas can be used for array manipulation and mathematics operations.
In Python, an array is a data structure that stores a collection of items. The items in an array can be of any data type, such as numbers, strings, or even other arrays.
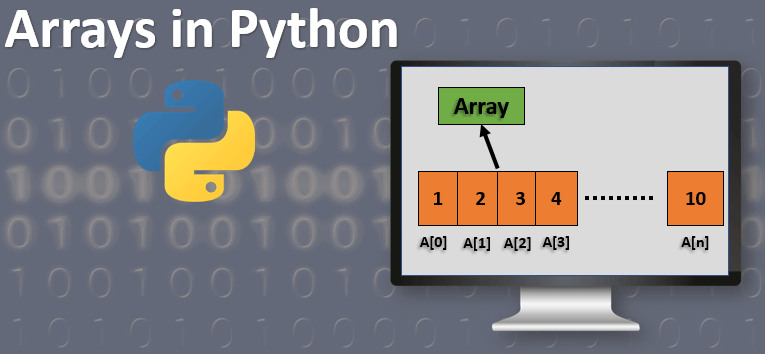
The standard library of Python does not have an array data type. Instead, Python has a list data type, which is very similar to an array and can be used in a similar way.
To create an array in Python, you can use the list() function, like this:
# Creating an empty array my_array = list() # Creating an array with items my_array = [1, 2, 3, 4, 5]
You can also use square brackets to create an array, like this:
my_array = [] my_array = [1, 2, 3, 4, 5]
You can access the items in an array by their index, which is the position of the item in the array. The first item in the array has an index of 0, the second item has an index of 1, and so on.
# Accessing items in the array
print(my_array[0]) # prints 1
print(my_array[1
]) # prints 2
You can also use negative indexing to access items in the array, where -1 represents the last item, -2 represents the second last item, and so on.
# Accessing items in the array using negative indexing print(my_array[-1]) # prints 5 print(my_array[-2]) # prints 4
You can also use slicing to access a specific range of items in the array. The syntax is my_array[start:end]
. This returns a new array containing the items from index start to index end-1.
# Slicing the array print(my_array[1:3]) # prints [2,3]
You can also use the len() function to get the number of items in an array.
# Getting the length of the array print(len(my_array)) # prints 5
You can also add, remove, update items in an array.
# Adding items to the array my_array.append(6) # Removing items from the array my_array.pop(3) # Updating items in the array my_array[2] = 7
There are many other things you can do with arrays in Python, such as iterating over the items in the array, sorting the array, and so on. Please let me know if you want me to explain any specific operation on array or want to know more about it.
Creating an Array in Python:
There are several ways to create an array in Python.

Using the array module:
import array
# Create an array of integers
arr = array.array('i', [1, 2, 3, 4, 5])
print(arr) # output: array('i', [1, 2, 3, 4, 5])
# Create an array of floating-point numbers
arr = array.array('f', [1.1, 2.2, 3.3, 4.4, 5.5])
print(arr) # output: array('f', [1.1, 2.2, 3.3, 4.4, 5.5])
Using a list comprehension:
# Create an array of integers
arr = [x for x in range(1, 6)]
print(arr) # output: [1, 2, 3, 4, 5]
# Create an array of floating-point numbers
arr = [x/10 for x in range(1, 6)]
print(arr) # output: [0.1, 0.2, 0.3, 0.4, 0.5]
Using numpy:
import numpy as np
# Create an array of integers
arr = np.array([1, 2, 3, 4, 5])
print(arr) # output: [1 2 3 4 5]
# Create an array of floating-point numbers
arr = np.array([1.1, 2.2, 3.3, 4.4, 5.5], dtype=np.float32)
print(arr) # output: [1.1 2.2 3.3 4.4 5.5]
Using list :
arr = [1,2,3,4,5]
Using range :
arr = range(1,6)
Note that numpy arrays are more powerful than python’s built-in arrays, as they allow for more efficient numerical operations and provide a wide range of functions and methods to work with arrays.
What are basic Array Operations in Python?
In Python, the array module provides an array() object that can be used to store and manipulate arrays of numeric data. Some of the basic operations that can be performed on arrays include:
- Indexing: Accessing individual elements of the array using their indices.
- Slicing: Extracting a sub-array from an array by specifying a range of indices.
- Reshaping: Changing the shape of the array by specifying a new number of rows and columns.
- Transpose: Flipping the array along its diagonal, changing the rows and columns.
- Concatenation: Combining two or more arrays into a single array.
- Splitting: Breaking an array into smaller arrays.
- Addition, subtraction, multiplication, and division: Performing mathematical operations on arrays and scalars.
- Aggregate functions: Computing statistics such as sum, mean, and standard deviation of the elements in the array.
Python also has numpy library which offers more advanced operations on array like Broadcasting, masking, and advanced indexing.
