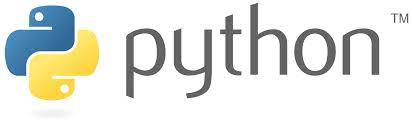
Python – Remove element from Lists
Python Lists have various in-built methods to remove items from the list. Apart from these, we can also use different methods to remove an element from the list by specifying a position using Python.
Delete an element from a list using the del
The Python del statement is not a function of List. Items of the list can be deleted using the del statement by specifying the index of the item (element) to be deleted.
- Python3
lst = ['Iris', 'Orchids', 'Rose', 'Lavender', 'Lily', 'Carnations'] print("Original List is :", lst) # using del statement # to delete item (Orchids at index 1) # from the list del lst[1] print("After deleting the item :", lst)
Output
Original List is : ['Iris', 'Orchids', 'Rose', 'Lavender', 'Lily', 'Carnations'] After deleting the item : ['Iris', 'Rose', 'Lavender', 'Lily', 'Carnations']
Remove an item from a list using list comprehension
In this method, we are using list comprehension. Here, we are appending all the elements except the elements that have to be removed.
- Python3
# Python program to remove given element from the list list1 = [1, 9, 8, 4, 9, 2, 9] # Printing initial list print ("original list : "+ str(list1)) # using List Comprehension # to remove list element 9 list1 = [ele for ele in list1 if ele != 9] # Printing list after removal print ("List after element removal is : " + str(list1))
Output
original list : [1, 9, 8, 4, 9, 2, 9] List after element removal is : [1, 8, 4, 2]
Remove an item from a list using remove()
We can remove an item from the list by passing the value of the item to be deleted as the parameter to remove() function.
- Python3
lst = ['Iris', 'Orchids', 'Rose', 'Lavender', 'Lily', 'Carnations'] print("Original List is :", lst) # using remove() # to delete item ('Orchids') # from the list lst.remove('Orchids') print("After deleting the item :", lst)
Output
Original List is : ['Iris', 'Orchids', 'Rose', 'Lavender', 'Lily', 'Carnations'] After deleting the item : ['Iris', 'Rose', 'Lavender', 'Lily', 'Carnations']
Delete an element from a List using pop()
The pop() is also a method of listing. We can remove the element at the specified index and get the value of that element using pop().
- Python3
lst = ['Iris', 'Orchids', 'Rose', 'Lavender', 'Lily', 'Carnations'] print("Original List is :", lst) # using pop() # to delete item ('Orchids' at index 1) # from the list a = lst.pop(1) print("Item popped :", a) print("After deleting the item :", lst)
Output
Original List is : ['Iris', 'Orchids', 'Rose', 'Lavender', 'Lily', 'Carnations'] Item popped : Orchids After deleting the item : ['Iris', 'Rose', 'Lavender', 'Lily', 'Carnations']
Remove an item from a list using discard() method
In this method, we convert a list into a set and then delete an item using the discard() function. Then we convert the set back to the list.
- Python3
# Python program to remove given element from the list lst = ['Iris', 'Orchids', 'Rose', 'Lavender', 'Lily', 'Carnations'] print("Original List is :", lst) # using discard() method to remove list element 'orchids' lst = set(lst) lst.discard('Orchids') # Converting set back to list lst=list(lst) print("List after element removal is :", lst)
Output
Original List is : ['Iris', 'Orchids', 'Rose', 'Lavender', 'Lily', 'Carnations'] List after element removal is : ['Lily', 'Carnations', 'Iris', 'Rose', 'Lavender']
Note: Since the list is converted to a set, all duplicates will be removed and the ordering of the list cannot be preserved.
Remove an item from a list using filter() method
In this method, we filter the unwanted element from the list using the filter() function.
- Python
# Python program to remove given element from the list lst = ['Iris', 'Orchids', 'Rose', 'Lavender', 'Lily', 'Carnations'] print("Original List is :", lst) # using discard() method to remove list element 'orchids' lst1 = filter(lambda item: item!='Orchids',lst) print("List after element removal is :", list(lst1))
Output
('Original List is :', ['Iris', 'Orchids', 'Rose', 'Lavender', 'Lily', 'Carnations']) ('List after element removal is :', ['Iris', 'Rose', 'Lavender', 'Lily', 'Carnations'])