Here are a few more operations you can perform on arrays in Python:
Iterating over the items in an array:
You can use a for loop to iterate over the items in an array, like this:
for item in my_array: print(item)
Sorting an array:
You can use the sort() method to sort the items in an array in ascending order, like this:
my_array.sort()
If you want to sort the array in descending order, you can use the sort(reverse=True)
method like this:
my_array.sort(reverse=True)
Reversing an array:
You can use the reverse() method to reverse the order of items in an array like this:
my_array.reverse()
Finding the minimum and maximum value in an array:
You can use the min() and max() functions to find the minimum and maximum values in an array, respectively, like this:
min_value = min(my_array) max_value = max(my_array)
Removing duplicates from an array:
You can use the set() function to remove duplicates from an array. The set() function creates a new set object and automatically removes duplicates.
my_array = [1, 2, 2, 3, 4, 4, 5] my_array = list(set(my_array))
Searching for an item in an array:
You can use the in
keyword to check if an item is present in an array, like this:
if 3 in my_array: print("Item found") else: print("Item not found")
These are just a few examples of the many things you can do with arrays in Python. You can use these examples as a starting point and explore more on your own. Please let me know if there is anything else I can help you with.
Is Python list same as an Array?
No, a Python list is not the same as an array. A Python list is a built-in data structure that can store a collection of items, whereas an array is a data structure available in some programming languages (such as C and Java) that stores a collection of items of the same type. While Python lists can store items of different types, arrays typically store items of the same type. Additionally, Python lists have more functionality and are more flexible than arrays in many other languages.
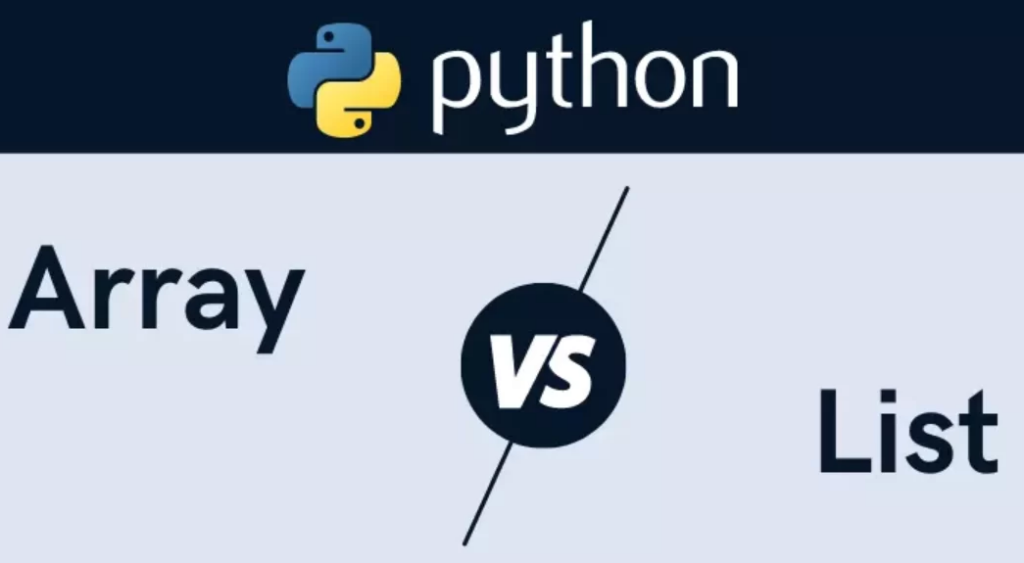
Here are a few more advanced operations you can perform on arrays in Python:
Using numpy library:
NumPy is a library for the Python programming language, adding support for large, multi-dimensional arrays and matrices, along with a large collection of high-level mathematical functions to operate on these arrays.
import numpy as np # Creating an array my_array = np.array([1, 2, 3, 4, 5]) # shape print(my_array.shape) # reshaping my_array = my_array.reshape(5,1) # Transpose print(my_array.T)
Stacking arrays:
You can use the numpy.concatenate()
function to stack multiple arrays along an axis.
import numpy as np # Creating arrays a = np.array([1, 2, 3]) b = np.array([4, 5, 6]) # Stacking arrays c = np.concatenate((a, b)) print(c)
Splitting an Array:
You can use the numpy.split()
function to split an array into multiple sub-arrays.
import numpy as np # Creating an array my_array = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10]) # Splitting the array sub_arrays = np.split(my_array, [2, 6]) # printing for array in sub_arrays: print(array)
Broadcasting:
Broadcasting is a powerful mechanism that allows numpy to work with arrays of different shapes when performing arithmetic operations.
import numpy as np # Creating arrays a = np.array([1, 2, 3]) b = 2 # Broadcasting c = a + b print(c)
Boolean indexing:
Boolean indexing is a powerful feature of numpy arrays that allows you to extract subsets of an array based on a Boolean mask.
import numpy as np # Creating an array my_array = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10]) # Creating the mask mask = my_array > 5 # Using the mask to extract the subset subset = my_array[mask] print(subset)
These are just a few examples of the many things you can do with arrays in Python using numpy library. It’s a powerful library with many other features which can be used for scientific computing and data analysis.